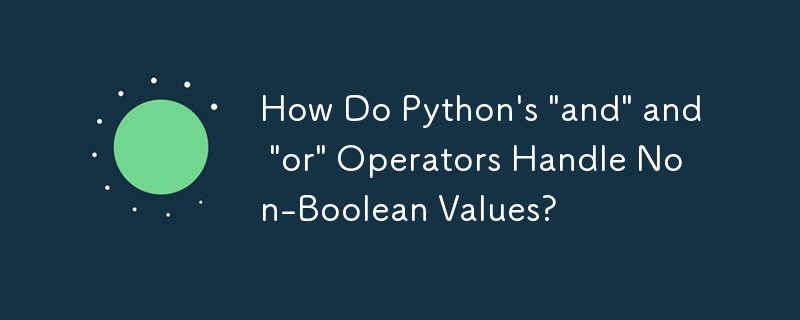
How Non-Boolean Values Interact with "and" and "or" in Python
Question:
In Python, it's observed that logical operators "and" and "or" exhibit unusual behavior when operating on non-boolean values. For instance, the expression "10 and 7-2" returns 5 instead of True or False. Explain this behavior and its implications in Python programming.
Answer:
Python's logical operators "and" and "or" have unique rules for handling non-boolean values:
"and" Behavior:
- If any value in the expression is False, "False" is returned.
- If no values are False, the last value in the expression is returned.
"or" Behavior:
- If any value in the expression is True, "True" is returned.
- If no values are True, the last value in the expression is returned.
Consider the example expression "10 and 7-2":
- 7-2 evaluates to 5 (a non-boolean value).
- There are no False values in the expression, so "and" returns the last value, which is 5.
Similarly, for "10 or 7-2":
- 7-2 evaluates to 5 (a non-boolean value).
- There is a True value in the expression (10), so "or" returns 10.
Legitimacy and Gotchas:
This behavior is legitimate and commonly used. However, it can lead to unexpected results if not properly understood. Gotchas to watch out for include:
-
Implicit Truthy/Falsy Values: Some non-boolean values, such as empty strings, empty lists, and None, are implicitly treated as False, while non-zero numbers are implicitly True.
-
Operand Ordering: The order of operands matters with "and" and "or". In the example above, "10 and 7-2" returns 5, but "7-2 and 10" returns 8 (since the implicit False value of 5 short-circuits "and").
-
Type Conversion: "and" and "or" will attempt to convert non-boolean operands to boolean values. This can lead to unexpected results in cases like "0 and '0' == True" (since the string '0' is implicitly treated as False).
The above is the detailed content of How Do Python's 'and' and 'or' Operators Handle Non-Boolean Values?. For more information, please follow other related articles on the PHP Chinese website!