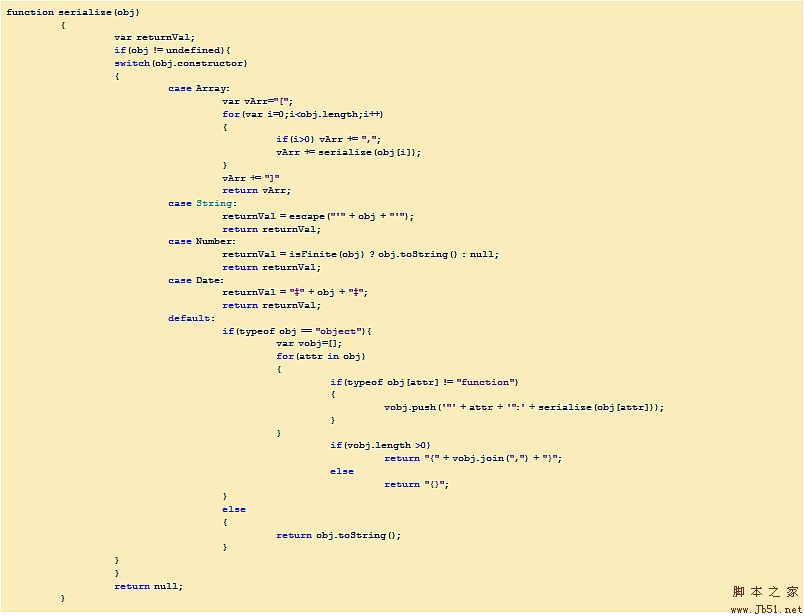
The author posted a picture, you can enlarge it to see it.
A few days ago I talked about the Literal Syntax issue of JavaScript. I thought it was quite interesting, so I studied it again. Can I convert objects into Literal form? Just like what we usually talk about serialization and deserialization. Of course it is possible, because JavaScript objects themselves provide a toString() method, which by default returns the literal form of a simple object.
What we need to do is to determine the specific type of the object, then Serialize each object separately, and then output it as the Literal syntax form of Object. To accurately determine the object type, just use the __typeof__ method I mentioned before. The code for serializing the object instance is as follows:
Object.prototype.Serialize = function()
{
var type = __typeof__(this);
switch(type)
{
case 'Array' :
{
var strArray = '[';
for ( var i=0 ; i < this.length ; i )
{
var value = '';
if ( this[i] )
{
value = this[i].Serialize();
}
strArray = value ',';
}
if ( strArray.charAt(strArray.length-1) == ',' )
{
strArray = strArray.substr(0, strArray.length-1);
}
strArray = ']';
return strArray;
}
case 'Date' :
{
return 'new Date(' this.getTime() ')';
}
case 'Boolean' :
case 'Function' :
case 'Number' :
case 'String' :
{
return this.toString();
}
default :
{
var serialize = '{';
for ( var key in this )
{
if ( key == 'Serialize' ) continue;
var subserialize = 'null';
if ( this[key] != undefined )
{
subserialize = this[key].Serialize();
}
serialize = 'rn' key ' : ' subserialize ',';
}
if ( serialize.charAt(serialize.length-1) == ',' )
{
serialize = serialize.substr(0, serialize .length-1);
}
serialize = 'rn}';
return serialize;
}
}
};
Actually Comparing the properties of Array and Object is troublesome, and the Serialize operation needs to be performed recursively. However, it should be noted that the Serialize method does not need to be serialized. The following is a test example, but this serialization method does not check circular references, and the objects that can be serialized are very limited.
var obj1 = [];
alert(obj1 .Serialize());
var obj2 = [1,[2,[3,[4,[5,[6,[7,[8,[9,[0]]]]]]] ]]]];
alert(obj2.Serialize());
var obj3 =
{
Properties1 : 1, Properties2 : '2', Properties3 : [3],
Method1 : function(){ return this.Properties1 this.Properties3[0];},
Method2 : function(){ return this.Preperties2; }
};
alert(obj3.Serialize( ));
var obj4 = [null, 1, 'string', true, function(){return 'keke';}, new Object()];
alert(obj4.Serialize() );
As for deserialization, it is very easy. Use eval to execute the above serialization result and you will get the class instance.