Core code:
function quickSort(arr){
//If the array has only one number, return it directly;
if(arr.length<1){
return arr;
}
//Find the index value of the middle number; if it is For floating point numbers, just round down
var centerIndex = Math.floor(arr.length/2);
//Find the value of this number based on the index value of the middle number;
var centerNum = arr.splice(centerIndex,1);
//Storage the number on the left
var arrLeft = [];
//Storage the number on the right
var arrRight = [];
for (i=0;i
if(arr[i]arrLeft.push(arr[i])
}else if(arr[i ]>centerNum){
arrRight.push(arr[i])
}
}
return quickSort(arrLeft).concat(centerNum,quickSort(arrRight));
};
var arrSort = [33,18,2,40,16,63,27];
var arr1 = quickSort(arrSort);
console.log(arr1);
The main principle is: the principle of quick sort: find the reference point, create two arrays to store separately, recurse
The reference point: find a number in the middle of the array;
Create two arrays and store them separately: using this reference point, store its left and right values into two defined new arrays respectively;
Recursion: call itself inside the function;
The point I summarize here is when using recursion:
1. There must be a judgment and return a value; otherwise it will be an infinite loop;
2. When calling yourself internally, the parameters passed are An internally defined variable, this variable is related to the parameters passed in for the first time;
3. To perform the same work, you can consider using recursion;
This is the first time the function is executed Variable situation: The middle number is 40; according to the judgment condition in the loop, if it is less than 40, it is stored in arrLeft, and if it is greater than 40, it is stored in arrRight. As shown below
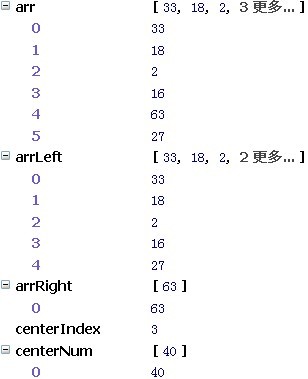
The second time the function
is called, when execution returns quickSort(arrLeft).concat(centerNum,quickSort(arrRight));
quickSort(arrLeft) The function will be called, and the parameters passed are [33,18,2,16,27]
The middle number is 2, the smaller number than 2 is placed on the left arrLeft, and the larger number than 2 is placed on the right arrRight
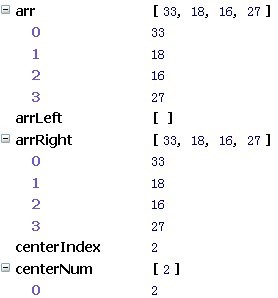
Finally call quickSort(arrRight)
Later, call yourself in a loop until the length of the parameter passed in is less than 1, then return the passed parameter parameters.