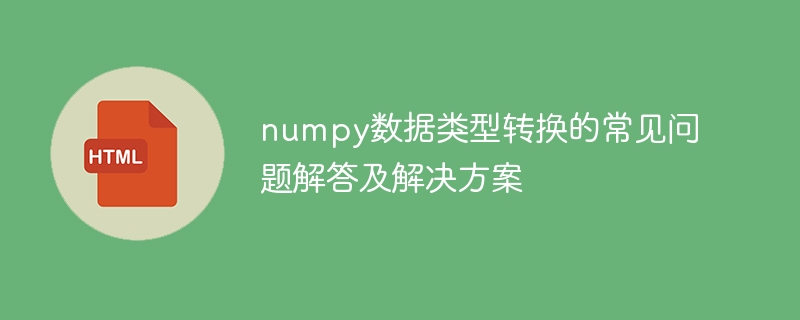
FAQs and solutions for numpy data type conversion
- Introduction
NumPy is a powerful Python library for scientific computing and data analysis. In NumPy, sometimes we need to convert between different data types, but we may encounter some common problems during the conversion process. This article will introduce some common data type conversion problems and give corresponding solutions and code examples.
- Question 1: How to convert the data type of an array from integer type to floating point type?
Solution: You can use the astype() function for type conversion.
Code example:
import numpy as np
Create an array of integer type
arr = np.array([1, 2, 3, 4, 5])
Convert the data type of the array to a floating point number type
arr_float = arr.astype(float)
print(arr_float)
Output result :[1. 2. 3. 4. 5.]
- Question 2: How to convert the data type of an array from floating point number type to integer type?
Solution: You can use the astype() function to convert an array of floating point type to an integer type, but you need to be aware that the precision of the decimal part may be lost.
Code example:
import numpy as np
Create an array of floating point number type
arr = np.array([1.1, 2.2, 3.3 , 4.4, 5.5])
Convert the data type of the array to an integer type
arr_int = arr.astype(int)
print(arr_int)
Output result :[1 2 3 4 5]
- Question 3: How to convert the data type of an array from Boolean to integer?
Solution: You can use the astype() function to convert an array of Boolean type to an integer type. In NumPy, True is represented as 1 and False is represented as 0.
Code example:
import numpy as np
Create an array of Boolean type
arr = np.array([True, False, True, False])
Convert the data type of the array to an integer type
arr_int = arr.astype(int)
print(arr_int)
Output result: [1 0 1 0]
- Question 4: How to convert the data type of an array from string type to integer type?
Solution: You can use the astype() function to convert an array of string type to an integer type. Note, however, that strings must be correctly converted to integers.
Code example:
import numpy as np
Create an array of string type
arr = np.array(['1', ' 2', '3', '4'])
Convert the data type of the array to an integer type
arr_int = arr.astype(int)
print(arr_int )
Output result: [1 2 3 4]
- Question 5: How to convert the data type of an array from integer type to string type?
Solution: You can use the astype() function to convert an array of integer type to a string type.
Code example:
import numpy as np
Create an array of integer type
arr = np.array([1, 2, 3, 4])
Convert the data type of the array to the string type
arr_str = arr.astype(str)
print(arr_str)
Output result: [ '1' '2' '3' '4']
- Conclusion
In NumPy, by using the astype() function, we can easily convert between different data types . However, when performing type conversion, special attention needs to be paid to the accuracy of the data and whether the string can be correctly converted to the target type. Common data type conversion problems can be easily solved using the astype() function to meet different scientific computing and data analysis needs.
The above is an introduction to frequently asked questions and solutions about numpy data type conversion. I hope it will be helpful to readers. If you have any other questions, please leave a message in the comment area.
The above is the detailed content of Solutions and answers to common numpy data type conversion problems. For more information, please follow other related articles on the PHP Chinese website!