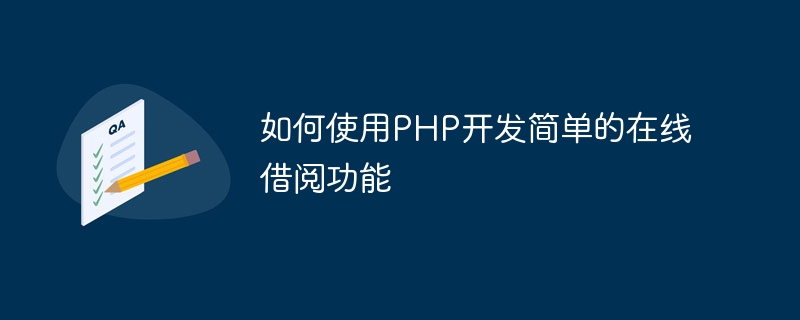
How to use PHP to develop a simple online borrowing function
With the rapid development of the Internet, the online borrowing function has become an integral part of more and more libraries and reading rooms essential features. Through the online borrowing function, users can quickly and easily query relevant information about target books, and implement operations such as reservation borrowing, renewal, and return. In this article, we will explain in detail how to use PHP to develop a simple online lending function and provide specific code examples.
- System design and database construction
First of all, we need to design the basic requirements of the system and build the corresponding database. The online lending system can be divided into the following key modules:
- Book management: including functions such as querying, adding, and deleting books.
- User management: including user registration, login, personal information modification and other functions.
- Borrowing management: including functions such as adding, querying, renewing, and returning borrowing records.
In terms of database, we need to design at least the following tables:
- books: stores book information, including book title, author, publisher, ISBN number, etc.
- users: Store user information, including user name, password, email, etc.
- borrow_records: Stores borrowing records, including books borrowed by users, borrowing dates, return dates, etc.
- User registration and login function
User registration and login function are the basis of the online borrowing system. We can use HTML forms and PHP back-end scripts to achieve this.
The specific steps are as follows:
(1) Create a registration page (register.html) and a login page (login.html), and include corresponding form fields (such as user name, password, etc.).
(2) In the backend, write PHP scripts to process the data submitted by the form and interact with the database. For example, when a user submits a registration form, the backend script should first validate the input data and then insert the user information into the users table.
(3) For the login function, the back-end script should verify the user name and password submitted by the user and check whether they exist in the users table. If the verification is passed, the user information can be saved in the session for subsequent use.
- Book query and management function
One of the core functions of the online lending system is book query and management. Users can search for books based on keywords and view related information.
The specific steps are as follows:
(1) Create a book search page (search.php), including an input box and a submit button.
(2) On the back end, write a PHP script to accept and process the keyword parameters passed by the front end. Use SQL statements to query matching books and return the results to the front-end for display.
(3) For the book management function, you can add a book management page (admin.php) in the background, including operations such as adding and deleting books. Likewise, corresponding PHP scripts need to be written to interact with the database.
- Borrowing record management function
Another important function of the online borrowing system is the management of borrowing records. Users can view their borrowing records and perform operations such as renewal and return.
The specific steps are as follows:
(1) Create a borrowing record query page (borrow.php), where users can view their borrowing records.
(2) At the back end, write a PHP script to accept and process the user information passed by the front end, query the user's borrowing record, and return the results to the front end for display.
(3) In the borrowing record management page, operations such as renewal and return can be provided. Likewise, corresponding PHP scripts need to be written to interact with the database.
To sum up, we use PHP to develop a simple online borrowing function, which includes user registration and login, book query and management, and borrowing record management. Through reasonable system design and database construction, combined with the writing of PHP back-end scripts, we can quickly implement a simple and practical online lending system.
Reference code example:
// 连接数据库
$host = 'localhost';
$dbname = 'library';
$username = 'root';
$password = '';
try {
$conn = new PDO("mysql:host=$host;dbname=$dbname", $username, $password);
} catch(PDOException $e) {
echo "数据库连接失败: " . $e->getMessage();
exit;
}
// 用户注册
$username = $_POST['username'];
$password = $_POST['password'];
$stmt = $conn->prepare("INSERT INTO users (username, password) VALUES (?, ?)");
$stmt->bindParam(1, $username);
$stmt->bindParam(2, $password);
if($stmt->execute()) {
echo "注册成功";
} else {
echo "注册失败";
}
// 用户登录
$username = $_POST['username'];
$password = $_POST['password'];
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ? AND password = ?");
$stmt->bindParam(1, $username);
$stmt->bindParam(2, $password);
$stmt->execute();
if($stmt->rowCount() > 0) {
echo "登录成功";
} else {
echo "用户名或密码错误";
}
// 图书查询
$keyword = $_GET['keyword'];
$stmt = $conn->prepare("SELECT * FROM books WHERE title LIKE ?");
$stmt->bindValue(1, "%$keyword%");
$stmt->execute();
while($row = $stmt->fetch(PDO::FETCH_ASSOC)) {
echo $row['title'];
}
// 借阅记录查询
$user_id = $_SESSION['user_id'];
$stmt = $conn->prepare("SELECT * FROM borrow_records WHERE user_id = ?");
$stmt->bindParam(1, $user_id);
$stmt->execute();
while($row = $stmt->fetch(PDO::FETCH_ASSOC)) {
echo $row['book_id'];
}
Copy after login
The above are the main steps and code examples for developing a simple online borrowing function using PHP. Developers can make appropriate adjustments and expansions based on actual needs to achieve a more complete and stable online lending system.
The above is the detailed content of How to use PHP to develop a simple online borrowing function. For more information, please follow other related articles on the PHP Chinese website!