


Interpretation and practice of practical methods of Java technology to improve database search efficiency
Database search is a very common task in modern software development. For databases with large amounts of data, improving search efficiency is a key requirement. In Java development, there are many technologies that can help us improve the efficiency of database search. This article will interpret and practice some practical methods and provide specific code examples.
- Index optimization
Index is one of the important means to improve search performance in the database. When designing database tables, you can create indexes for fields that are frequently searched. In Java, you can use JDBC to connect to the database and execute a Create Index statement to create an index. Here is a sample code:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement; public class IndexDemo { public static void main(String[] args) { try(Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); Statement stmt = conn.createStatement()) { String createIndexSQL = "CREATE INDEX index_name ON table_name(column_name);"; stmt.executeUpdate(createIndexSQL); System.out.println("Index created successfully."); } catch (SQLException e) { e.printStackTrace(); } } }
- Parameterized query
Parameterized query is a way to improve search efficiency, avoid SQL injection attacks, and allow query reuse plan. In Java, you can use PreparedStatement to implement parameterized queries. The following is a sample code:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class ParameterizedQueryDemo { public static void main(String[] args) { try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); PreparedStatement stmt = conn.prepareStatement("SELECT * FROM table_name WHERE column_name = ?")) { stmt.setString(1, "search_value"); try (ResultSet rs = stmt.executeQuery()) { while (rs.next()) { // 处理查询结果 } } } catch (SQLException e) { e.printStackTrace(); } } }
- Paging query
For databases with large amounts of data, returning all query results at once may cause memory overflow. In order to improve search efficiency, you can use paging query to return only a part of the results. In Java, you can use the LIMIT keyword to implement paging queries. The following is a sample code:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class PaginationDemo { public static void main(String[] args) { int pageIndex = 1; int pageSize = 10; try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); PreparedStatement stmt = conn.prepareStatement("SELECT * FROM table_name LIMIT ? OFFSET ?")) { stmt.setInt(1, pageSize); stmt.setInt(2, (pageIndex - 1) * pageSize); try (ResultSet rs = stmt.executeQuery()) { while (rs.next()) { // 处理查询结果 } } } catch (SQLException e) { e.printStackTrace(); } } }
- Asynchronous query
For some long-time query tasks, you can use asynchronous query to avoid blocking the main thread. In Java, you can use CompletableFuture and ExecutorService to implement asynchronous queries. The following is a sample code:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.concurrent.CompletableFuture; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class AsyncQueryDemo { public static void main(String[] args) throws Exception { ExecutorService executor = Executors.newFixedThreadPool(10); CompletableFuture.supplyAsync(() -> { try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); PreparedStatement stmt = conn.prepareStatement("SELECT * FROM table_name")) { try (ResultSet rs = stmt.executeQuery()) { // 处理查询结果 return "Query executed successfully."; } } catch (SQLException e) { e.printStackTrace(); return "Query failed: " + e.getMessage(); } }, executor).thenAccept(System.out::println); executor.shutdown(); } }
To sum up, through index optimization, parameterized query, paging query and asynchronous query, the efficiency of database search can be improved. In Java development, we can use technologies such as JDBC to implement these methods and practice them through sample code. By rationally using these methods, the needs of large-volume database searches can be better met.
The above is the detailed content of Interpretation and practice of practical methods of Java technology to improve database search efficiency. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undress AI Tool
Undress images for free

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
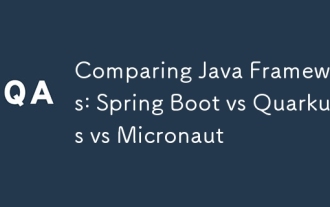
Pre-formanceTartuptimeMoryusage, Quarkusandmicronautleadduetocompile-Timeprocessingandgraalvsupport, Withquarkusoftenperforminglightbetterine ServerLess scenarios.2.Thyvelopecosyste,
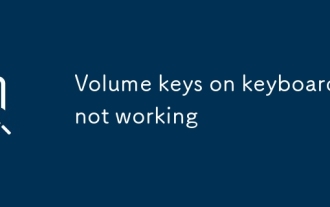
First,checkiftheFnkeysettingisinterferingbytryingboththevolumekeyaloneandFn volumekey,thentoggleFnLockwithFn Escifavailable.2.EnterBIOS/UEFIduringbootandenablefunctionkeysordisableHotkeyModetoensurevolumekeysarerecognized.3.Updateorreinstallaudiodriv
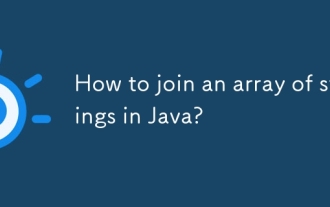
Using String.join() (Java8) is the easiest recommended method for connecting string arrays, just specify the separator directly; 2. For old versions of Java or when more control is needed, you can use StringBuilder to manually traverse and splice; 3. StringJoiner is suitable for scenarios that require more flexible formats such as prefixes and suffixes; 4. Using Arrays.stream() combined with Collectors.joining() is suitable for filtering or converting the array before joining; To sum up, if Java8 and above is used, the String.join() method should be preferred in most cases, which is concise and easy to read, but for complex logic, it is recommended.
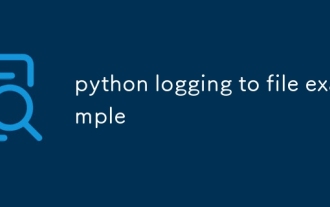
Python's logging module can write logs to files through FileHandler. First, call the basicConfig configuration file processor and format, such as setting the level to INFO, using FileHandler to write app.log; secondly, add StreamHandler to achieve output to the console at the same time; Advanced scenarios can use TimedRotatingFileHandler to divide logs by time, for example, setting when='midnight' to generate new files every day and keep 7 days of backup, and make sure that the log directory exists; it is recommended to use getLogger(__name__) to create named loggers, and produce
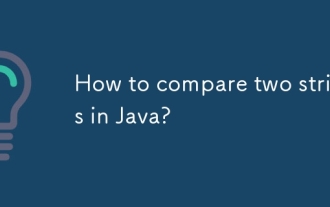
Use the .equals() method to compare string content, because == only compare object references rather than content; 1. Use .equals() to compare string values equally; 2. Use .equalsIgnoreCase() to compare case ignoring; 3. Use .compareTo() to compare strings in dictionary order, returning 0, negative or positive numbers; 4. Use .compareToIgnoreCase() to compare case ignoring; 5. Use Objects.equals() or safe call method to process null strings to avoid null pointer exceptions. In short, you should avoid using == for string content comparisons unless it is explicitly necessary to check whether the object is in phase.
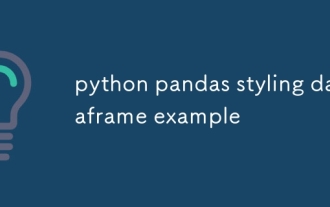
Using PandasStyling in JupyterNotebook can achieve the beautiful display of DataFrame. 1. Use highlight_max and highlight_min to highlight the maximum value (green) and minimum value (red) of each column; 2. Add gradient background color (such as Blues or Reds) to the numeric column through background_gradient to visually display the data size; 3. Custom function color_score combined with applymap to set text colors for different fractional intervals (≥90 green, 80~89 orange, 60~79 red,

Computed has a cache, and multiple accesses are not recalculated when the dependency remains unchanged, while methods are executed every time they are called; 2.computed is suitable for calculations based on responsive data. Methods are suitable for scenarios where parameters are required or frequent calls but the result does not depend on responsive data; 3.computed supports getters and setters, which can realize two-way synchronization of data, but methods are not supported; 4. Summary: Use computed first to improve performance, and use methods when passing parameters, performing operations or avoiding cache, following the principle of "if you can use computed, you don't use methods".
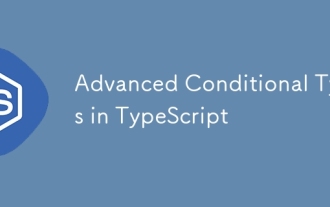
TypeScript's advanced condition types implement logical judgment between types through TextendsU?X:Y syntax. Its core capabilities are reflected in the distributed condition types, infer type inference and the construction of complex type tools. 1. The conditional type is distributed in the bare type parameters and can automatically split the joint type, such as ToArray to obtain string[]|number[]. 2. Use distribution to build filtering and extraction tools: Exclude excludes types through TextendsU?never:T, Extract extracts commonalities through TextendsU?T:Never, and NonNullable filters null/undefined. 3
