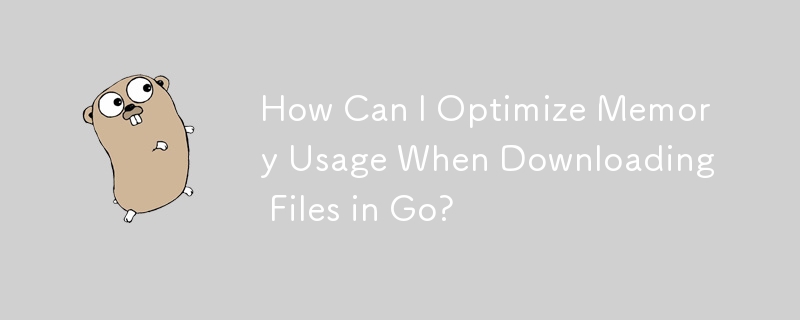
Optimizing Memory Utilization for File Downloading
In the provided code, the function getURL reads the entire content of a file into memory before processing. While this approach is straightforward, it can result in excessive memory consumption.
Alternatives to Allocating Large Memory Blocks
Instead of allocating a large memory block for the entire file, consider the following strategies to optimize memory usage:
-
Limit Request Size: Restrict the files eligible for download to a specific maximum size. This prevents excessive memory consumption for large files.
-
Buffer Pooling: Maintain a buffer pool to allocate and reuse small memory blocks, avoiding the need to allocate and deallocate large arrays/slices frequently.
-
Stream Processing: Process the file using an io.Reader interface instead of reading the entire content into memory. This enables processing of the file without the need to hold the entire file in memory.
Additional Considerations
-
Garbage Collection: Use runtime.GC() to explicitly trigger garbage collection.
-
FreeOSMemory: Use debug.FreeOSMemory() to request the Go runtime to release unused memory to the operating system. However, these measures are temporary and cannot fully compensate for inefficient memory allocation.
Best Practices
- Design your processing units to operate on io.Readers instead of allocating large byte slices.
- Establish memory/buffer pools to optimize allocation and deallocation.
- Consider limiting the size of eligible files to minimize memory consumption.
The above is the detailed content of How Can I Optimize Memory Usage When Downloading Files in Go?. For more information, please follow other related articles on the PHP Chinese website!