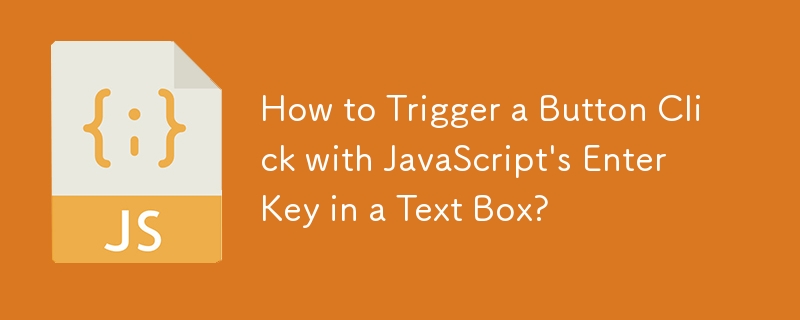
Trigger Button Click with JavaScript on Enter Key in Text Box
JavaScript offers a convenient solution to trigger a button click event upon pressing the Enter key within a specific text box. Let's delve into the solution:
jQuery Implementation:
Within the jQuery framework, the following code snippet achieves the desired behavior:
$("#id_of_textbox").keyup(function(event) {
if (event.keyCode === 13) {
$("#id_of_button").click();
}
});
Copy after login
Here's how it works:
- The $("#id_of_textbox") selector targets the text box with the specified ID.
- The keyup event listener monitors when the Enter key is released within the text box.
- Inside the event handler, the event.keyCode property checks if the pressed key's code matches that of the Enter key (13).
- If the Enter key was pressed, the $("#id_of_button").click() statement simulates a click event on the specified button.
Native JavaScript Implementation:
For pure JavaScript, the following code can be used:
const textbox = document.getElementById("id_of_textbox");
const button = document.getElementById("id_of_button");
textbox.addEventListener("keypress", (event) => {
if (event.key === "Enter") {
button.click();
}
});
Copy after login
This code:
- References the text box and button elements using their IDs.
- Attaches a keypress event listener to the text box.
- Within the event handler, it checks if the pressed key is the Enter key.
- If the Enter key was pressed, the button.click() method simulates a click on the button.
Implementation Considerations:
-
Specifying IDs: Ensure that the text box and button elements have unique IDs for the code to work correctly.
-
Avoiding Form Submit: If the Enter key is used to submit a form, it may conflict with the button's click event. To prevent this, avoid using the Enter key for form submission if possible.
-
Compatibility: The keypress solution is considered more modern than keyup, but keyup may be necessary for older browsers.
The above is the detailed content of How to Trigger a Button Click with JavaScript's Enter Key in a Text Box?. For more information, please follow other related articles on the PHP Chinese website!