The principle is very simple, it is a hierarchical traversal of the tree, and the work is completed when the first leaf node is encountered during the traversal process.
The effect is as follows:
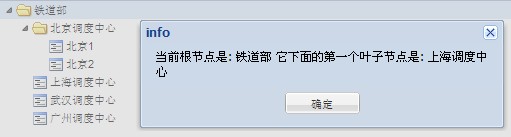
The code is as follows:
var currentRootNode = null; //The currently selected root node
function NodeClass()
{ //Define a node class
var nodeValue = null;
var nextNode = null ;//Next node
}
function InitQueue(queue)
{ //Initialize a queue
queue = new NodeClass();//The head node is empty
return queue;
}
function Empty(queue)
{ //Determine whether a queue is empty
var returnValue = false;
if(queue.nextNode == null)
{
returnValue = true;
}
return returnValue;
}
function EnQueue(queue,x)
{ //enqueue operation
var returnValue = queue;
var currentNode = queue;//Head node
while(currentNode.nextNode != null)
{//current until the last element is reached
currentNode = currentNode.nextNode; //
}
var tempNode = new NodeClass(); //Generate a new element with value X
tempNode.nodeValue = x;
currentNode.nextNode = tempNode; //Insert to the end
return returnValue; >}
function DeQueue(queue)
{ //Dequeue operation
var returnValue = null;
if(queue.nextNode != null)
{ //If the queue is not empty
if(queue.nextNode.nextNode == null)
{ //If it is the last element (even if the head of the queue is the tail of the queue, there is only one element)
returnValue = queue.nextNode.nodeValue; // Get the value of the element
queue.nextNode = null;//Set the nextNode of the queue of the head pointer to NULL
}
else
{
returnValue = queue.nextNode.nodeValue; / /Get the value of the element
queue.nextNode = queue.nextNode.nextNode; //Assign the pointer of the second element to the nextNode of the queue, which is equivalent to deleting the first element
}
}
return returnValue; //Return the value of the first deleted element
}
function GetHead(queue)
{ //Get the value of the queue head element
return queue.nextNode. nodeValue;
}
function Clear(queue)
{ //Clear a queue
queue.nextNode = null;
queue.nodeValue = null;
}
function Current_Size (queue)
{ //Get the size of the current queue
var returnValue = 0;
var currentNode = queue.nextNode; //Header node
while(currentNode != null)
{ //Calculate from beginning to end
returnValue;
currentNode = currentNode.nextNode; //Point to the next element
}
return returnValue; //Return size
}
function findFirstCheafNode ()
{
var childNodes = null;
var targetNode = null;//The target leaf node to be found
var queue = null;//Auxiliary queue
queue = InitQueue(queue );//Initialize the queue
queue = EnQueue(queue,currentRootNode);//Enter the root node into the queue
while (!Empty(queue))
{//As long as the queue is not empty
node = DeQueue(queue);//Dequeue
if (node.hasChildNodes())
{//Non-leaf node
childNodes = node.childNodes;
//Their child nodes are from left to right Enter the queue in sequence
for (var i = 0,len = childNodes.length; i < len ; i)
{
queue = EnQueue(queue,childNodes[i]);
}
}
else
{//Find the first leaf node
return node;
}
}
}
Ext.onReady(function()
{
var tree = new Ext.tree.TreePanel({
el: 'treeDiv',
useArrows: true,
autoScroll: true,
animate: true,
enableDD: true,
containerScroll: true,
border: false,
// auto create TreeLoader
loader: new Ext.tree.TreeLoader({dataUrl:'Level1.txt'})
} );
var rootID = '0';
var rootnode = new Ext.tree.AsyncTreeNode({
id : rootID,
text : 'Ministry of Railways',
draggable : false, // The root node is not allowed to be dragged
expanded : false
});
// Set the root node for the tree
tree.setRootNode(rootnode);
tree.render();
tree.on('click',function(node,event)
{//Query the first leaf node of the tree
currentRootNode = node;
var targetNode = findFirstCheafNode();
Ext.MessageBox.alert("info","The current root node is: " currentRootNode.text " and the first leaf node below it is: " targetNode.text);
});
}); var childNodes = null;
var targetNode = null;//The target leaf node to be found
var queue = null;//Auxiliary queue
queue = InitQueue(queue);//Initialization queue
queue = EnQueue(queue,currentRootNode);//Enter the root node into the queue
while (!Empty(queue))
{//As long as the queue is not empty
node = DeQueue(queue);//Dequeue
if (node.hasChildNodes())
{//Non-leaf nodes
childNodes = node.childNodes;
//Their child nodes are queued from left to right in sequence
for (var i = 0,len = childNodes.length; i < len ; i)
{
queue = EnQueue(queue,childNodes[i]);
}
}
else
{//Find the first leaf node
return node;
}
}
}
Ext.onReady(function()
{
var tree = new Ext. tree.TreePanel({
el: 'treeDiv',
useArrows: true,
autoScroll: true,
animate: true,
enableDD: true,
containerScroll: true,
border: false,
// auto create TreeLoader
loader: new Ext.tree.TreeLoader({dataUrl:'Level1.txt'})
});
var rootID = '0';
var rootnode = new Ext.tree.AsyncTreeNode({
id : rootID,
text : 'Ministry of Railways',
draggable : false, // The root node is not allowed to be dragged
expanded : false
});
// Set the root node for the tree
tree.setRootNode(rootnode);
tree.render();
tree.on('click',function(node,event)
{//Query the first leaf node of the tree
currentRootNode = node;
var targetNode = findFirstCheafNode();
alert("The current root node is: " currentRootNode.text " The first leaf node below it is: " targetNode.text);
});
});