


Implement a highly customizable data transfer framework using PHP trait DTO
Use PHP trait DTO to implement a highly customizable data transfer framework
As websites and applications become more and more complex, data transfer becomes more and more complex important. In PHP, using Data Transfer Object (DTO) to handle data transfer can greatly simplify the code and improve maintainability and scalability. This article will introduce how to use PHP traits and DTO to implement a highly customizable data transfer framework, and provide corresponding code examples.
First, we need to define a basic DTO class. This class will serve as a base class for other DTO classes and provide some basic properties and methods. In this base class, we will use PHP traits to provide some common functions, such as attribute assignment, attribute reading, attribute verification, etc. The following is a sample code of a basic DTO class:
/** * 基本的DTO类 */ abstract class BaseDTO { /** * 属性赋值 */ public function assign(array $data) { foreach ($data as $key => $value) { if (property_exists($this, $key)) { $this->{$key} = $value; } } } /** * 属性读取 */ public function get($property) { if (property_exists($this, $property)) { return $this->{$property}; } return null; } /** * 属性校验 */ public function has($property) { return property_exists($this, $property); } }
Next, we can create subclasses according to specific business needs and inherit basic functions by inheriting the base class. In the subclass, we can add more properties and methods as needed. The following is the code of an example subclass:
/** * 示例子类 */ class UserDTO extends BaseDTO { /** * 属性定义 */ public $id; public $name; public $email; /** * 自定义方法 */ public function isEmailValid() { return filter_var($this->email, FILTER_VALIDATE_EMAIL); } }
By inheriting the base class, the subclass will automatically inherit the attribute assignment, attribute reading and attribute verification functions in the base class. We can also add custom methods in subclasses to meet specific business needs.
Using the above DTO class, we can easily handle data transmission in business logic. The following is an example usage scenario:
// 创建DTO对象 $userDTO = new UserDTO(); // 属性赋值 $userDTO->assign([ 'id' => 1, 'name' => '张三', 'email' => 'zhangsan@example.com' ]); // 属性读取 $userName = $userDTO->get('name'); $emailValid = $userDTO->isEmailValid();
Through the above code, we can see that using DTO to handle data transmission is very simple and intuitive. By inheriting the base class, we can obtain basic functions, and by extending the subclass, we can customize the DTO class according to specific business needs. This modular design approach not only improves the reusability and maintainability of the code, but also meets the need for high customizability in PHP development.
To sum up, by using PHP traits and DTO, we can implement a highly customizable data transmission framework. By inheriting base classes and using subclasses, we can easily handle data transmission, improving development efficiency while keeping the code clean and maintainable.
The above is the detailed content of Implement a highly customizable data transfer framework using PHP trait DTO. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


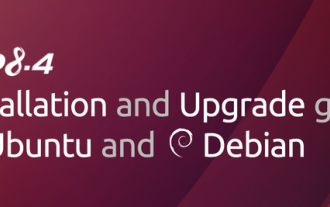
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
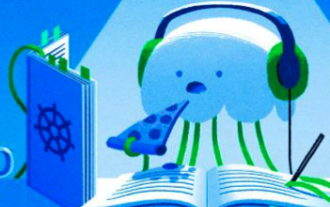
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
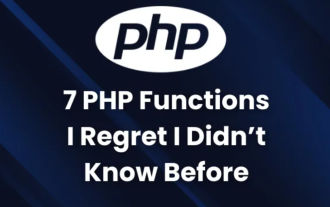
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
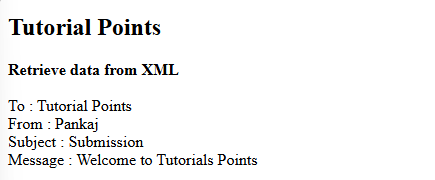
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
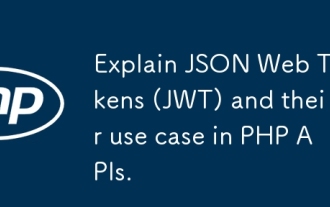
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
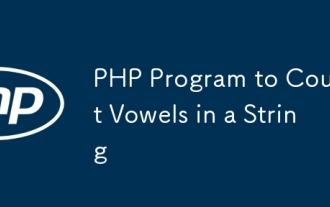
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
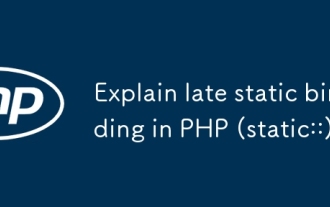
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
