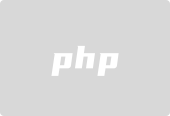
Accessing Twitter Data with API v1.1 and OAuth
Overview:
This guide details how to access Twitter data using the updated API v1.1 and OAuth authentication. Since Twitter API v1 is obsolete, this method is essential for modern Twitter development. We'll use HttpWebRequest
objects for demonstration.
OAuth Authentication:
-
Obtain API Keys: Register your application on the Twitter developer portal (//m.sbmmt.com/link/30fad467b7363d55fa24b3398fdef557) to receive your consumer key and secret.
-
Create Authentication Header: Encode your consumer key and secret using Base64 encoding and combine them with the "Basic" authorization scheme.
-
Send Authentication Request: Issue a POST request to Twitter's OAuth token endpoint (//m.sbmmt.com/link/f055c54d16a8cc75a8cc996511cc9a9c), supplying your client credentials and encoded keys.
-
Process Authentication Response: Parse the JSON response to retrieve the access token and token type.
Fetching User Timeline:
-
Construct Timeline URL: Create the URL for the user timeline request, specifying the screen name, desired tweet count, inclusion of retweets, and exclusion of replies.
-
Create Timeline Request: Formulate a GET request and include the access token in the authorization header, formatted according to the retrieved token type.
-
Parse Timeline Response: Process the JSON response containing the user's timeline data and convert it into a usable data structure.
Code Example Snippet:
// Replace with your actual keys and screen name
string oAuthConsumerKey = "yourConsumerKey";
string oAuthConsumerSecret = "yourConsumerSecret";
string oAuthUrl = "//m.sbmmt.com/link/f055c54d16a8cc75a8cc996511cc9a9c";
// ...
// Construct Authentication Header
string authHeaderFormat = "Basic {0}";
// ...
// Construct Timeline URL
string timelineFormat = "https://api.twitter.com/1.1/statuses/user_timeline.json?screen_name={0}&include_rts=1&exclude_replies=1&count=5";
// ...
public class TwitAuthenticateResponse
{
public string token_type { get; set; }
public string access_token { get; set; }
}
Copy after login
Important Considerations:
- Timeline responses are JSON-formatted.
- Access tokens have a limited lifespan and will need to be refreshed periodically.
- See the associated GitHub project for complete ASP.NET web app and MVC app examples.
- JSON parsing techniques may vary based on your chosen development environment.
The above is the detailed content of How to Authenticate with OAuth and Request a User's Timeline Using the Twitter API v1.1?. For more information, please follow other related articles on the PHP Chinese website!