


How to Authenticate and Retrieve a Twitter User's Timeline Using the v1.1 API?
Accessing Twitter Data via API v1.1: Authentication and Timeline Retrieval
Due to the deprecation of Twitter's REST API v1, developers must now utilize the v1.1 API for accessing Twitter data. This guide provides a step-by-step walkthrough of authenticating and retrieving a user's timeline using direct HTTP requests, eliminating the need for third-party libraries.
Authentication Process
- Obtain oAuth Credentials: Secure your oAuth Consumer Key and Secret from the Twitter Developer Portal.
-
Construct Authorization Header:
- Concatenate your Consumer Key and Secret.
- Encode the combined string using Base64 encoding.
- Format the authorization header as: "Basic {Base64EncodedString}".
-
Submit Authentication Request:
- Send a POST request to Twitter's authentication endpoint ("//m.sbmmt.com/link/f055c54d16a8cc75a8cc996511cc9a9c").
- Include the constructed authorization header.
- The POST body should specify the grant type.
- Parse the JSON response to obtain your authentication response object.
Retrieving the User Timeline
-
Create Timeline URL:
- Construct the URL using the target user's screen name and any desired parameters (e.g., the number of tweets to retrieve).
-
Generate Timeline Authorization Header:
- Utilize the access token received during the authentication process to build the authorization header for this request.
-
Send Timeline Request:
- Submit a GET request to Twitter's timeline endpoint, incorporating the authorization header.
-
Process Timeline JSON:
- Read the response as a string.
- Parse the JSON data into a suitable data structure within your application.
Illustrative C# Code Snippet
The following C# code example illustrates the implementation:
// Your oAuth consumer key and secret string oAuthConsumerKey = "superSecretKey"; string oAuthConsumerSecret = "superSecretSecret"; // Twitter's authentication endpoint string oAuthUrl = "//m.sbmmt.com/link/f055c54d16a8cc75a8cc996511cc9a9c"; // Target user's screen name string screenname = "aScreenName"; // Construct authorization header string authHeaderFormat = "Basic {0}"; string authHeader = string.Format(authHeaderFormat, ...); // Base64 encoding omitted for brevity // Send authentication request var authRequest = (HttpWebRequest)WebRequest.Create(oAuthUrl); authRequest.Headers.Add("Authorization", authHeader); // ... (rest of authentication request handling) // Parse authentication response TwitAuthenticateResponse twitAuthResponse = ...; // Construct timeline URL string timelineFormat = "https://api.twitter.com/1.1/statuses/user_timeline.json?screen_name={0}&...;"; string timelineUrl = string.Format(timelineFormat, screenname); // Send timeline request var timeLineRequest = (HttpWebRequest)WebRequest.Create(timelineUrl); timeLineRequest.Headers.Add("Authorization", ...); // Authorization using access token // ... (rest of timeline request handling) // Retrieve and process timeline JSON string timeLineJson = ...;
This example showcases the core steps using raw HTTP requests, granting you fine-grained control over your interaction with the Twitter API. Remember to replace placeholder values with your actual credentials and handle potential errors appropriately.
The above is the detailed content of How to Authenticate and Retrieve a Twitter User's Timeline Using the v1.1 API?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undress AI Tool
Undress images for free

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
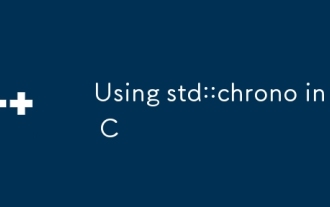
std::chrono is used in C to process time, including obtaining the current time, measuring execution time, operation time point and duration, and formatting analysis time. 1. Use std::chrono::system_clock::now() to obtain the current time, which can be converted into a readable string, but the system clock may not be monotonous; 2. Use std::chrono::steady_clock to measure the execution time to ensure monotony, and convert it into milliseconds, seconds and other units through duration_cast; 3. Time point (time_point) and duration (duration) can be interoperable, but attention should be paid to unit compatibility and clock epoch (epoch)
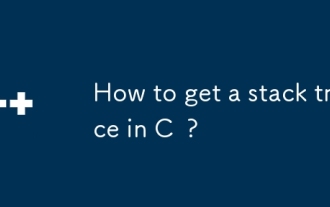
There are mainly the following methods to obtain stack traces in C: 1. Use backtrace and backtrace_symbols functions on Linux platform. By including obtaining the call stack and printing symbol information, the -rdynamic parameter needs to be added when compiling; 2. Use CaptureStackBackTrace function on Windows platform, and you need to link DbgHelp.lib and rely on PDB file to parse the function name; 3. Use third-party libraries such as GoogleBreakpad or Boost.Stacktrace to cross-platform and simplify stack capture operations; 4. In exception handling, combine the above methods to automatically output stack information in catch blocks
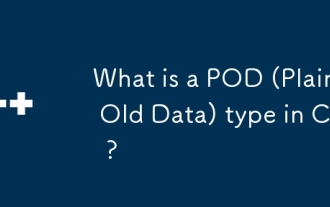
In C, the POD (PlainOldData) type refers to a type with a simple structure and compatible with C language data processing. It needs to meet two conditions: it has ordinary copy semantics, which can be copied by memcpy; it has a standard layout and the memory structure is predictable. Specific requirements include: all non-static members are public, no user-defined constructors or destructors, no virtual functions or base classes, and all non-static members themselves are PODs. For example structPoint{intx;inty;} is POD. Its uses include binary I/O, C interoperability, performance optimization, etc. You can check whether the type is POD through std::is_pod, but it is recommended to use std::is_trivia after C 11.
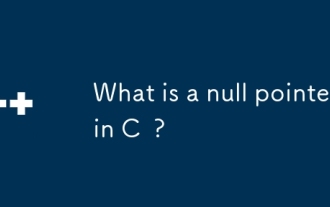
AnullpointerinC isaspecialvalueindicatingthatapointerdoesnotpointtoanyvalidmemorylocation,anditisusedtosafelymanageandcheckpointersbeforedereferencing.1.BeforeC 11,0orNULLwasused,butnownullptrispreferredforclarityandtypesafety.2.Usingnullpointershe
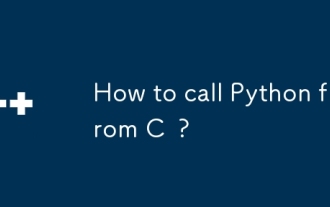
To call Python code in C, you must first initialize the interpreter, and then you can achieve interaction by executing strings, files, or calling specific functions. 1. Initialize the interpreter with Py_Initialize() and close it with Py_Finalize(); 2. Execute string code or PyRun_SimpleFile with PyRun_SimpleFile; 3. Import modules through PyImport_ImportModule, get the function through PyObject_GetAttrString, construct parameters of Py_BuildValue, call the function and process return
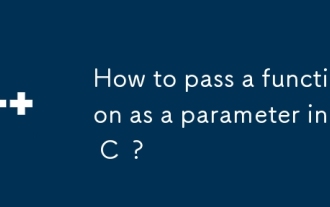
In C, there are three main ways to pass functions as parameters: using function pointers, std::function and Lambda expressions, and template generics. 1. Function pointers are the most basic method, suitable for simple scenarios or C interface compatible, but poor readability; 2. Std::function combined with Lambda expressions is a recommended method in modern C, supporting a variety of callable objects and being type-safe; 3. Template generic methods are the most flexible, suitable for library code or general logic, but may increase the compilation time and code volume. Lambdas that capture the context must be passed through std::function or template and cannot be converted directly into function pointers.
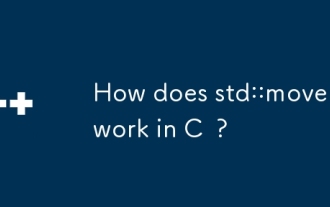
std::move does not actually move anything, it just converts the object to an rvalue reference, telling the compiler that the object can be used for a move operation. For example, when string assignment, if the class supports moving semantics, the target object can take over the source object resource without copying. Should be used in scenarios where resources need to be transferred and performance-sensitive, such as returning local objects, inserting containers, or exchanging ownership. However, it should not be abused, because it will degenerate into a copy without a moving structure, and the original object status is not specified after the movement. Appropriate use when passing or returning an object can avoid unnecessary copies, but if the function returns a local variable, RVO optimization may already occur, adding std::move may affect the optimization. Prone to errors include misuse on objects that still need to be used, unnecessary movements, and non-movable types
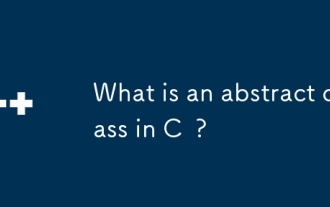
The key to an abstract class is that it contains at least one pure virtual function. When a pure virtual function is declared in the class (such as virtualvoiddoSomething()=0;), the class becomes an abstract class and cannot directly instantiate the object, but polymorphism can be realized through pointers or references; if the derived class does not implement all pure virtual functions, it will also remain an abstract class. Abstract classes are often used to define interfaces or shared behaviors, such as designing Shape classes in drawing applications and implementing the draw() method by derived classes such as Circle and Rectangle. Scenarios using abstract classes include: designing base classes that should not be instantiated directly, forcing multiple related classes to follow a unified interface, providing default behavior, and requiring subclasses to supplement details. In addition, C
