What is localstorage
A few days ago, I found that the operation of cookies was very strange in an old project. After consulting, I wanted to cache some information to avoid passing parameters on the URL, but I did not consider the problems that cookies would cause:
① The cookie size is limited to about 4k, which is not suitable for storing business data
② Cookies are sent together with HTTP transactions each time, which wastes bandwidth
We are doing mobile projects, so the technology that is really suitable to use here is localstorage. Localstorage can be said to be an optimization of cookies. It can be used to store data on the client conveniently and will not be transmitted with HTTP, but it is not No problem:
① The size of localstorage is limited to about 5 million characters, and different browsers are inconsistent
② Localstorage cannot be read in privacy mode
③ The essence of localstorage is to read and write files. If there is a lot of data, it will be stuck (firefox will Importing data into memory at one time is scary when you think about it)
④ localstorage cannot be crawled by crawlers, do not use it to completely replace URL parameter passing
Flaws do not hide the advantages, the above problems can be avoided, so our focus should be on how to use localstorage, and how to use it correctly.
Usage of localstorage
Basic knowledge
There are two types of localstorage storage objects:
① sessionStrage: session means session. The session here refers to the time period from entering the website to closing the website when the user browses a website. The validity period of the session object is only so long.
② localStorage: Save the data on the client hardware device, no matter what it is, which means that the data will still be there when the computer is turned on next time.
The difference between the two is that one is for temporary storage and the other is for long-term storage.
Here is a simple code to illustrate its basic use:
XML/HTML CodeCopy content to clipboard
- <div id="msg" style="margin: 10px 0; border: 1px solid black; padding: 10px; width: 300px;
- height: 100px;">
-
div>
-
<input type="text" id="text" />
-
<select id="type">
-
<option value="session">sessionStorageoption>
-
<option value="local">localStorageoption>
-
select>
-
<button onclick="save();">
-
保存数据button>
-
<button onclick="load();">
-
读取数据button>
-
<script type="text/javascript">
-
var msg = document.getElementById('msg'),
-
text = document.getElementById('text'),
-
type = document.getElementById('type');
-
-
function save() {
-
var str = text.value;
-
var t = type.value;
-
if (t == 'session') {
-
sessionStorage.setItem('msg', str);
-
} else {
-
localStorage.setItem('msg', str);
-
}
-
}
-
-
function load() {
-
var t = type.value;
-
if (t == 'session') {
-
msg.innerHTML = sessionStorage.getItem('msg');
-
} else {
-
msg.innerHTML = localStorage.getItem('msg');
-
}
-
}
-
script>
真实场景
实际工作中对localstorage的使用一般有以下需求:
① 缓存一般信息,如搜索页的出发城市,达到城市,非实时定位信息
② 缓存城市列表数据,这个数据往往比较大
③ 每条缓存信息需要可追踪,比如服务器通知城市数据更新,这个时候在最近一次访问的时候要自动设置过期
④ 每条信息具有过期日期状态,在过期外时间需要由服务器拉取数据
XML/HTML Code复制内容到剪贴板
-
define([], function () {
-
-
var Storage = _.inherit({
-
//Default attributes
-
properties: function () {
-
-
//Proxy object, default is localstorage
-
this.sProxy = window.localStorage;
-
-
//60 * 60 * 24 * 30 * 1000 ms ==30 days
-
this.defaultLifeTime = 2592000000;
-
-
//Local cache is used to store the mapping between all localstorage key values and expiration dates
-
this.keyCache = 'SYSTEM_KEY_TIMEOUT_MAP';
-
-
//When the cache capacity is full, the number of caches deleted each time
-
this.removeNum = 5;
-
-
},
-
-
assert: function () {
-
if (this.sProxy === null) {
-
throw 'not override sProxy property';
-
}
-
},
-
-
initialize: function (opts) {
-
this.propertys();
-
this.assert();
-
},
-
-
/*
-
Add localstorage
-
Data format includes unique key value, json string, expiration date, deposit date
-
sign is a formatted request parameter, used to return new data when the same request has different parameters. For example, if the list is the city of Beijing, and then switched to Shanghai, it will judge that the tag is different and update the cached data. The tag is equivalent to Signature
-
Only one piece of information will be cached for each key value
-
*/
-
set: function (key, value, timeout, sign) {
-
var _d = new Date();
-
//Deposit date
-
var indate = _d.getTime();
-
-
//Finally saved data
-
var entity = null;
-
-
if (!timeout) {
-
_d.setTime(_d.getTime() this.defaultLifeTime);
-
timeout = _d.getTime();
-
}
-
-
//
-
this.setKeyCache(key, timeout);
-
entity = this.buildStorageObj(value, indate, timeout, sign);
-
-
try {
-
this.sProxy.setItem(key, JSON.stringify(entity));
-
return true;
-
} catch (e) {
-
//When localstorage is full, clear it all
-
if (e.name == 'QuotaExceededError') {
-
// this.sProxy.clear();
-
//When localstorage is full, select the data closest to the expiration time to delete. This will also have some impact, but it feels better than clearing it all. If there are too many caches, this process will be more time-consuming, within 100ms
-
if (!this.removeLastCache()) throw 'The amount of data stored this time is too large';
-
this.set(key, value, timeout, sign);
-
console && console.log(e); -
}
-
return false;
-
},
-
-
//Delete expired cache
-
removeOverdueCache: function () {
-
var tmpObj = null, i, len;
-
-
var now = new Date().getTime();
-
//Get the key-value pair
-
var cacheStr = this.sProxy.getItem(this.keyCache);
-
var cacheMap = [];
-
var newMap = [];
-
if (!cacheStr) {
-
return;
-
}
-
-
cacheMap = JSON.parse(cacheStr);
-
-
for (i = 0, len = cacheMap.length; i < len; i ) {
-
tmpObj = cacheMap[i];
-
if (tmpObj.timeout < now) {
-
this.sProxy.removeItem(tmpObj.key);
-
} else {
-
newMap.push(tmpObj);
-
} -
this.sProxy.setItem(this.keyCache, JSON.stringify(newMap)); -
-
},
-
-
removeLastCache: function () {
-
var i, len;
-
var num = this.removeNum || 5;
-
-
//Get the key-value pair
-
var cacheStr = this.sProxy.getItem(this.keyCache);
-
var cacheMap = [];
-
var delMap = [];
-
-
//Indicates that the storage is too large
-
if (!cacheStr) return false;
-
-
cacheMap.sort(function (a, b) {
-
return a.timeout - b.timeout;
-
});
-
-
//What data was deleted
-
delMap = cacheMap.splice(0, num);
-
for (i = 0, len = delMap.length; i < len; i ) {
-
this.sProxy.removeItem(delMap[i].key);
-
}
-
-
this.sProxy.setItem(this.keyCache, JSON.stringify(cacheMap));
-
return true;
-
},
-
-
setKeyCache: function (key, timeout) {
-
if (!key || !timeout || timeout < new Date().getTime( )) return;
-
var i, len, tmpObj;
-
-
//Get the currently cached key value string
-
var oldstr = this.sProxy.getItem(this.keyCache);
-
var oldMap = [];
-
//Whether the current key already exists
-
var flag = false;
-
var obj = {};
-
obj.key = key;
-
obj.timeout = timeout;
-
-
if (oldstr) {
-
oldMap = JSON.parse(oldstr);
-
if (!_.isArray(oldMap)) oldMap = [];
-
}
-
-
for (i = 0, len = oldMap.length; i < len; i ) {
-
tmpObj = oldMap[i];
-
if (tmpObj.key == key) {
-
oldMap[i] = obj;
-
flag = true;
-
break;
-
}
-
}
-
if (!flag) oldMap.push(obj);
-
//最后将新数组放到缓存中
-
this.sProxy.setItem(this.keyCache, JSON.stringify(oldMap));
-
-
},
-
-
buildStorageObj: function (value, indate, timeout, sign) {
-
var obj = {
-
value: value,
-
timeout: timeout,
-
sign: sign,
-
indate: indate
-
};
-
return obj;
-
},
-
-
get: function (key, sign) {
-
var result, now = new Date().getTime();
-
try {
-
result = this.sProxy.getItem(key);
-
if (!result) return null;
-
result = JSON.parse(result);
-
-
//Data expiration
-
if (result.timeout < now) return null;
-
-
//Signature verification is required
-
if (sign) {
-
if (sign === result.sign)
-
return result.value;
-
return null;
-
} else {
-
return result.value;
-
-
} catch (e) { -
console && console.log(e); -
} -
return null; -
}, -
-
//Get signature -
getSign: function (key) { -
var result, -
sign = null;
try { -
-
result = this.sProxy.getItem(key);
if (result) { -
-
result = JSON.parse(result);
-
sign = result && result.sign
-
} catch (e) {
-
console && console.log(e);
-
}
-
return sign;
-
},
-
-
remove: function (key) {
-
return this.sProxy.removeItem(key);
-
},
-
-
clear: function () {
-
this.sProxy.clear();
-
}
-
});
-
-
Storage.getInstance = function () {
-
if (this.instance) {
-
return this.instance;
-
} else {
-
return this.instance = new this();
-
}
-
};
-
-
return Storage;
-
-
});
这段代码包含了localstorage的基本操作,并且对以上问题做了处理,而真实的使用还要再抽象:
XML/HTML Code复制内容到剪贴板
-
define(['AbstractStorage'], function (AbstractStorage) {
-
-
var Store = _.inherit({
-
//Default attributes
-
properties: function () {
-
-
//Each object must have a storage key and cannot be repeated
-
this.key = null;
-
-
//The default life cycle of a piece of data, S is seconds, M is minutes, D is days
-
this.lifeTime = '30M';
-
-
//Default return data
-
// this.defaultData = null;
-
-
//Proxy object, localstorage object
-
this.sProxy = new AbstractStorage();
-
-
},
-
-
setOption: function (options) {
-
_.extend(this, options);
-
},
-
-
assert: function () {
-
if (this.key === null) {
-
throw 'not override key property';
-
}
-
if (this.sProxy === null) {
-
throw 'not override sProxy property';
-
}
-
},
-
-
initialize: function (opts) {
-
this.propertys();
-
this.setOption(opts);
-
this.assert();
-
},
-
-
_getLifeTime: function () {
-
var timeout = 0;
-
var str = this.lifeTime;
-
var unit = str.charAt(str.length - 1);
-
var num = str.substring(0, str.length - 1);
-
var Map = {
-
D: 86400,
-
H: 3600,
-
M: 60,
-
S: 1
-
};
-
if (typeof unit == 'string') {
-
unitunit = unit.toUpperCase();
-
}
-
timeout = num;
-
if (unit) timeout = Map[unit];
-
-
//单位为毫秒
-
return num * timeout * 1000 ;
-
},
-
-
//缓存数据
-
set: function (value, sign) {
-
//获取过期时间
-
var timeout = new Date();
-
timeout.setTime(timeout.getTime() this._getLifeTime());
-
this.sProxy.set(this.key, value, timeout.getTime(), sign);
-
},
-
-
//设置单个属性
-
setAttr: function (name, value, sign) {
-
var key, obj;
-
if (_.isObject(name)) {
-
for (key in name) {
-
if (name.hasOwnProperty(key)) this.setAttr(k, name[k], value);
-
}
-
return;
-
}
-
-
if (!sign) sign = this.getSign();
-
-
//获取当前对象
-
obj = this.get(sign) || {};
-
if (!obj) return;
-
obj[name] = value;
-
this.set(obj, sign);
-
-
},
-
-
getSign: function () {
-
return this.sProxy.getSign(this.key);
-
},
-
-
remove: function () {
-
this.sProxy.remove(this.key);
-
},
-
-
removeAttr: function (attrName) {
-
var obj = this.get() || {};
-
if (obj[attrName]) {
-
delete obj[attrName];
-
}
-
this.set(obj);
-
},
-
-
get: function (sign) {
-
var result = [], isEmpty = true, a;
-
var obj = this.sProxy.get(this.key, sign);
-
var type = typeof obj;
-
var o = { 'string': true, 'number': true, 'boolean': true };
-
if (o[type]) return obj;
-
-
if (_.isArray(obj)) {
-
for (var i = 0, len = obj.length; i < len; i ) {
-
result[i] = obj[i];
-
}
-
} else if (_.isObject(obj)) {
-
result = obj;
-
}
-
-
for (a in result) {
-
isEmpty = false;
-
break;
-
}
-
return !isEmpty ? result : null;
-
},
-
-
getAttr: function (attrName, tag) {
-
var obj = this.get(tag);
-
var attrVal = null;
-
if (obj) {
-
attrVal = obj[attrName];
-
}
-
return attrVal;
-
}
-
-
});
-
-
Store.getInstance = function () {
-
if (this.instance) {
-
return this.instance;
-
} else {
-
return this.instance = new this();
-
}
-
};
-
-
return Store;
-
});
我们真实使用的时候是使用store这个类操作localstorage,代码结束简单测试:
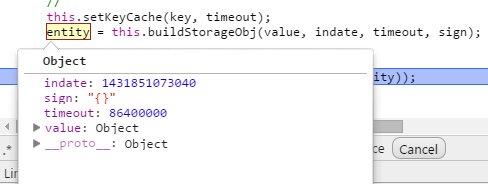
存储完成,以后都不会走请求,于是今天的代码基本结束 ,最后在android Hybrid中有一后退按钮,此按钮一旦按下会回到上一个页面,这个时候里面的localstorage可能会读取失效!一个简单不靠谱的解决方案是在webapp中加入:
XML/HTML Code复制内容到剪贴板
-
window.onunload = function () { };//适合单页应用,不要问我为什么,我也不知道
结语
localstorage是移动开发必不可少的技术点,需要深入了解,具体业务代码后续会放到git上,有兴趣的朋友可以去了解