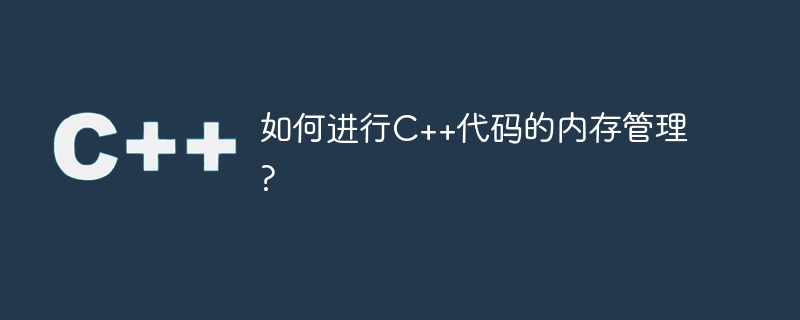
How to manage memory in C code?
C is a powerful programming language, but it also requires programmers to manage memory by themselves. Proper memory management is one of the keys to ensuring that programs run stably and efficiently. This article will introduce some common memory management techniques and best practices to help beginners and experienced developers better manage the memory of C code.
- Using stack and heap:
There are two main methods of memory allocation in C: stack and heap. The stack is a place where memory is automatically allocated and released, and is used to store local variables and function call information. The heap is where memory is dynamically allocated to store dynamically created objects and data structures. The memory on the stack is automatically released, while the memory on the heap needs to be released manually.
- Avoid memory leaks:
A memory leak refers to the failure to properly release memory that is no longer used, resulting in useless memory blocks in the system. To avoid memory leaks, you should always proactively release memory when it is no longer needed. Use the delete keyword to free the memory on the heap and set the pointer to nullptr to avoid dangling pointers.
- Use smart pointers:
C 11 introduces smart pointers, which are a method of automatically managing dynamic memory. Smart pointers use reference counting or other forms of garbage collection to track and manage pointers. Using smart pointers reduces the risk of memory leaks and simplifies code. Common smart pointers include std::shared_ptr and std::unique_ptr.
- Pay attention to the life cycle of the pointer:
The life cycle of the pointer refers to the life cycle of the object that the pointer effectively points to. Make sure that the pointer is only used when it is valid, and when the pointer is no longer valid, it is set to nullptr in time. Especially when passing pointers between functions, make sure that the pointer is always valid during transfer and use.
- Use the RAII principle:
RAII (resource acquisition is initialization) is a C programming paradigm that ensures the correct acquisition and release of resources by acquiring resources in the constructor and releasing them in the destructor . Using the RAII principle can effectively avoid resource leaks and incorrect releases.
- Avoid buffer overflow:
Buffer overflow is the phenomenon of writing data outside the allocated memory block. To avoid buffer overflows, you should incorporate bounds checking into your code and ensure that the data being written does not exceed the allocated memory space.
- Reasonable use of dynamic memory allocation:
Dynamic memory allocation is the process of allocating memory as needed when the program is running. When using dynamic memory allocation, pay attention to the balance between allocating and releasing memory. Avoid allocating too much or too little memory to avoid wasting resources or causing program crashes.
- Use container classes:
Container classes in C such as std::vector and std::list provide convenient dynamic memory management functions. Container classes are responsible for memory allocation and release, and provide a set of convenient methods to manipulate and access data. Using container classes simplifies code and reduces the complexity of memory management.
To sum up, correct memory management is crucial to the running and performance of C code. By following the above guidelines and best practices, developers can effectively manage the memory of their C code and reduce the risk of memory leaks and other memory-related issues, thus improving the quality and maintainability of their programs.
The above is the detailed content of How to perform memory management in C++ code?. For more information, please follow other related articles on the PHP Chinese website!