Disadvantages of synchronous form validation
When responding to an error message, the entire page needs to be reloaded (although there is caching, the client still needs to compare whether each file has been updated through the http protocol to keep the file up to date)
After the server responds with an error, all the information previously entered by the user is lost, and the user needs to fill it in from scratch (some browsers cache this data for us)
The original intention of asynchronous verification form
Improve user experience
Minimize network requests and reduce server pressure
Let’s look at a common asynchronous form verification (verify whether the employee number exists in the background, and if it exists, it is a valid employee number)

Verify employee number
var BASE_PATH = '${rc.contextPath}';
var $workerIdInput = $('#workerIdInput');
var $workerIdError = $('#workerIdError');
//Identify whether the job number entered by the user is correct
var isWorkerIdCorrect = false;
var ERROR_WORKER_ID_IS_NULL = "Employee ID cannot be empty";
var ERROR_WORKER_ID_IS_NOT_CORRECT = "Please enter a valid employee ID";
//Display error message
function showWorkerIdError(errorMessage) {
$workerIdError.html(errorMessage);
$workerIdError.show();
}
//Hide error message
$workerIdInput.on('keydown', function() {
$workerIdError.hide();
});
//Save the last entered job number
$workerIdInput.on('focus', function() {
var workerId = $.trim($(this).val());
$(this).data('before', workerId);
});
//Verify when blurring
$workerIdInput.on('blur', function() {
var workerId = $.trim($(this).val());
//When the length is 0, the error message that the job number is empty is displayed
if (!workerId.length) {
ShowWorkerIdError(ERROR_WORKER_ID_IS_NULL);
Return false;
}
//If the data currently entered by the user is the same as the data entered last time, the background interface will not be called
//Suppose the user enters 123456, calls the background interface, and returns the result as incorrect job number
//After the user changes the input content and it is still 123456, the verification program will not access the network and directly display the error message
if (workerId == $(this).data('before')) {
If (!isWorkerIdCorrect) {
showWorkerIdError(ERROR_WORKER_ID_IS_NOT_CORRECT);
}
Return false;
}
//Call the backend interface to check whether the employee ID is correct
checkWorkerIdExists(workerId, function(data) {
isWorkerIdCorrect = data.isWorkerIdExists;
If (!isWorkerIdCorrect) {
showWorkerIdError(ERROR_WORKER_ID_IS_NOT_CORRECT);
}
});
});
function checkWorkerIdExists(workerId, callback) {
$.ajax({
URL: BASE_PATH '/forgotPwd/checkWorkerIdExists.htm',
Data: {
workerId: workerId
},
Success: callback
});
}
$workerIdForm.on('submit', function() {
//Only when the server returns true, our form can be submitted
if (!isWorkerIdCorrect) {
$workerIdInput.focus();
Return false;
}
});
After writing the above code, the verification of an input box is basically completed.
I think there are still areas that affect user experience
The carriage return operation is not supported yet, oh my god, even carriage return must be able to submit the form
If the user's Internet speed is slow, there will be no response when clicking the submit button, because isWorkerIdCorrect is false, and it will be true only if the server verification is successful
The following is the modified code:
var BASE_PATH = '${rc.contextPath}';
var $workerIdInput = $('#workerIdInput');
var $workerIdError = $('#workerIdError');
//Identify whether the job number entered by the user is correct
var isWorkerIdCorrect = false;
//Identify whether the background verification of employee ID has been completed (true: verification is in progress, false: verification has not started or has ended)
var isWorkerIdLoading = false;
//Identify whether the user has submitted the form
var isSubmit = false;
var ERROR_WORKER_ID_IS_NULL = "Employee ID cannot be empty";
var ERROR_WORKER_ID_IS_NOT_CORRECT = "Please enter a valid employee ID";
//Display error message
function showWorkerIdError(errorMessage) {
$workerIdError.html(errorMessage);
$workerIdError.show();
}
//Hide error message
$workerIdInput.on('keydown', function() {
$workerIdError.hide();
});
//Save the last entered job number
$workerIdInput.on('focus', function() {
var workerId = $.trim($(this).val());
$(this).data('before', workerId);
});
//Verify when blurring
$workerIdInput.on('blur', function() {
var workerId = $.trim($(this).val());
//When the length is 0, the error message that the job number is empty is displayed
if (!workerId.length) {
ShowWorkerIdError(ERROR_WORKER_ID_IS_NULL);
Return false;
}
//If the data currently entered by the user is the same as the data entered last time, the background interface will not be called
//Suppose the user enters 123456, calls the background interface, and returns the result as incorrect job number
//After the user changes the input content and it is still 123456, the verification program will not access the network and directly display the error message
if (workerId == $(this).data('before')) {
If (!isWorkerIdCorrect) {
showWorkerIdError(ERROR_WORKER_ID_IS_NOT_CORRECT);
}
Return false;
}
//Call the backend interface to check whether the employee ID exists
checkWorkerIdExists(workerId, function(data) {
isWorkerIdCorrect = data.isWorkerIdExists;
If (!isWorkerIdCorrect) {
showWorkerIdError(ERROR_WORKER_ID_IS_NOT_CORRECT);
}
});
});
function checkWorkerIdExists(workerId, callback) {
$.ajax({
URL: BASE_PATH '/forgotPwd/checkWorkerIdExists.htm',
Data: {
workerId: workerId
},
beforeSend: function() {
//Before sending the request, the ID is being verified
isWorkerIdLoading = true;
},
Success: callback,
Complete: function() {
//After finishing, close the logo
isWorkerIdLoading = false;
//During the background data verification process, if the user submits the form, it will be automatically submitted here
If (isSubmit) {
$workerIdForm.submit();
}
}
});
}
//Press enter to submit the form
$workerIdInput.on('keypress', function(e) {
if (e.which === 13) {
$(this).blur();
$workerIdForm.submit();
}
});
$workerIdForm.on('submit', function() {
//If the employee number is being verified in the background, it indicates that the user has submitted the form
if (isWorkerIdLoading) {
isSubmit = true;
Return false;
}
if (!isWorkerIdCorrect) {
$workerIdInput.focus();
Return false;
}
});
Final effect, the two input boxes in the picture are both asynchronously verified, but the effect looks the same as synchronous.
The picture uses gprs network to simulate the situation of slow network speed
Rendering
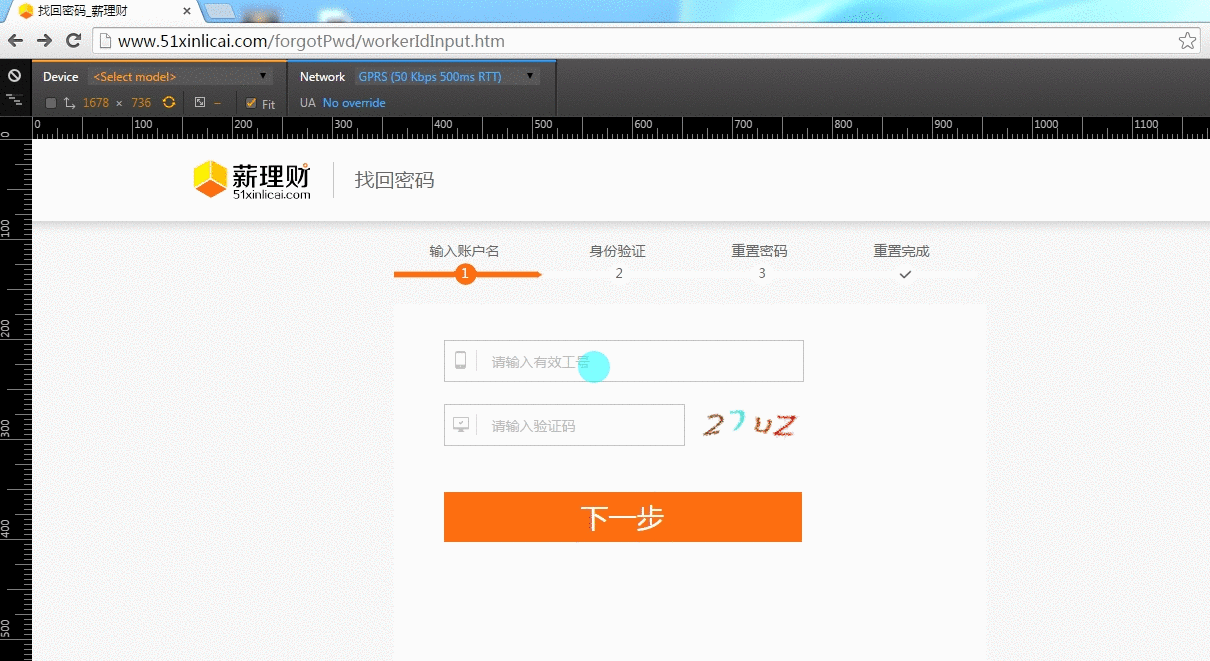