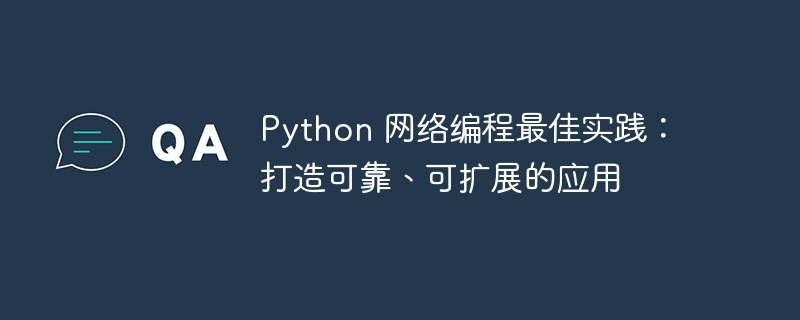
python is a popular high-level programming language that can be used for a variety of purposes, including network programming. Python NetworkProgramming can help you build various web applications such as WEB applications, api and Network Server.
To help you build reliable, scalable web applications, here are some best practices for Python web programming:
Using asynchronous programming:
Asynchronous programming allows your application to handle multiple requests simultaneously, improving performance and scalability. Python 3.5 and above has a built-in async
io module that can be used to easily write asynchronous code.
import asyncio
async def handle_client(reader, writer):
data = await reader.read(100)
writer.write(data)
await writer.drain()
async def main():
server = await asyncio.start_server(handle_client, "127.0.0.1", 8888)
async with server:
await server.serve_forever()
asyncio.run(main())
Copy after login
Using
Framework:
Python has many web programming frameworks to choose from, such as
Django, flask and Tornado. Using a framework can help you develop web applications quickly and save you a lot of time and effort.
Use
Cache:
Caching can help you improve the performance of your application, especially for frequently accessed data. Python has many caching libraries to choose from, such as
Redis and Memcached.
import redis
r = redis.Redis()
r.set("my-key", "my-value")
value = r.get("my-key")
Copy after login
Use
Load Balancing:
Load balancing can help you distribute requests across multiple servers, thereby improving the scalability of your application. Python has many load balancing libraries to choose from, such as HAProxy and
Nginx.
Use
MonitoringTools:
Monitoring tools can help you track the performance and health of your application. Python has many monitoring tools to choose from, such as
prometheus and Grafana.
Use
LogRecord:
Logging can help you track how your application is running and help you troubleshoot when problems arise. Python has many logging libraries to choose from, such as logging and
elk Stack.
Use
https:
HttpS can help you protect your applications from cyberattacks. Python has many libraries for easily implementing HTTPS, such as OpenSSL and pyOpenSSL.
Use CORS:
CORS helps you allow applications from different domains to access your application. Python has many libraries for easily implementing CORS, such as flask-cors and Djan
Go-cors-headers.
Test Application:
Testing your application can help you ensure that your application works as expected and can help you identify problems before deployment. Python has many testing frameworks to choose from, such as unittest and pytest.
Optimization Application:
Optimizing applications can help you improve the performance of your application. Python has many tools for optimizing applications, such as profile and cProfile.
Interaction with
database :
Python has many libraries for interacting with
databases, such as sqlAlchemy and Peewee.
import sqlalchemy
engine = sqlalchemy.create_engine("postgresql://user:passWord@host:port/database")
connection = engine.connect()
result = connection.execute("SELECT * FROM table")
for row in result:
print(row)
Copy after login
Interacting with web services:
Python has many libraries for interacting with web services, such as requests and urllib.
import requests
response = requests.get("https://example.com")
print(response.status_code)
print(response.text)
Copy after login
The above is the detailed content of Python Network Programming Best Practices: Building Reliable, Scalable Applications. For more information, please follow other related articles on the PHP Chinese website!