This article details best practices for writing effective PHPUnit unit tests in PHP 8. It emphasizes principles like independence, atomicity, and speed, advocating for leveraging PHP 8 features and avoiding common pitfalls such as over-mocking and
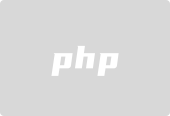
How Do I Write Effective Unit Tests for PHP 8 Code?
Effective unit tests for PHP 8 code follow the principles of good unit testing in general, but with a focus on leveraging PHP 8's features where appropriate. A good unit test should be:
-
Independent: Each test should be self-contained and not rely on the outcome of other tests. This ensures that failures are easily isolated. Use a setup method (
setUp
) in your PHPUnit test class to initialize necessary objects and resources for each test, and a teardown method (tearDown
) to clean up after each test.
-
Atomic: A single test should focus on verifying a single, specific aspect of your code's functionality. Avoid testing multiple things within one test; if a test fails, it should be immediately clear what part of the code is broken.
-
Repeatable: The test should produce the same result every time it's run, regardless of external factors. Avoid relying on external resources like databases or network connections unless absolutely necessary (and mocked in those cases).
-
Fast: Unit tests should execute quickly. Slow tests hinder the development process and discourage frequent testing.
-
Readable: Tests should be easy to understand and maintain. Use descriptive names for your tests and methods, and keep the test logic clear and concise. PHP 8's attributes can improve readability by reducing boilerplate.
Example: Let's say you have a function that adds two numbers:
<?php
function add(int $a, int $b): int {
return $a $b;
}
Copy after login
A PHPUnit test might look like this:
<?php
use PHPUnit\Framework\TestCase;
class AddTest extends TestCase {
public function testAddPositiveNumbers(): void {
$this->assertEquals(5, add(2, 3));
}
public function testAddNegativeNumbers(): void {
$this->assertEquals(-1, add(-2, 1));
}
public function testAddZero(): void {
$this->assertEquals(5, add(5, 0));
}
}
Copy after login
What are the best practices for writing PHPUnit tests in PHP 8?
Best practices for writing PHPUnit tests in PHP 8 build upon the principles of effective unit testing and leverage PHPUnit's features:
How can I improve the code coverage of my unit tests for PHP 8 applications?
Code coverage measures the percentage of your code that is executed by your unit tests. Improving code coverage requires a systematic approach:
-
Identify Untested Code: Use a code coverage tool (like PHPUnit's built-in code coverage reporting or a dedicated tool like Xdebug) to identify parts of your code that are not covered by tests.
-
Write Tests for Untested Code: Focus on writing tests for the uncovered code sections. Prioritize testing critical paths and complex logic first.
-
Refactor for Testability: If parts of your code are difficult to test (e.g., due to tight coupling or excessive dependencies), refactor your code to make it more testable. This often involves using dependency injection and separating concerns.
-
Increase Test Granularity: Break down large functions into smaller, more manageable units that are easier to test individually.
-
Don't Obsess Over 100% Coverage: While striving for high code coverage is beneficial, don't aim for 100% coverage at all costs. Focus on testing the most critical parts of your application and avoid writing trivial tests that don't add value. 100% coverage doesn't guarantee bug-free code; focus on testing the critical functionality and edge cases.
What are some common pitfalls to avoid when unit testing PHP 8 code?
Several common pitfalls can hinder effective unit testing:
-
Testing Implementation Details: Focus on testing the public interface of your classes and functions, not their internal implementation details. Changes to the internal implementation should not break your tests unless the public behavior changes.
-
Ignoring Edge Cases: Pay attention to edge cases and boundary conditions (e.g., empty inputs, null values, extreme values). These are often where bugs hide.
-
Over-reliance on Mocking: While mocking is essential for testing interactions with external dependencies, over-reliance on mocking can lead to brittle tests that don't accurately reflect the real-world behavior of your code.
-
Neglecting Error Handling: Test how your code handles errors and exceptions. Ensure that your tests cover both successful and unsuccessful scenarios.
-
Writing Slow Tests: Slow tests discourage frequent testing. Keep your tests concise and efficient to avoid slowing down the development process.
-
Ignoring Test Maintainability: Write clean, readable, and maintainable tests. Tests should be easy to understand and update as your code evolves. Poorly written tests become a burden over time. Use descriptive names and comments where necessary.
The above is the detailed content of How Do I Write Effective Unit Tests for PHP 8 Code?. For more information, please follow other related articles on the PHP Chinese website!