<?php class GeoHelper { /** * @param int $lat1 * @param int $lon1 * @param int $lat2 * @param int $lon2 * @param string $unit * @return */ public static function distance($lat1, $lon1, $lat2, $lon2, $unit = "K") { $theta = $lon1 - $lon2; $dist = sin(deg2rad($lat1)) * sin(deg2rad($lat2)) + cos(deg2rad($lat1)) * cos(deg2rad ($lat2)) * cos(deg2rad($theta)); $dist = acos($dist); $dist = rad2deg($dist); $miles = $dist * 60 * 1.1515; $unit = strtoupper($unit); if ($unit == "K") { return ($miles * 1.609344); } else if ($unit == "N") { return ($miles * 0.8684); } else { //mi return $miles; } } /** * * @param string $address * @param string $apikey * @return array [1]:lat [0]:lng */ public static function getLatLng($address, $apikey) { $find = array("\n", "\r", " "); $replace = array("", "", "+"); $address = str_replace($find, $replace, $address); $url = 'http://maps.google.com/maps/geo?q=' . $address . '&key=' . $apikey . '&sensor=false&output=xml&oe=utf8'; $response = self::xml2array($url); $coordinates = $response['kml']['Response']['Placemark']['Point']['coordinates']; if (!empty($coordinates)) { $point_array = split(",", $coordinates); return $point_array; } } }
Calculate the distance between two points on the map, using Google Maps
All resources on this site are contributed by netizens or reprinted by major download sites. Please check the integrity of the software yourself! All resources on this site are for learning reference only. Please do not use them for commercial purposes. Otherwise, you will be responsible for all consequences! If there is any infringement, please contact us to delete it. Contact information: admin@php.cn
Related Article
25 Jul 2016
Calculate the distance between two points on the map
29 Jul 2016
:This article mainly introduces PHP to calculate the distance between two GPS points. Students who are interested in PHP tutorials can refer to it.
25 Jul 2016
PHP calculates the distance between two points on the map
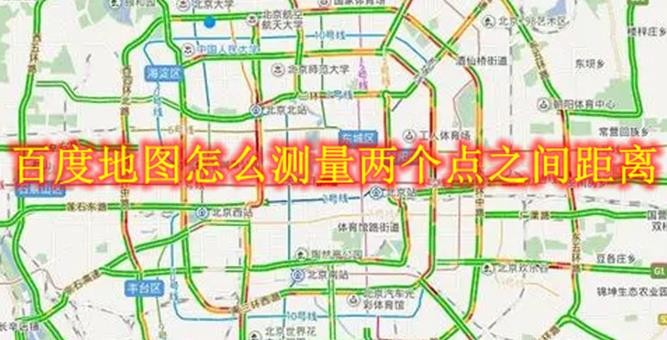
26 Jun 2024
How to measure the distance between two points on Baidu Maps? First, we open Baidu Maps, enter the homepage and click My in the lower right corner to enter the personal center. Then click on all functions next to the common function section on the personal center page. After entering the page, scroll down until you find the distance measurement function option, and then You can start to measure the distance between any two points. How to measure the distance between two points on Baidu Maps 1. Enter Baidu Maps, click My on the menu bar below, and click All Functions on the right side of the commonly used functions. 2. Scroll to the bottom and click on the distance measurement below. 3. Mark any location on the map to measure the distance.
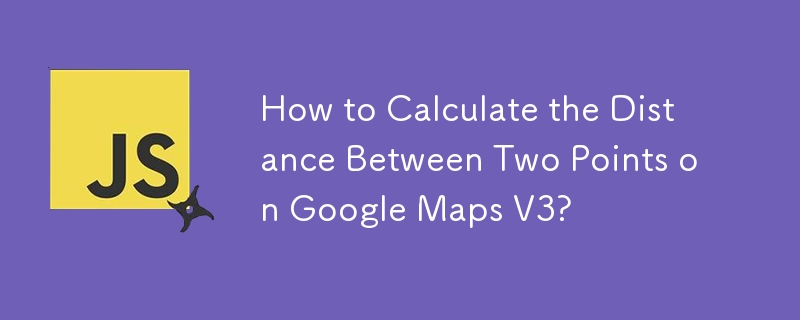
27 Nov 2024
Calculating Distance Between Points in Google Maps V3In Google Maps V3, the distance between two markers can be calculated using the Haversine...
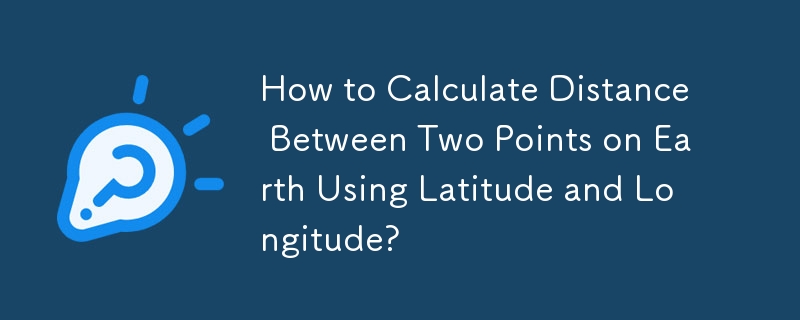
01 Nov 2024
Introducing Latitude and Longitude-Based Distance CalculationsCalculating the distance between two points on the Earth's surface using their...


Hot Tools

PHP library for dependency injection containers
PHP library for dependency injection containers
A collection of 50 excellent classic PHP algorithms
Classic PHP algorithm, learn excellent ideas and expand your thinking
Small PHP library for optimizing images
Small PHP library for optimizing images
