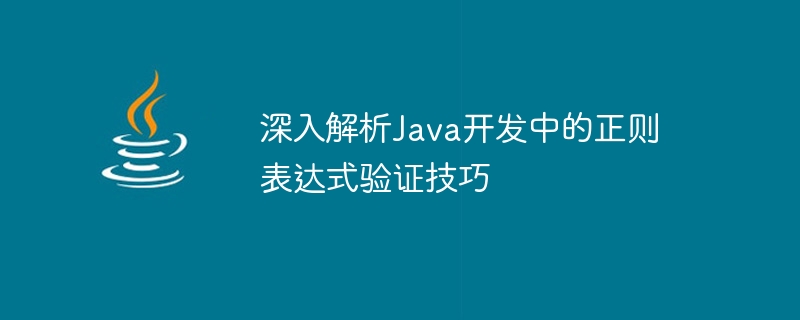
In-depth analysis of regular expression verification techniques in Java development
Regular expression is a general language for matching, finding and replacing character patterns. In Java development, regular expressions are often used to verify the legitimacy of input data, such as email addresses, mobile phone numbers, etc. Mastering regular expression validation skills is critical to developing efficient, accurate, and secure applications. This article will delve into the verification techniques of regular expressions in Java development.
- Using Pattern class and Matcher class: In Java, regular expressions can be used through Pattern class and Matcher class. The Pattern class represents the compiled regular expression pattern, and the Matcher class is used to match the input string. You can use the Pattern.compile method to compile a string into a Pattern object, and the Pattern.matcher method to match the Pattern object with the input string.
- Use of character classes: Character classes are used to specify one of a set of characters. For example, [abc] represents any character among a, b, and c. In email verification, you can use [a-zA-Z0-9] to indicate the allowed character range.
- Use of qualifiers: Qualifiers are used to specify the number of matching characters. For example, * means zero or more, means one or more,? Indicates zero or one. In mobile phone number verification, you can use d{11} to indicate that 11 digits must be matched.
- Use of escape characters: Some characters have special meanings, such as ., *, etc. If you need to match the characters themselves, rather than their special meanings, you can use escape characters. For example, when matching periods in email addresses, you can use .
- Boundary matching: Boundary matching is used to limit the matching position. ^ represents the starting position of the matched string, and $ represents the end position of the matched string. In email address verification, you can use ^[a-zA-Z0-9] @[a-zA-Z0-9] .[a-zA-Z0-9] $ to ensure the legitimacy of the email address.
- Grouping and backreference: Grouping is used to combine multiple characters into a whole for matching. You can use brackets () to group characters, and use backreferences, etc. to refer to previous groups. For example, when matching consecutive identical characters, you can use (.) to represent at least two consecutive occurrences of the same character.
- Non-greedy matching: By default, regular expressions are greedy and will match longer character sequences as much as possible. In some cases, you can use ? to achieve non-greedy matching, matching only the shortest character sequence. For example, use . ? to match the shortest non-empty sequence in text.
- Backreferences and zero-width assertions: Backreferences can refer to previous groups without being specified repeatedly in the pattern. Zero-width assertions are used to match a position, such as a word boundary. These advanced regular expression techniques can improve the flexibility and precision of regular expressions.
- Use of predefined character classes: Predefined character classes are shorthand for some commonly used character sets. For example, d represents a numeric character, w represents letters, numbers, and underscore characters, and s represents a whitespace character. You can use these predefined character classes to simplify writing regular expressions when validating input data.
- Performance Optimization of Java Regular Expressions: Regular expressions may face performance issues, especially when processing large amounts of data. To improve performance, you can use precompiled regular expression patterns to avoid recompiling the pattern on each match. In addition, greedy quantifiers and assertions can be used to reduce the number of backtracking, thereby increasing the matching speed.
Summary:
Regular expressions are a very powerful and practical tool in Java development and can be used to verify and process the legality of string data. The article introduces some techniques for regular expression verification in Java development, including character classes, qualifiers, escape characters, boundary matching, etc. Also, some more advanced techniques such as grouping and backreferences, non-greedy matching, backreferences, and zero-width assertions are mentioned. Finally, performance optimization methods for regular expressions are also mentioned. Mastering these skills can help developers better apply regular expressions and improve development efficiency and application quality.
The above is the detailed content of In-depth analysis of regular expression verification techniques in Java development. For more information, please follow other related articles on the PHP Chinese website!