Have you noticed that in the case codes in our previous articles, each There are some similar codes in the templates of each case. These codes are our setup function, but as the entry function of the combined API, all our combined APIs must be written in it. Do we have to write this code every time? Well, Vue actually provides syntactic sugar for setup. Does everyone know what syntactic sugar is?
For example, v-model in our Vue2 is just syntax sugar. You can save a lot of two-way data binding code through such a command! Then let’s take a look at how our setup can be simplified. Taking the following code as an example, we declare a function and click the button to trigger a simple effect such as printing hi;
<template> <div> <button @click="hello">hello</button> </div> </template> <script> export default { setup() { const hello = () => { console.log('hi') } return { hello } } } </script>
<template>
<div>
<button @click="hello">hello</button>
</div>
</template>
<script setup>
const hello = () => {
console.log('hi')
}
</script>
Copy after login The above is the code effect after we use setup syntax sugar. The functional implementation is the same; in the script setup tag, all data and functions can be used directly in the template!
The top-level variables in script setup can be used directly in the template
computed function
Usage of computed function : In fact, under what circumstances will we use calculated properties? It must be to obtain new data through dependent data!
1) Introduce computed from Vue
2) Use it in setup, use a function, the return value of the function is the calculated data
3) Finally, return it through setup, template Use it. If you use setup syntax sugar, this step is not needed.
We can give a simple example. For example, if we define a score number, pure score information, then we use the computed function to calculate it for us. More than 60 passing results were obtained; we directly used the script setup method to code!
<template>
<div>
<p>成绩单</p>
<a v-for="num in achievement"> {{ num }} / </a>
<p>及格成绩单</p>
<a v-for="num in passList"> {{ num }} / </a>
</div>
</template>
<script setup>
import { computed, ref } from 'vue';
const achievement = ref([44, 22, 66, 77, 99, 88, 70, 21])
const passList = computed(() => {
return achievement.value.filter(item => item > 60)
})
</script>
Copy after login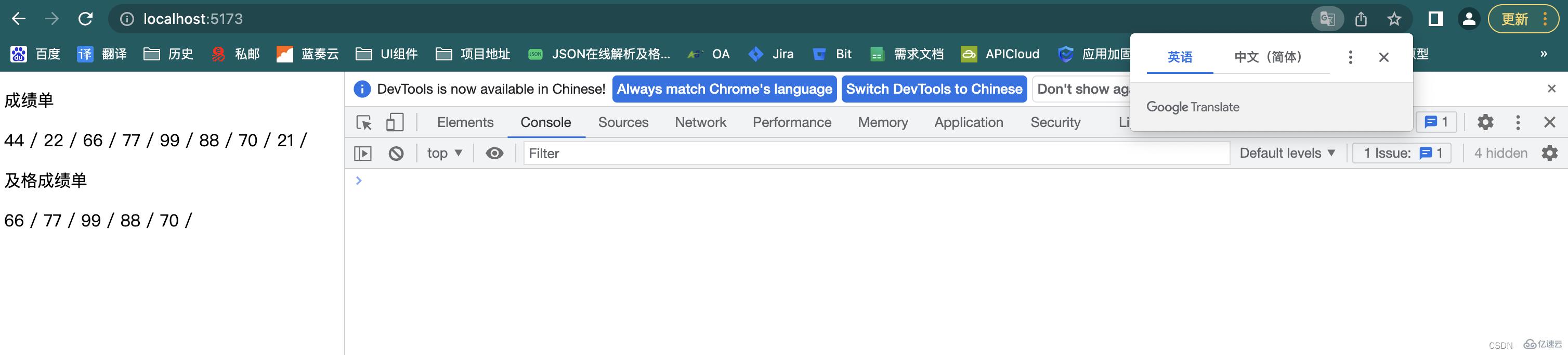
watch function
Like the computed function, the watch function is also a member of the combined API. Watch is actually monitoring. Data changing function, so what are its uses in Vue3? You can use watch to monitor one or more responsive data, you can use watch to monitor an attribute in the responsive data (simple data or complex data), you can configure deep monitoring, or you can use watch monitoring to implement default execution; let's try the code separately. How to write
Monitor a data through watch
watcha monitors a data, the function has two parameters: the first data to be monitored, the second parameter It is a callback function triggered after the monitoring value changes. The callback function also has two parameters, new value and old value.
<template>
<div>
总赞数:{{ num }} <button @click="num++">点赞</button>
</div>
</template>
<script setup>
import { ref, watch } from 'vue';
//创建一个响应式数据,我们通过点赞按钮改变num的值
const num = ref(0)
watch(num, (nv, ov) => {
console.log(nv, ov)
})
</script>
Copy after login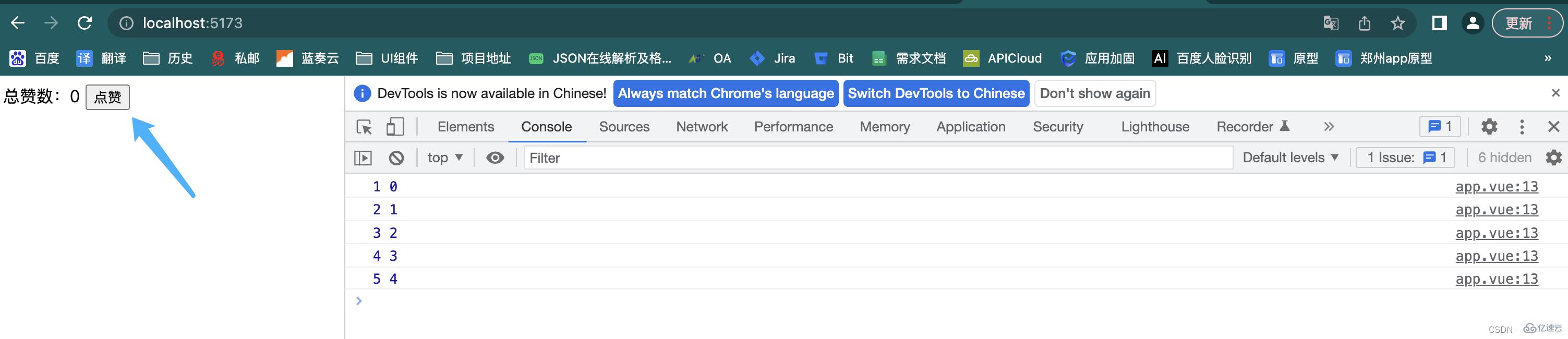
Monitor multiple values through watch Data
watcha monitors multiple data. For example, below we need to monitor changes in num and user objects. The function has two parameters: the first data to be monitored (because it is multiple data So use an array), the second parameter is the callback function that is triggered when the listening value changes.
<template>
<div>
总赞数:{{ num }} <button @click="num++">点赞</button>
</div>
<p>姓名:{{ user.name }}</p>
<p>年龄:{{ user.age }}</p>
<button @click="user.age++">过年啦</button>
</template>
<script setup>
import { ref, watch, reactive } from 'vue';
const num = ref(0)
let user = reactive(
{
name: "几何心凉",
age: 18
}
)
watch([num, user], () => {
console.log('我监听到了')
})
</script>
Copy after login
Listen to a property of the object through watch (simple type)
watch to listen to a property of the object Attributes are of a simple type. For example, if we monitor the age value in the user below, which is a simple type, then the first parameter form of our watch needs to be a function that uses the object attribute as the return value; the second parameter is The changed callback function.
<template>
<p>姓名:{{ user.name }}</p>
<p>年龄:{{ user.age }}</p>
<button @click="user.age++">过年啦</button>
</template>
<script setup>
import { ref, watch, reactive } from 'vue';
let user = reactive(
{
name: "几何心凉",
age: 18
}
)
watch(()=>user.age, () => {
console.log('我监听到了user.age的变化')
})
</script>
Copy after login
Listen to a property of the object through watch (complex type)
watch to listen to a property of the object Attributes are complex types. For example, below we want to monitor the info in user. We try to change the wages value in info in user. Then the first parameter form of our watch needs to be a function that uses the object attribute as the return value. ;The second parameter is the changed callback function. At this time, a third parameter is needed, which is deep to enable deep monitoring
<template>
<p>姓名:{{ user.name }}</p>
<p>年龄:{{ user.age }}</p>
<p>薪资:{{ user.info.wages }}</p>
<button @click="user.age++">过年啦</button>
<button @click="user.info.wages+=2000">加薪了</button>
</template>
<script setup>
import { ref, watch, reactive } from 'vue';
let user = reactive(
{
name: "几何心凉",
age: 18,
info:{
wages:20000
}
}
)
watch(()=>user.info, () => {
console.log('我监听到了user.info的变化')
},{
deep:true
})
</script>
Copy after login
##Monitoring data through watch is executed by default
In fact, there are not many such cases, but we will also encounter this situation. When we monitor data changes, we execute it once by default; in fact, we add our immediate parameter to true, and we use the initial num as Example Ha!
<template>
<div>
总赞数:{{ num }} <button @click="num++">点赞</button>
</div>
</template>
<script setup>
import { ref, watch, reactive } from 'vue';
const num = ref(0)
watch(num, () => {
console.log('我打印了')
},{
immediate:true
})
</script>
Copy after login
The above is the detailed content of How to use setup syntax sugar, computed function, and watch function in Vue3. For more information, please follow other related articles on the PHP Chinese website!