During front-end development, there are many places where the drag effect is used. Of course http://jqueryui.com/draggable/ is a good choice, but I am a person who wants to ask the question. I took some time to implement a similar plug-in using js, not much to say.
first: html and css
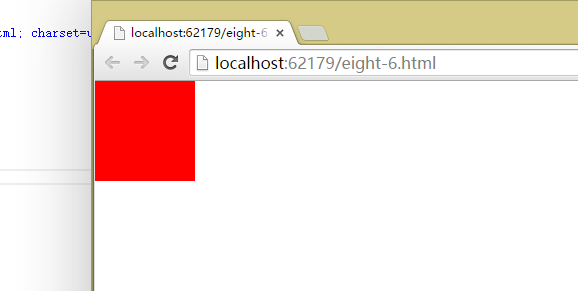
Now, let’s implement the main algorithm first:
<script><br>
window.onload = function () {<br>
//Get the div that needs to be dragged <br>
var div1 = document.getElementById("div1");<br>
//Add mouse press event<br>
div1.onmousedown = function (evt) {<br>
var oEvent = evt || event;<br>
// Get the distance from the mouse button to the div left top <br>
var distanceX = oEvent.clientX - div1.offsetLeft;<br>
var distanceY = oEvent.clientX - div1.offsetTop;<br>
//Add doc sliding time<br>
document.onmousemove = function (evt) {<br>
var oEvent = evt || event;<br>
//Recalculate the left top value of the div<br>
var left = oEvent.clientX - distanceX;<br>
var top = oEvent.clientY - distanceY;<br>
//left When less than or equal to zero, set to zero to prevent the div from being dragged out of the document <br>
If (left <= 0) {<br />
left = 0;<br />
}<br />
//When left exceeds the right boundary of the document, set it to the right boundary<br />
else if (left >= document.documentElement.clientWidth - div1.offsetWidth) {<br>
left = document.documentElement.clientWidth - div1.offsetWidth;<br>
}<br>
If (top <= 0) {<br />
top = 0;<br />
}<br />
else if (top >= document.documentElement.clientHeight - div1.offsetHeight) {<br>
Top = document.documentElement.clientHeight - div1.offsetHeight;<br>
}<br>
//Reassign the value to the div<br>
div1.style.top = top "px";<br>
div1.style.left = left "px";<br>
}<br>
//Add mouse lift event<br> div1.onmouseup = function () {<br>
document.onmousemove = null;<br>
div1.onmouseup = null;<br>
}<br>
}<br>
}<br>
</script>
yeah, use object-oriented implementation
js Draggle class:
Copy code The code is as follows:
function Drag(id) {
this.div = document.getElementById(id);
if (this.div) {
this.div.style.cursor = "move";
this.div.style.position = "absolute";
}
this.disX = 0;
this.disY = 0;
var _this = this;
this.div.onmousedown = function (evt) {
_this.getDistance(evt);
document.onmousemove = function (evt) {
_this.setPosition(evt);
}
_this.div.onmouseup = function () {
_this.clearEvent();
}
}
}
Drag.prototype.getDistance = function (evt) {
var oEvent = evt || event;
this.disX = oEvent.clientX - this.div.offsetLeft;
this.disY = oEvent.clientY - this.div.offsetTop;
}
Drag.prototype.setPosition = function (evt) {
var oEvent = evt || event;
var l = oEvent.clientX - this.disX;
var t = oEvent.clientY - this.disY;
if (l <= 0) {
l = 0;
}
else if (l >= document.documentElement.clientWidth - this.div.offsetWidth) {
l = document.documentElement.clientWidth - this.div.offsetWidth;
}
if (t <= 0) {
t = 0;
}
else if (t >= document.documentElement.clientHeight - this.div.offsetHeight) {
t = document.documentElement.clientHeight - this.div.offsetHeight;
}
this.div.style.left = l "px";
this.div.style.top = t "px";
}
Drag.prototype.clearEvent = function () {
this.div.onmouseup = null;
document.onmousemove = null;
}
at last: Final implementation:
window.onload = function () {
new Drag("div1");
new Drag("div2");
}
The effect is as follows:
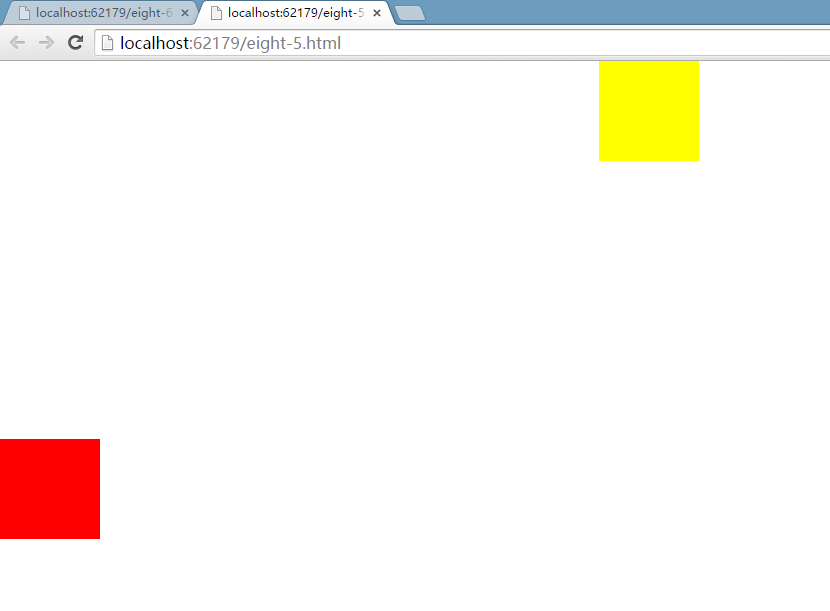
The above is the entire content of this article. I hope it will help everyone master javascript more proficiently.