Popular Headlines
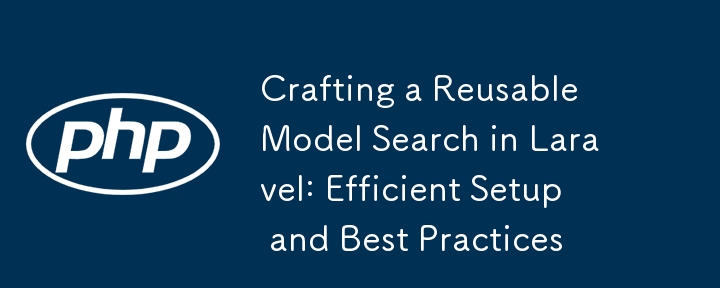
- Building a multi-tenant application with honeystone/contextNot to be confused with Laravel’s new context library, this package can be used to build multi-context multi-tenant applications. Most multi-tenant libraries essentially have a single ‘tenant’ context, so if you need multiple contexts, things can get
- The journey to building large-scale language models in 20242024 will see a technological leap forward in large language models (LLMs), as researchers and engineers continue to push the boundaries of natural language processing. These parameter-rich LLMs are revolutionizing how we interact with machines, enabling more natural conversations, code generation, and complex reasoning. However, building these behemoths is no easy task, involving the complexity of data preparation, advanced training techniques, and scalable inference. This review delves into the technical details required to build LLMs, covering recent advances from data sourcing to training innovations and alignment strategies.
- Growing Success: How to Strategically Retire Products in the Digital AgeAs the wave of digitalization sweeps through product markets, innovation happens at lightning speed. Product managers drive growth by relentlessly pursuing innovation, launching new products, and capturing new markets. What happens when a job is no longer engaging or exciting, such as retiring a product? How to motivate the team to take on the mission of retiring the product gracefully? This article will explore the key steps and considerations for product managers when bidding farewell to a product, providing a comprehensive guide to the sunset journey.
- IP Geolocation Myths and FactsIP geolocation is a technology used to obtain geographical location information, such as country, city, longitude and latitude, etc., by accessing the IP address of a device. This technology is widely used in areas such as website content customization, ad targeting, network security, and user analysis. It works by querying large databases containing IP addresses and geolocation mappings, but there are some pitfalls around accuracy, personal privacy and cost. Understanding these myths is critical for developers and users to make informed decisions when using IP geolocation.
- SSE and WebSocketIn this article, we will compare Server Sent Events (SSE) and WebSockets, both of which are reliable methods for delivering data. We will analyze them in eight aspects, including communication direction, underlying protocol, security, ease of use, performance, message structure, ease of use, and testing tools. A comparison of these aspects is summarized as follows: Category Server Sent Event (SSE) WebSocket Communication Direction Unidirectional Bidirectional Underlying Protocol HTTP WebSocket Protocol Security Same as HTTP Existing security vulnerabilities Ease of use Setup Simple setup Complex performance Fast message sending speed Affected by message processing and connection management Message structure Plain text or binary Ease of use Widely available Helpful for WebSocket integration
Popular Topics
- Douyin level price list 1-75Douyin level price comparison table: 1, level 1 = 0.5 yuan; level 2, 2 = 1 yuan; level 3, 3 = 2 yuan; level 4, 4 = 3 yuan; level 5, 5 = 5 yuan; 6, 6 Level = 7 yuan; Level 7 and 7 = 9 yuan; Level 8 and 8 = 13 yuan; Level 9 and 9 = 18 yuan; Level 10 and 10 = 24 yuan and so on. This topic provides you with relevant articles, downloads, and course content for you to download and experience for free.
- wifi shows no ip assignedSolution to wifi showing no IP allocation: 1. Restart the device and router, turn off the Wi-Fi connection on the device, turn off the device, turn off the router, wait a few minutes, then reopen the router to connect to wifi; 2. Check the router settings and restart DHCP, make sure the DHCP function is enabled; 3. Reset network settings, which will delete all saved WiFi networks and passwords. Please make sure they are backed up before performing this operation; 4. Update the router firmware, log in to the router management interface, and find the firmware Update options and follow the prompts. This topic provides you with various wifi-related articles, downloads and courses. I hope
- Virtual mobile phone number to receive verification codeTo receive the verification code with a virtual mobile phone number: first enter the Yima verification code receiving platform; then register as a website member; then open the SMS verification code service and select an operator; finally obtain the virtual mobile phone number, go to the platform where the verification code is to be sent, and put the phone Fill in the number and select [Send Verification Code].
- How to turn off windows security centerWindows Security Center is a comprehensive security control panel for Windows systems, including firewall status prompts, anti-virus software status prompts, automatic update prompts and other basic system security information. So how to turn off Windows Security Center? I believe many friends are still not clear about it. PHP Chinese website has brought you relevant tutorials and tutorials. You are welcome to come and learn and read.
- myfreemp3Myfreemp3 is a free online music search engine that allows users to search and play music directly on the web. Myfreemp3 has the advantages of fast search engine and rich resources. PHP Chinese website has compiled related articles and APP downloads about myfreemp3 for everyone. etc.
IntelligentAIQ&A
Ask
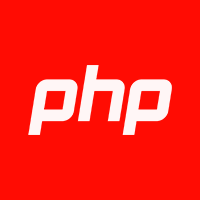
【Learning PHP】
PHP is a widely used open source multi-purpose scripting language. Compared with other technologies, PHP itself is open source and free; the program can be embedded in HTML for execution, and the execution efficiency is much higher than CGI that completely generates HTML tags. It runs on the server side, consumes very few system resources, has strong cross-platform and high efficiency features, and PHP supports almost all popular databases and operating systems. The most important thing is that PHP can also use C and C for program expansion. , PHP language has a very wide range of applications in WEB development. In addition, object-oriented has been greatly improved in PHP4 and PHP5, and can also be used to develop large-scale commercial programs.
- php array_diff_assoc() function
- php array_merge_recursive() function
- TCP/IP mail
- TCP/IP protocol
- TCP/IP addressing
- Web Forms - Database Connection
- Web Forms - ArrayList object
- Web Forms - Button control
- Web Forms - TextBox control
- Web Forms - HTML forms
- Web Forms - Events
- ionic Toggle
- ionic forms and input boxes
- ionic list
- ionic button
- ionic bottom
- ionic head
- MVC - Model
- MVC-SQL Database
- MVC - View
- Intermediate The latest ThinkPHP 5.1 world premiere video tutorial (60 days to become a PHP expert online training course)
-
1394784 times of learning
- Free
- Elementary PHP introductory tutorial one: Learn PHP in one week
-
4206471 times of learning
- Free
- Elementary PHP complete self-study manual
-
2272250 times of learning
- Free
- Intermediate Dugu Jiujian (4)_PHP video tutorial
-
1206562 times of learning
- Free
- Hot
- New
- Follow
- web3.0
- Backend Development
- Web Front-end
- Database
- Operation and Maintenance
- Development Tools
- PHP Framework
- Daily Programming
- WeChat Applet
- Common Problem
- Other
- Tech
- CMS Tutorial
- Java
- System Tutorial
- Computer Tutorials
- Hardware Tutorial
- Mobile Tutorial
- Software Tutorial
- Mobile Game Tutorial
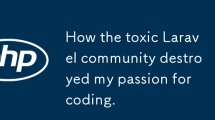
How the toxic Laravel community destroyed my passion for coding.
I still remember it like it was yesterday, but it was over two decades ago when I embarked upon my journey of becoming a web developer. I dialled up on my 56k modem, hogging up the phone line so I could browse some of my favourite websites. Then I w
2024-08-16 18:42:45
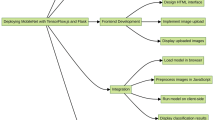
Recommended Project: Deploying MobileNet with TensorFlow.js and Flask
Unlock the power of machine learning in your web applications with this comprehensive project from LabEx. In this hands-on course, you'll learn how to deploy a pre-trained MobileNetV2 model using TensorFlow.js within a Flask web application, enabling
2024-08-16 18:04:09
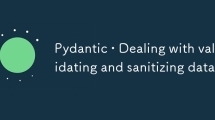
Pydantic • Dealing with validating and sanitizing data
Since I started programming, I've mostly used structured and procedural paradigms, as my tasks required more practical and direct solutions. When working with data extraction, I had to shift to new paradigms to achieve a more organized code. A examp
2024-08-16 18:03:08

Step-by-Step Guide: Loading a HuggingFace ControlNet Dataset from a Local Path
Huggingface provides different options for loading a dataset. When loading a local image dataset for your ControlNet, it's important to consider aspects such as dataset structure, file paths, and compatibility with Huggingface's data handling tools.
2024-08-16 18:02:36
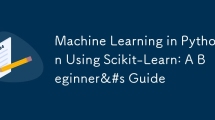
Machine Learning in Python Using Scikit-Learn: A Beginners Guide
Are you interested in learning about machine learning using Python? Look no further than the Scikit-Learn library! This popular python library is designed for efficient data mining, analysis, and model building. In this guide, we will introduce you t
2024-08-16 18:02:33
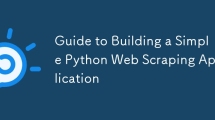
Guide to Building a Simple Python Web Scraping Application
Scraping web data in Python usually involves sending HTTP requests to the target website and parsing the returned HTML or JSON data. Below is an example of a simple web scraping application that uses the requests library to send HTTP requests and u
2024-08-16 18:02:12
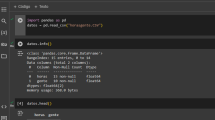
Making something new in the work
Hello to everyone in the DEV community. As you might imagine, customer service can be a bit dull at times, and I try to shake off those dull moments with some fun, exercise, and (yes, it's a bit unusual) a bit of work. Well, combining these three ele
2024-08-16 18:02:03
More
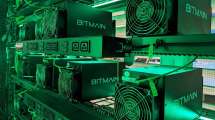
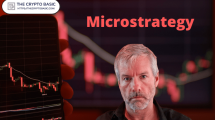

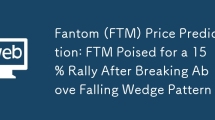
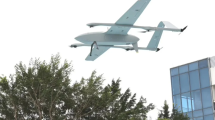
Shanghai issued a document to support the development of low-altitude economy and accelerate the development of commercial manned urban air transportation
According to news from this site on August 16, Shanghai today issued the "Shanghai Action Plan for the High-Quality Development of Low-altitude Economic Industries (2024-2027)". The goal is to establish a complete industrial system of R&D, design, assembly and manufacturing, airworthiness testing, and commercial application of new low-altitude aircraft by 2027. The main goals attached to this site are as follows: It is mentioned among them to support the development of more than 10 leading companies in the R&D and manufacturing of electric vertical take-off and landing aircraft, industrial drones and new energy general aviation aircraft, and to cultivate about 20 leading companies in low-altitude operation services, 3- Five industry-leading airworthiness certification technical service organizations have gathered more than 100 key supporting companies to build a full industry chain of R&D and manufacturing, airworthiness certification, flight services, and scenario applications. Create more than 30 iconic products, covering the entire machine
2024-08-17 00:05:33
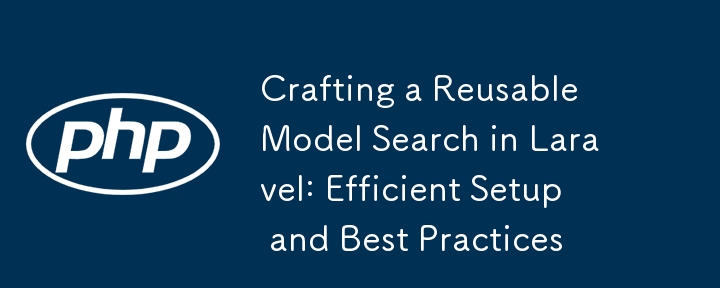
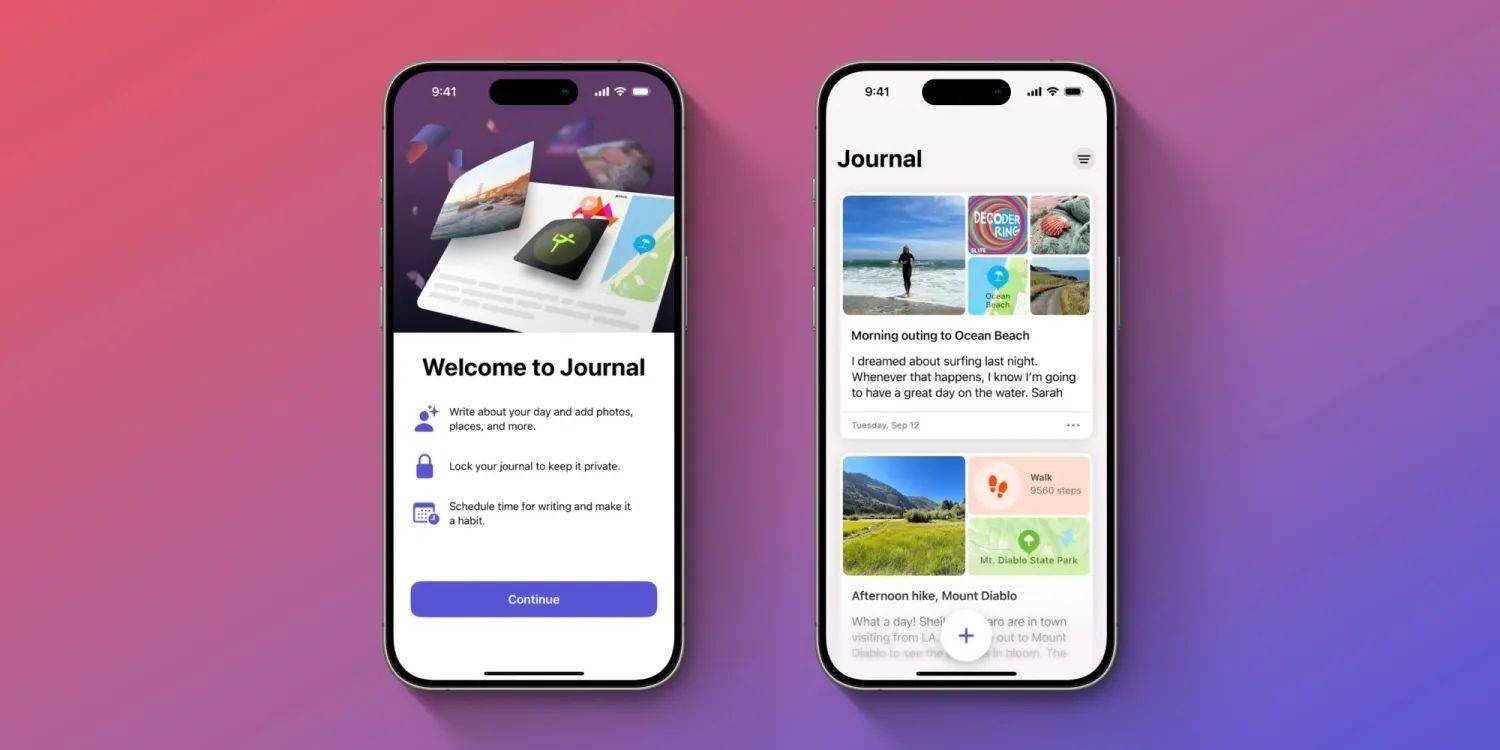
MoreArticle
![[Web front-end] Node.js quick start](https://img.php.cn/upload/course/000/000/067/662b5d34ba7c0227.png?x-oss-process=image/resize,m_fill,h_134,w_220)
- Elementary [Web front-end] Node.js quick start
-
3096
- Free
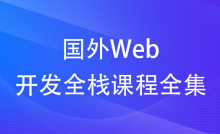
- Elementary Complete collection of foreign web development full-stack courses
-
2481
- Free
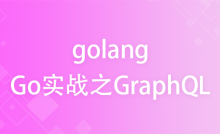
- Elementary Go language practical GraphQL
-
1965
- Free
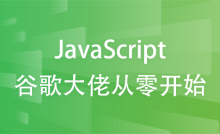
- Elementary 550W fan master learns JavaScript from scratch step by step
-
464
- Free
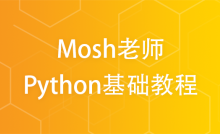
- Elementary Python master Mosh, a beginner with zero basic knowledge can get started in 6 hours
-
10707
- Free
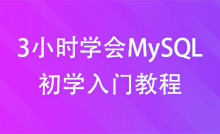
- Elementary Getting Started with MySQL (Teacher mosh)
-
805
- Free
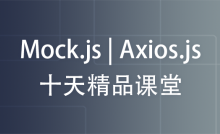
- Elementary Mock.js | Axios.js | Json | Ajax--Ten days of quality class
-
1523
- Free
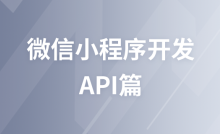
- Elementary WeChat Mini Program Development API Chapter
-
3837
- Free
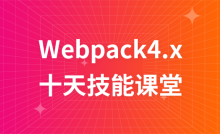
- Elementary Webpack4.x---Ten-day skills class
-
4664
- Free
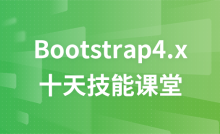
- Elementary Bootstrap4.x---Ten days of quality class
-
5466
- Free
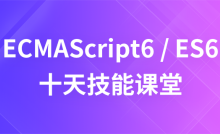
- Elementary ECMAScript6/ES6---Ten-day skills class
-
6018
- Free
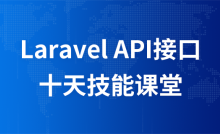
- Elementary Laravel---API interface
-
2204
- Free
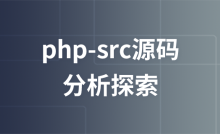
- Elementary php-src source code analysis and exploration
-
1353
- Free
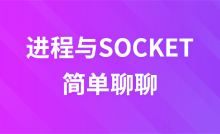
- Elementary Processes and SOCKETs
-
1093
- Free
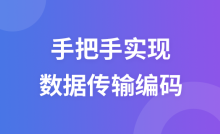
- Elementary Step by step implementation of data transmission encoding
-
361
- Free
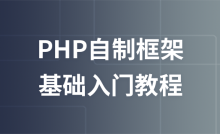
- Elementary PHP self-made framework
-
2381
- Free
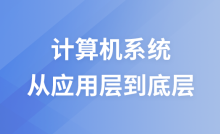
- Elementary Computer system from application layer to bottom layer
-
1479
- Free
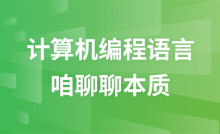
- Elementary Briefly talk about the nature of computer programming languages
-
292
- Free
-
0 Like0 answer930 Views
-
0 Like0 answer1006 Views
-
0 Like0 answer923 Views
-
0 Like0 answer782 Views
-
0 Like0 answer862 Views
-
0 Like0 answer4348 Viewshe" class="wdrCon aClass font33">he
凡人 2024-04-1609:34Ask
1 Like1 answer1051 ViewsI have a URL parameter that contains the category ID and I want to treat it as an array like this: http://example.com?cat[]=3,9,13 In PHP I use Getting the array: $catIDs=$_GET['cat']; When I do echogettype($catIDs); it shows that it is actually treated as an array, but when I do print_r($catIDs); I get the following Result: Array([0]=>3,9,13)P粉785905797 2024-04-0622:09Ask
Shanghai issued a document to support the development of low-altitude economy and accelerate the development of commercial manned urban air transportation According to news from this site on August 16, Shanghai today issued the "Shanghai Action Plan for the High-Quality Development of Low-altitude Economic Industries (2024-2027)". The goal is to establish a complete industrial system of R&D, design, assembly and manufacturing, airworthiness testing, and commercial application of new low-altitude aircraft by 2027. The main goals attached to this site are as follows: It is mentioned among them to support the development of more than 10 leading companies in the R&D and manufacturing of electric vertical take-off and landing aircraft, industrial drones and new energy general aviation aircraft, and to cultivate about 20 leading companies in low-altitude operation services, 3- Five industry-leading airworthiness certification technical service organizations have gathered more than 100 key supporting companies to build a full industry chain of R&D and manufacturing, airworthiness certification, flight services, and scenario applications. Create more than 30 iconic products, covering the entire machine2024-08-17 00:05:33Hikvision's revenue in the first half of 2024 was 41.209 billion yuan, a year-on-year increase of 9.68% According to news from this website on August 16, this evening, Hikvision announced its financial report for the first half of 2024. In the first half of this year, Hikvision achieved revenue of 41.209 billion yuan, a year-on-year increase of 9.68%. The overall revenue of entrepreneurial new business reached 10.328 billion yuan, a year-on-year increase of 26.13%, accounting for 25.06% of total revenue. Main financial data of Hikvision: Revenue in the first half of the year was 41.209 billion yuan, a year-on-year increase of 9.68%. Profit: Net profit attributable to shareholders of listed companies in the first half of the year was 5.064 billion yuan, a year-on-year decrease of 5.13%; net profit after deducting non-recurring gains and losses in the first half of the year was 5.243 billion yuan, a year-on-year increase of 4.11%. Cash flow: net cash flow generated from operating activities -1.2024-08-17 00:00:33Xiaohongshu announced adjustments to its rank system: removing 'seniority ranking” and simplifying management levels Xiaohongshu adjusts the ranking system. On August 16, Xiaohongshu announced that it would no longer set up ranks and simplify the management level. This move aims to eliminate seniority and create an agile organization focused on creating value for users, customers and merchants. Adjustments include: no longer setting up the R rank, simplifying the management level, adopting a new appointment system for leaders at all levels, and focusing more on: quickly discovering outstanding talents, flexibly matching business development, stimulating front-line vitality, allowing outstanding employees to stand out, and implementing the new system of "giving self-motivation a battlefield and achieving success." The concept of "return" matches Xiaohongshu's current business development. Recently, Xiaohongshu promoted a group of new leaders. After the adjustment of the rank system: Salary is directly linked to work difficulty. Bonuses are linked to work results. According to industry headhunting analysis, this move unbundles incentives and rank promotions, and timely incentives are tied.2024-08-17 00:00:11Designing antibodies from scratch, Tencent and Peking University teams pre-trained large language models and published them in Nature sub-journal Editor | KXAI technology has made tremendous progress in assisting antibody design. However, antibody design still relies heavily on isolation of antigen-specific antibodies from serum, which is a resource-intensive and time-consuming process. To solve this problem, research teams from Tencent AILab, Peking University Shenzhen Graduate School, and Xijing Digestive Disease Hospital proposed a pre-trained antibody generation large language model (PALM-H3) for de novo generation of artificial antibodies with required antigen-binding specificity. Antibody CDRH3, reducing dependence on natural antibodies. In addition, a high-precision antigen-antibody binding prediction model A2binder was designed to pair the antigen epitope sequence with the antibody sequence to predict binding specificity and affinity. In summary, this study established a framework for anti-2024-08-16 22:32:03Why stick to the human form? Yunji Technology is betting on the composite polymorphic robot 'UP': Let embodied intelligence enter people's lives For Yunji Technology, which was the first to lay out the commercial service robot track, it has conquered the market in the hotel industry, making delivery robots a "partner" in our daily lives. While embodied intelligence players are exploring how to find scenarios and how to make products continue to exert value, Yunji Technology has once again explored a new path. "Ideal AI should allow machines to think like humans, products to work like humans, and be combined with specific scenario applications to solve practical problems end-to-end and create value. The Yunji Technology robot with the evolution of AI+embodied intelligence has already done so. At this point. One of its core is to let the robot be able to use tools, understand reasoning, assign tasks, and realize swarm intelligence” Yunji Technology CSilk Road Bitcoin (BTC) Stash May Be Unwound as US Government Negotiates or Sells Coins Bitcoin (BTC) may feel additional pressure from Silk Road's potential unwinding of its wallet. For now, there are no direct signs of selling, but the coin's specific segregated wallet raises suspicions.2024-08-17 00:03:10- WIKINDX Reference management, document management, citations and more. Designed by scholars for scholars, continuously developed since 2003 and used by individuals and major research institutions worldwide, WIKINDX is a virtual research environment (enhanced online literature manager) that stores searchable references, notes, documents, Quotes, thoughts, etc. The integrated WYSIWYG word processor exports formatted articles to RTF and HTML. Plug-ins include a citation style editor and import/export of references (BibTeX, Endnote, RIS, etc.). WIKINDX supports every reference text
- phpList phpList provides open source email marketing services, including analysis, list segmentation, content personalization and bounce processing. The rich technical functions and secure and stable code base are the result of 17 years of continuous development. Used in 95 countries, available in more than 20 languages, and used in 25 billion email campaigns sent last year. You can deploy it with your own SMTP server or get a free hosting account at http://phplist.com.
- SecLists SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
- DVWA Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
- PHPMailer PHPMailer - A full-featured email creation and delivery class for PHP. Supports UTF-8 content as well as 8-bit, base64, binary and quoted-printable encodings. Provides SMTP authentication for LOGIN, PLAIN, CRAM-MD5 and XOAUTH2 mechanisms over SMTPS and SMTP+STARTTLS transports. Automatically verify email addresses. Many PHP developers need to send emails from their code. The only PHP function that directly supports this is mai
- Front-end template2024-05-092339112
- vip courseware source code2024-05-09100436
- Front-end template2024-02-29197749
- Front-end template2024-02-28189233
-
2020-03-26214424063
-
2018-09-261135090381
-
2018-01-2636324521
-
2018-01-2656518286
- jQuery enterprise message form contact code jQuery enterprise message form contact code is a simple and practical enterprise message form and contact us introduction page code.form button2024-02-29234729
- HTML5 MP3 music box playback effects HTML5 MP3 music box playback special effect is an mp3 music player based on HTML5 css3 to create cute music box emoticons and click the switch button.Player special effects2024-02-29199528
- HTML5 cool particle animation navigation menu special effects HTML5 cool particle animation navigation menu special effect is a special effect that changes color when the navigation menu is hovered by the mouse.Menu navigation2024-02-29175741
- jQuery visual form drag and drop editing code jQuery visual form drag and drop editing code is a visual form based on jQuery and bootstrap framework.form button2024-02-29175023
- php7.3.8 offline Chinese manual (official version) php7.3.8 official Chinese manual (chm format) Downloaded by42682people in total Recent UpdatesServer side development
- PHP7.2 Chinese manual PHP Chinese manual download|chm|mobile version|pdf Downloaded by41712people in total Recent UpdatesServer side development
- html5 Chinese manual (CHM version) HTML5 Chinese manual download (CHM version) Downloaded by34322people in total Recent UpdatesFront-end development
- php 5.6 Chinese manual PHP 5.6 Chinese manual download Downloaded by27692people in total Recent UpdatesServer side development
- PHP7.2 manual (latest version) php7.2 development manual download CHM version Downloaded by27182people in total Recent UpdatesServer side development
- MySQL 5.1 Reference Manual MySQL 5.1 Reference Manual Download Downloaded by22100people in total Recent Updatesdatabase
- Adobe official Flash animation optimization guide pdf version Tutorials from Adobe's official Flash animation optimization guide, including the following content: How to save memory How to minimize CPU usage How to improve ActionScript 3.0 performance Speed up rendering Speed Optimize network interaction Use audio and video Optimize SQ Downloaded by5people in total Recent UpdatesFront-end development
- Flex 3 CookBook Chinese pdf version A Flex application consists of two language codes: ActionScript and MXML. Since 3.0, ActionScript has evolved from a prototype-based scripting language to a fully object-oriented, strongly typed scripting language that complies with the ECMAScript standard. MXML is a markup language, very similar to the familiar Hypertext Markup Language (HTML) and Extensible Markup Language (XML). This Chinese version of FLEX 3 COOKBOOK is translated by Chang Qing Translation Team, whose main members are Chang Qing and Nigel Downloaded by5people in total Recent UpdatesFront-end development
- Introduction to Adobe Flex Chinese WORD version Flex is a component-based development framework that can generate a rich Internet application run by Flash Player. Flex combines a standards-based language with a variety of extensible user interface and data access components, allowing developers to build applications with rich data presentation, powerful client logic and integrated multimedia. Flex is a rich client application development toolkit built on the Flash platform. As a new technology representative in the Rich Internet Application (RIA) era, Flex has been used by Adobe since 2007. Downloaded by5people in total Recent UpdatesFront-end development
- Flash ActionScript3 Advanced Tutorial pdf version Flash ActionScript3 advanced tutorial pdf, book directory: Chapter 1 Advanced collision detection Detection of collisions of irregular graphics BitmapData.hitTest is used for collision detection of a large number of non-bitmap objects Implementing grid-based collision detection Writing grid code testing and adjusting the grid Grid uses this type of detection not just for collision Chapter 2 Steering Behavior 2D Vector (Vector2D) Class Locomotive (V Downloaded by5people in total Recent UpdatesFront-end development
- Flex development configuration manual Chinese PDF version This document mainly talks about flex (eclipse) development configuration; interested friends can come and take a look. Downloaded by0people in total Recent UpdatesFront-end development
- ActionScript3 design pattern Chinese WORD version This document mainly talks about the ActionScript3 design pattern; I hope this document will help friends in need; interested friends can come and take a look. Downloaded by0people in total Recent UpdatesFront-end development
- Flash commonly used button codes word version Flash Player is a program that can play small and fast multimedia animations, as well as interactive animations, flying logos and images created with Macromedia Flash. This player is very small, takes only a moment to download, and is a great start for experiencing multimedia effects on the web. Flash also supports high-quality mp3 audio streams, text input fields, interactive interfaces, and much more. This latest version can view all flash formats. If you want to watch multimedia content on the web, flash player is almost Downloaded by0people in total Recent UpdatesFront-end development
- Flex Designer Basics English Text PDF Version "Foundation Flex for Designers" English text version [PDF] Downloaded by0people in total Recent UpdatesFront-end development
- Perl learning handbook chm version Perl Learning Handbook is an article written by a Taiwanese Perl master, specially packaged as a chm version for everyone's convenience. About this book 1. About Perl 1.1 History of Perl 1.2 Concepts of Perl 1.3 Features 1.4 Environment for using Perl 1.5 Getting started with Perl 1.6 Your first Perl program 2. Scalar variables (Scalar) 2.1 About scalars 2.1.1 Numeric values 2.1. 2 Strings 2.1.3 Number and string conversion 2.2 Use your own Downloaded by5people in total Recent UpdatesServer side development
- Perl Lwp documentation chm version LWP is the abbreviation of Library for Web access in Perl. The purpose is very clear, it is a Perl package for accessing the Web server. Using the LWP package, we can easily access resources on external web servers in our perl scripts. Why use LWP? Today's website applications are becoming more and more complex. It is simply impossible to simply write a Sockettelnet to obtain resources using the GET command, especially for some websites that require passwords to log in. If you just want to simply get Downloaded by5people in total Recent UpdatesServer side development
- Perl DBI Chinese manual pdf version Perl DBI manual Chinese translation pdf, Perl DBI Chinese help document, covering Perl DBI name, overview, description, DBI classes, processor general methods, general properties, DBI database processing objects, database processing methods, etc. In order to make it easier for everyone to learn, Script House has specially packaged the Chinese translation version of Perl+DBI Programming (chm).chm Perl+DBI Programming-[US] Descartes-China Electric Power Press-2001.pdf Perl.DBI Manual. pdfProgram Downloaded by5people in total Recent UpdatesServer side development
- Install perl module under linux Chinese WORD version This document mainly talks about installing the perl module under Linux; Linux and perl are both free software, and it is really wonderful to combine the two. I hope this document will help friends in need; interested friends can come and take a look. Downloaded by5people in total Recent UpdatesServer side development
- Ruby skills Chinese WORD version Ruby is a simple and fast object-oriented (object-oriented programming) scripting language. This document introduces the skills of Ruby in detail; interested friends can come and take a look. Downloaded by0people in total Recent UpdatesServer side development
- Summary of three major techniques for performance optimization of programming language Perl Chinese WORD version This article will focus on discussing Perl performance optimization techniques. When using Perl to develop some service applications, sometimes you will encounter problems with Perl performance or resource usage. You can use require to load modules, use system functions and XS-based modules, and write yourself with low overhead. Modules, etc. to optimize Perl performance. Perl is a powerful language, a powerful tool, and a very delicious dish:-) Using many of Perl's features, you can achieve some very interesting and practical functions. I hope this document will help friends in need; interested friends can come and take a look. Downloaded by0people in total Recent UpdatesServer side development
- go language reference manual Chinese CHM version Go is an open source programming language that makes it easy to build simple, reliable, and efficient software. This article brings you the Go reference manual, you can download it if you need it! Go was developed by Robert Griesemer, Rob Pike, Ken Thompson from the end of 2007, and later joined by Ian Lance Taylor, Russ Cox and others. It was finally open sourced in November 2009 and Go 1 stable was released in early 2012. Version. The development of Go is now fully open Downloaded by3people in total Recent UpdatesServer side development
- Perl basic tutorial chm A basic introductory Chinese tutorial on Perl, in chm format, describing an overview of PERL, simple variables, operators, list and array variables, file reading and writing, pattern matching, control structures, subroutines, associative arrays/hash tables, formatted output, and file systems , reference, object-oriented, packages and modules and other knowledge points. Suitable for beginners to read and understand the Perl scripting language. Downloaded by0people in total Recent UpdatesServer side development
- Summary of information related to Perl DBI programming pdf+chm Script House Special Edition Mainly includes Perl+DBI programming (chm).chmPerl+DBI programming-[US] Descartes-China Electric Power Press-2001.pdfPerl DBI Tutorial on using DBI in Perl, for beginners to refer to the Chinese translation version of Perl.DBI manual .pdfPerl DBI Chinese help document, covering Perl DBI name, overview, description, DBI classes, processor general methods, general attributes, DBI database processing objects, database processing methods, etc. Programming_the_P Downloaded by0people in total Recent UpdatesServer side development
- MySQL 8 Cookbook (Chinese version) (PDF version) MySQL 8 Cookbook (Chinese version) (PDF version) Downloaded by8504people in total Recent Updatesdatabase
- Comprehensive list of commonly used MySQL commands (PDF version) Comprehensive list of commonly used MySQL commands (PDF version) Downloaded by8090people in total Recent Updatesdatabase
- oracle knowledge base oracle knowledge base download Downloaded by2523people in total Recent Updatesdatabase
- Android development tutorials and notes pdf version Android file access and database programming knowledge. File operations mainly include reading files, writing files, reading static files, etc. It also introduces creating, adding file content and saving, opening files and displaying the content; database programming mainly introduces the SQLite database. The use includes knowledge of creating, deleting, opening databases, non-query SQL operation instructions, query SQL instructions-cursors, etc. Downloaded by12people in total Recent UpdatesMobile terminal
- Advanced Bash Scripting Guide chm version This book assumes that you do not have any programming knowledge about scripting or general programming, but if you do, you will easily be able to reach an intermediate to advanced level... All of this is just a small part of the vast knowledge of UNIX® . You can use this book as a textbook, a self-study manual, or a document about shell scripting technology. The exercises in the book and the comments in the sample scripts will better interact with readers, but the most critical premise is: want to The only way to truly learn scripting is to write scripts yourself. This book also Downloaded by5people in total Recent Updatesdevelopment tools
- Unity3d game development camera switching Chinese WORD version This document mainly talks about camera switching in unity3d game development; I hope it will be helpful to everyone; interested friends can come and take a look. Downloaded by6people in total Recent UpdatesOther manuals
- Lucene learning and summary Chinese WORD version This document mainly talks about the learning and summary of Lucene; Lucene is an efficient, Java-based full-text search library. I hope this document will help friends in need; interested friends can come and take a look. Downloaded by5people in total Recent UpdatesOther manuals
24-hour Reading Ranking
- 1People Have Been Wondering If We Can Alter Mars's Climate to Make It Livable for Humans, and We Might Be Closer Than Ever to Answering This Question
- 2TON Blockchain Integrates Injective (INJ) and Pyth Network (PYTH), Further Advancing Telegram's Web3 Reach
- 3What exactly is Hotcoin Exchange like? Are hot currency exchanges safe?
- 4Kobe Bryant logo picture mobile wallpaper
- 5how goodbyedpi operate
- 6Ranking of household water purification equipment?
- 7application launcher.exe - What is application launcher.exe?
- 8People Have Been Wondering If We Can Alter Mars's Climate to Make It Livable for Humans, and We Might Be Closer Than Ever to Answering This Question
- 9TON Blockchain Integrates Injective (INJ) and Pyth Network (PYTH), Further Advancing Telegram's Web3 Reach
Mallsource Code
More>
DownloadRanking
More>
- 1
641340 Download
- 2
227845 Download
- 3
218221 Download
- 4
162285 Download
- 5
141064 Download
- 6
103805 Download
- 7
85965 Download
- 8
71415 Download
- 9
65313 Download
- About us Disclaimer Sitemap
- php.cn:Public welfare online PHP training,Help PHP learners grow quickly!
http://m.scstlh.com/eayabc/
http://m.sdsbkt.com/eayabc/
http://m.sem33.com/eayabc/
http://m.sendraenrich.com/eayabc/
http://m.sgfp123.com/eayabc/
http://m.shangcaifc.com/eayabc/
http://m.shanggh.com/eayabc/
http://m.shanzushou.com/eayabc/
http://m.sharingli.com/eayabc/
http://m.siennasward.com/eayabc/
http://m.sjhealthsystem.com/eayabc/
http://m.smartwinvip.org/eayabc/
http://m.smhongyun.com/eayabc/
http://m.somso368.com/eayabc/
http://m.spunkyisthree.com/eayabc/
http://m.spwzs.com/eayabc/
http://m.sqxhnkyy.com/eayabc/
http://m.stclairws.com/eayabc/
http://m.stylutionusa.com/eayabc/
http://m.susansterner.com/eayabc/
http://m.sxchequan.com/eayabc/
http://m.syqtv.com/eayabc/
http://m.szyisq.com/eayabc/
http://m.tengfeihs.com/eayabc/
http://m.texcalinv.com/eayabc/
http://m.thaifn.com/eayabc/
http://m.thisismypot.com/eayabc/
http://m.tiandalx.com/eayabc/
http://m.tiantianjifen.com/eayabc/
http://m.tianzimy.com/eayabc/
http://m.tiaoweiba.com/eayabc/
http://m.torkax.com/eayabc/
http://m.totaltennisuk.com/eayabc/
http://m.triagin.com/eayabc/
http://m.t-seto.com/eayabc/
http://m.twlfighting.com/eayabc/
http://m.unplu.com/eayabc/
http://m.vacs-system.com/eayabc/
http://m.vector-spaces.com/eayabc/
http://m.vhaohe.com/eayabc/
http://m.vicbnbs.com/eayabc/
http://m.wch21.com/eayabc/
http://m.wdszulin.com/eayabc/
http://m.webmundus.com/eayabc/
http://m.wenaishequ.com/eayabc/
http://m.wifespicture.com/eayabc/
http://m.williamsandward.com/eayabc/
http://m.wlyxkj.com/eayabc/
http://m.wwmsg.com/eayabc/
http://m.wxcsw.net/eayabc/
http://m.xaxcxnjl.com/eayabc/
http://m.xcxys.com/eayabc/
http://m.xenoglass.com/eayabc/
http://m.xinxiqudao.com/eayabc/
http://m.xjrfwy.com/eayabc/
http://m.xtbdy.com/eayabc/
http://m.xungou99.com/eayabc/
http://m.xyschoolife.com/eayabc/
http://m.ybkzhijia.com/eayabc/
http://m.ydcfashion.com/eayabc/
http://m.yiyangwei.com/eayabc/
http://m.yjsmarthome.com/eayabc/
http://m.ymkpr.com/eayabc/
http://m.ymztx.net/eayabc/
http://m.yongxinmifeng.com/eayabc/
http://m.youyongnet.com/eayabc/
http://m.youzhu88.com/eayabc/
http://m.yqs-tattoo.com/eayabc/
http://m.yumi-yumi.com/eayabc/
http://m.yyxjcj.com/eayabc/
http://m.zgflgw.com/eayabc/
http://m.zhenaiabc.com/eayabc/
http://m.zhuoxia.net/eayabc/
http://m.ziggyramone.com/eayabc/
http://m.zsbn.net/eayabc/
http://m.zzfuming.com/eayabc/
http://m.021shtlzy.com/eayabe/
http://m.1gmr.com/eayabe/
http://m.1mao88.com/eayabe/
http://m.1-tg.com/eayabe/
http://m.28d586.com/eayabe/
http://m.28f53.com/eayabe/
http://m.28f588.com/eayabe/
http://m.28i589.com/eayabe/
http://m.28s681.com/eayabe/
http://m.28s687.com/eayabe/
http://m.30811.net/eayabe/
http://m.360kss.com/eayabe/
http://m.411montgomery.com/eayabe/
http://m.911address.com/eayabe/
http://m.911stockalert.com/eayabe/
http://m.91gouhui.com/eayabe/
http://m.91hq.net/eayabe/
http://m.abetteran.com/eayabe/
http://m.adfhair.com/eayabe/
http://m.amc-comm.com/eayabe/
http://m.aolgunchat.com/eayabe/
http://m.aotandz.com/eayabe/
http://m.aprmall.com/eayabe/
http://m.asqxzs.com/eayabe/
http://m.asqxzs.com/eayabe/
- 部落采取措施减缓新的冠状病毒的传播
- 领先的球员希望下一个赛季获得完整的向其开放
- 吃红萝卜可以减肥吗
- 乔·拜登看到了超级星期二的惊人飙升而伯尼·桑德斯撞墙
- 新冠状病毒可在表面存活数小时政府与经济研究
- 免费减肥报
- 抗议者无法接受的暴力袭击使巴黎火车站附近发生大火
- 韦恩·霍克斯赢得了《金拖鞋》
- 穆罕默德·萨拉赫雕像因前锋获得罗纳尔多待遇而受到球迷嘲笑
- 土耳其誓言如果叙利亚休战遭到违反将采取强有力的军事行动
- 青稞粉减肥吗
- 翁吸减肥价格
- 红酒减肥的
- 冠状病毒的影响_说新德里射击世界杯没有排名积分
- 在喀布尔集会的袭击中人丧生
- 本田非洲双胞胎年本田非洲双胞胎推出起价
- 走路半小时可以减肥吗
- 干体力活能减肥吗
- 现在即使是年代以上的板球奖杯也能胜任
- 我和讨论了举动–
- 导演艾哈迈德·汗在称失败后称为宝莱坞英雄
- 为什么打篮球不能减肥
- 减肥健身器械
- 热敷经络减肥
- 减肥期间喝中药会胖吗
- 亿澳元援助方案
- 醇品咖啡减肥
- 南非将在半决赛中面对澳大利亚因为女子世界杯遭雨淋
- 美国公民想推翻美国
- 预定阅读的笔记本
- susuya减肥纤体丸 效果
- dika减肥药怎么吃
- 在年学科排名中继续发光
- 面筋减肥能吃
- 自我欣赏的舒适电影
- 善贝勒减肥药怎么样
- 美食减肥句子
- 西尔斯周五的最后期限
- 尚赫精油减肥
- 一然堂减肥
- 罗志祥减肥励志故事
- 人力资源部要求鉴于推迟所有考试包括
- 假货最新新闻和头条
- 加利福尼亚宣布首例冠状病毒死亡后进入紧急状态
- 美联社事实检查特朗普大肆宣传弹无罪
- 广州减肥伊丽沙白1高端
- 伊人减肥瘦吧
- 怎么减肥不掉头发
- 谁的减肥操好
- 工会发现生命与文化对普拉西多·多明戈的女性不合适
- 印度选举委员会努力解决虚假信息
- 图片迪莎·帕塔尼对粉丝们的免费造型印象深刻
- 这是
- 例新确诊的病例
- 为什么喂母乳可以减肥
- 新西兰大屠杀生命与文化发生一年后穆斯林仍然感到不安全
- 玄米茶减肥功效
- 我将去欧元誓言英格兰队长哈里·凯恩
- 红豆绿豆减肥方法
- 小城市最聪明吗
- 减肥不瘦是什么原因
- 便秘引起的肥胖怎么减肥
- 减肥去油茶
- 神术散 减肥用量
- 启动了数十亿美元的项目
- 可以部署在街上巡逻报告
- 手机减肥器下载
- 齐柏林飞艇在斗争
- 减肥中药胶囊
- 喝汤减肥么
- 减肥能吃意面
- 贝克男孩食品和饮料
- 在冠状病毒封锁期间中国社交媒体充满了欢笑和愤怒
- 喝荷叶茶可以减肥吗
- 14天辟谷减肥
- 伊姆兰的哈姆利特困境
- 尽管双方都说达成协议即将达成
- 孙俪减肥视频
- 深圳中药减肥粉
- 新鲜枣子能减肥吗
- 下一个单身女郎是克莱尔·克劳利
- 怎样梦减肥
- 汗蒸不减肥
- 腿部有肌肉怎么减肥
- 波士顿基于收入的停车罚单的新提议
- 吴君如分食减肥法
- 减肥店减肥可靠吗
- 形美减肥片
- 妻子汤姆·汉克斯冠状病毒检测呈阳性
- 尚无针对冠状病毒威胁的应急计划
- iu喝水减肥
- 德国减肥奶昔
- 跨部门女权主义与性剥削作斗争蒙特利尔人庆祝国际妇女节
- 魔芋的减肥方法
- 减肥哪些东西不能吃
- 荷叶水真的可以减肥吗
- 韩式塞纳减肥
- 几点吃早餐最利于减肥
- 特朗普的访问意图高涨前方有一些坎
- 减肥晚餐牛奶
- 为什么要在月确保特朗普的冬季白宫
- 刚果与致命的艾滋病作斗争埃博拉治疗中心再次遭到袭击
- 减肥好方法体操视频
- 减肥脂肪怎么排出体外
- 老婆突然减肥
- 贫血的人能减肥吗
- 每天只吃一个鸡蛋减肥
- 超过一半的科技股跌至
- 党姐买的减肥药管用吗
- 减肥每天怎么吃
- 金仪轩减肥
- 今天在斋浦尔举行的青年集会上拉胡尔·甘地专注于经济就业
- 翼龙新类别荣权力游戏
- 在冠状病毒大流行期间暂停比赛直到另行通知
- 又子减肥
- 英国法院阻止希思罗机场扩展气候问题_
- 谁来领导工党播客
- 减肥广告语幽默
- 视频很有趣
- 切尔西·泰勒和乔恩·福斯特欢迎儿子文森特·弗兰克
- 喝皮蛋瘦肉粥能减肥吗
- 徐州按摩减肥
- 减肥瘦身果汁
- 特朗普表示如果被问及准备帮助伊朗提供冠状病毒
- 量子减肥
- 平板支撑减肥法
- 割肠子减肥
- 这位婴儿唱着永远是我的宝贝会让你开心
- 埋线减肥大概多少费用
- 火星漫游者有一个新名字毅力
- 消脂减肥膏
- 前卡纳塔克邦挑战莫迪以在克什米尔接待特朗普以证明常态
http://m.sbmmt.com/xrrv/9827465.html
http://m.sbmmt.com/xrrv/864910.html
http://m.sbmmt.com/xrrv/1502394.html
http://m.sbmmt.com/xrrv/828533.html
http://m.sbmmt.com/xrrv/588480.html
http://m.sbmmt.com/xrrv/5683654.html
http://m.sbmmt.com/xrrv/845830.html
http://m.sbmmt.com/xrrv/45426.html
http://m.sbmmt.com/xrrv/10393.html
http://m.sbmmt.com/xrrv/1365383.html
http://m.sbmmt.com/ehjjo/23334.html
http://m.sbmmt.com/ehjjo/56289.html
http://m.sbmmt.com/ehjjo/3226556.html
http://m.sbmmt.com/ehjjo/5530402.html
http://m.sbmmt.com/ehjjo/6598539.html
http://m.sbmmt.com/ehjjo/16110.html
http://m.sbmmt.com/ehjjo/40715.html
http://m.sbmmt.com/ehjjo/658552.html
http://m.sbmmt.com/ehjjo/688854.html
http://m.sbmmt.com/ehjjo/5964712.html
http://m.sbmmt.com/ehjjo/4940820.html
http://m.sbmmt.com/ehjjo/85620.html
http://m.sbmmt.com/ehjjo/68951.html
http://m.sbmmt.com/ehjjo/410369.html
http://m.sbmmt.com/ehjjo/5105438.html
http://m.sbmmt.com/ehjjo/348502.html
http://m.sbmmt.com/ehjjo/7184799.html
http://m.sbmmt.com/ehjjo/308324.html
http://m.sbmmt.com/ehjjo/98629.html
http://m.sbmmt.com/ehjjo/204502.html
http://m.sbmmt.com/ehjjo/7621496.html
http://m.sbmmt.com/ehjjo/625664.html
http://m.sbmmt.com/ehjjo/505224.html
http://m.sbmmt.com/ehjjo/74237.html
http://m.sbmmt.com/ehjjo/497096.html
http://m.sbmmt.com/ehjjo/4422354.html
http://m.sbmmt.com/ehjjo/398091.html
http://m.sbmmt.com/ehjjo/8008536.html
http://m.sbmmt.com/ehjjo/43455.html
http://m.sbmmt.com/ehjjo/8478424.html
http://m.sbmmt.com/ehjjo/507039.html
http://m.sbmmt.com/ehjjo/773298.html
http://m.sbmmt.com/ehjjo/523394.html
http://m.sbmmt.com/ehjjo/256909.html
http://m.sbmmt.com/ehjjo/3277270.html
http://m.sbmmt.com/ehjjo/75935.html
http://m.sbmmt.com/ehjjo/790678.html
http://m.sbmmt.com/ehjjo/50820.html
http://m.sbmmt.com/ehjjo/131551.html
http://m.sbmmt.com/ehjjo/6477072.html
http://m.sbmmt.com/ehjjo/453157.html
http://m.sbmmt.com/ehjjo/88653.html
http://m.sbmmt.com/ehjjo/8848738.html
http://m.sbmmt.com/ehjjo/18209.html
http://m.sbmmt.com/ehjjo/424464.html
http://m.sbmmt.com/ehjjo/494586.html
http://m.sbmmt.com/ehjjo/537376.html
http://m.sbmmt.com/ehjjo/3681870.html
http://m.sbmmt.com/ehjjo/6020842.html
http://m.sbmmt.com/ehjjo/570049.html
http://m.sbmmt.com/ehjjo/310923.html
http://m.sbmmt.com/ehjjo/89118.html
http://m.sbmmt.com/ehjjo/5326418.html
http://m.sbmmt.com/ehjjo/4256424.html
http://m.sbmmt.com/ehjjo/5854371.html
http://m.sbmmt.com/ehjjo/7023733.html
http://m.sbmmt.com/ehjjo/6420028.html
http://m.sbmmt.com/ehjjo/11750.html
http://m.sbmmt.com/ehjjo/346747.html
http://m.sbmmt.com/ehjjo/8324627.html
http://m.sbmmt.com/ehjjo/49970.html
http://m.sbmmt.com/ehjjo/17910.html
http://m.sbmmt.com/ehjjo/5249682.html
http://m.sbmmt.com/ehjjo/956402.html
http://m.sbmmt.com/ehjjo/8760679.html
http://m.sbmmt.com/ehjjo/5886033.html
http://m.sbmmt.com/ehjjo/29991.html
http://m.sbmmt.com/ehjjo/72453.html
http://m.sbmmt.com/ehjjo/2475474.html
http://m.sbmmt.com/ehjjo/121380.html
http://m.sbmmt.com/ehjjo/7264745.html
http://m.sbmmt.com/ehjjo/2928644.html
http://m.sbmmt.com/ehjjo/728765.html
http://m.sbmmt.com/ehjjo/558370.html
http://m.sbmmt.com/ehjjo/852679.html
http://m.sbmmt.com/ehjjo/450863.html
http://m.sbmmt.com/ehjjo/323074.html
http://m.sbmmt.com/ehjjo/169391.html
http://m.sbmmt.com/ehjjo/383110.html
http://m.sbmmt.com/ehjjo/68318.html
http://m.sbmmt.com/ehjjo/4789011.html
http://m.sbmmt.com/ehjjo/4234687.html
http://m.sbmmt.com/ehjjo/7832081.html
http://m.sbmmt.com/ehjjo/4153278.html
http://m.sbmmt.com/ehjjo/5928254.html
http://m.sbmmt.com/ehjjo/446158.html
http://m.sbmmt.com/ehjjo/9237493.html
http://m.sbmmt.com/ehjjo/9239002.html
http://m.sbmmt.com/ehjjo/3360997.html
http://m.sbmmt.com/ehjjo/33437.html