C#으로 구현된 24점 게임 계산 알고리즘
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.IO; namespace Calc24Points { public class Cell { public enum Type { Number, Signal } public int Number; public char Signal; public Type Typ; public Cell Right; public Cell Left; /// <summary> /// 符号优先级 /// </summary> public int Priority { get { if (Typ == Type.Signal) { switch (Signal) { case '+': return 0; case '-': return 0; case '*': return 1; case '/': return 1; default: return -1; } } return -1; } } /// <summary> /// 基本单元构造函数 /// </summary> /// <param name="t">单元类型,数值或符号</param> /// <param name="num">数值</param> /// <param name="sig">符号</param> public Cell(Type t, int num, char sig) { Right = null; Left = null; Typ = t; Number = num; Signal = sig; } public Cell() { Right = null; Left = null; Number = 0; Typ = Type.Number; } public Cell(Cell c) { Right = null; Left = null; Number = c.Number; Signal = c.Signal; Typ = c.Typ; } } public class Calc24Points { string m_exp; bool m_stop; Cell[] m_cell; int[] m_express; StringWriter m_string; public Calc24Points(int n1, int n2, int n3, int n4) { m_cell = new Cell[8]; m_cell[0] = new Cell(Cell.Type.Number, n1, '?'); m_cell[1] = new Cell(Cell.Type.Number, n2, '?'); m_cell[2] = new Cell(Cell.Type.Number, n3, '?'); m_cell[3] = new Cell(Cell.Type.Number, n4, '?'); m_cell[4] = new Cell(Cell.Type.Signal, 0, '+'); m_cell[5] = new Cell(Cell.Type.Signal, 0, '-'); m_cell[6] = new Cell(Cell.Type.Signal, 0, '*'); m_cell[7] = new Cell(Cell.Type.Signal, 0, '/'); m_stop = false; m_express = new int[7]; m_string = new StringWriter(); m_exp = null; } public override string ToString() { if (m_exp == null) { PutCell(0); m_exp = m_string.ToString(); } if (m_exp != "") return m_exp; return null; } /// <summary> /// 在第n位置放置一个单元 /// </summary> /// <param name="n"></param> void PutCell(int n) { if (n >= 7) { if (Calculate()) { m_stop = true; Formate(); } return; } int end = 8; if (n < 2) end = 4; for (int i = 0; i < end; ++i) { m_express[n] = i; if (CheckCell(n)) PutCell(n + 1); if (m_stop) break; } } /// <summary> /// 检查当前放置是否合理 /// </summary> /// <param name="n"></param> /// <returns></returns> bool CheckCell(int n) { int nums = 0, sigs = 0; for (int i = 0; i <= n; ++i) { if (m_cell[m_express[i]].Typ == Cell.Type.Number) ++nums; else ++sigs; } if (nums - sigs < 1) return false; if (m_cell[m_express[n]].Typ == Cell.Type.Number) //数值不能重复,但是符号可以重复 { for (int i = 0; i < n; ++i) if (m_express[i] == m_express[n]) return false; } if (n == 6) { if (nums != 4 || sigs != 3) return false; if (m_cell[m_express[6]].Typ != Cell.Type.Signal) return false; return true; } return true; } /// <summary> /// 计算表达式是否为24 /// </summary> /// <returns>返回值true为24,否则不为24</returns> bool Calculate() { double[] dblStack = new double[4]; int indexStack = -1; for (int i = 0; i < 7; ++i) { if (m_cell[m_express[i]].Typ == Cell.Type.Number) { ++indexStack; dblStack[indexStack] = m_cell[m_express[i]].Number; } else { switch (m_cell[m_express[i]].Signal) { case '+': dblStack[indexStack - 1] = dblStack[indexStack - 1] + dblStack[indexStack]; break; case '-': dblStack[indexStack - 1] = dblStack[indexStack - 1]-+ dblStack[indexStack]; break; case '*': dblStack[indexStack - 1] = dblStack[indexStack - 1] * dblStack[indexStack]; break; case '/': dblStack[indexStack - 1] = dblStack[indexStack - 1] / dblStack[indexStack]; break; } --indexStack; } } if (Math.Abs(dblStack[indexStack] - 24) < 0.1) return true; return false; } /// <summary> /// 后缀表达式到中缀表达式 /// </summary> void Formate() { Cell[] c = new Cell[7]; for (int i = 0; i < 7; ++i) c[i] = new Cell(m_cell[m_express[i]]); int[] cStack = new int[4]; int indexStack = -1; for (int i = 0; i < 7; ++i) { if (c[i].Typ == Cell.Type.Number) { ++indexStack; cStack[indexStack] = i; } else { c[i].Right = c[cStack[indexStack]]; --indexStack; c[i].Left = c[cStack[indexStack]]; cStack[indexStack] = i; } } ToStringFormate(c[cStack[indexStack]]); } void ToStringFormate(Cell root) { if (root.Left.Typ == Cell.Type.Number) { m_string.Write(root.Left.Number); m_string.Write(root.Signal); } else { if (root.Priority > root.Left.Priority) { m_string.Write("("); ToStringFormate(root.Left); m_string.Write(")"); } else ToStringFormate(root.Left); m_string.Write(root.Signal); } if (root.Right.Typ == Cell.Type.Number) m_string.Write(root.Right.Number); else { if (root.Priority >= root.Right.Priority) { m_string.Write("("); ToStringFormate(root.Right); m_string.Write(")"); } else ToStringFormate(root.Right); } } } }

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










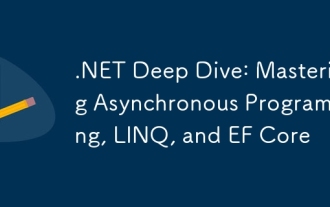
.NET 비동기 프로그래밍, LINQ 및 EFCORE의 핵심 개념은 다음과 같습니다. 1. 비동기 프로그래밍은 비동기 및 대기하는 응용 프로그램 응답 성을 향상시킵니다. 2. LINQ는 Unified Syntax를 통해 데이터 쿼리를 단순화합니다. 3. Efcore는 ORM을 통해 데이터베이스 작업을 단순화합니다.
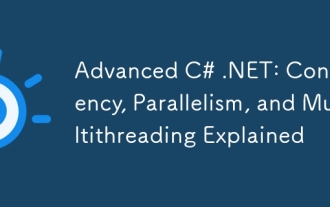
C#.NET은 동시, 병렬 및 멀티 스레드 프로그래밍을위한 강력한 도구를 제공합니다. 1) 스레드 클래스를 사용하여 스레드를 생성하고 관리합니다. 2) 작업 클래스는 스레드 풀을 사용하여 자원 활용을 개선하기 위해 나사산을 통해 병렬 컴퓨팅을 구현합니다. 4) ASYNC/AWAIT 및 작업을 통해 병렬 컴퓨팅을 구현합니다. ASYNC/AWAIT 및 TASK. ANDAL에서 데이터를 얻고 프로세스하는 데 사용되면 5) 스레드 풀을 사용하지 않으면 스레드 풀을 사용하고 성능을 발휘합니다.
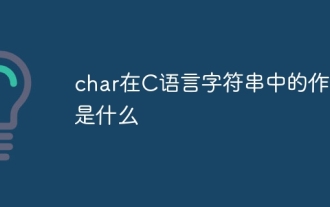
C에서 숯 유형은 문자열에 사용됩니다. 1. 단일 문자를 저장하십시오. 2. 배열을 사용하여 문자열을 나타내고 널 터미네이터로 끝납니다. 3. 문자열 작동 함수를 통해 작동합니다. 4. 키보드에서 문자열을 읽거나 출력하십시오.
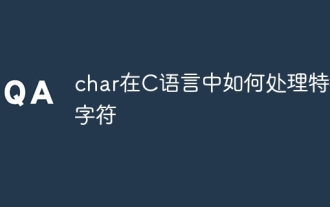
C 언어에서 특수 문자는 다음과 같은 탈출 시퀀스를 통해 처리됩니다. \ n 라인 브레이크를 나타냅니다. \ t는 탭 문자를 의미합니다. char c = '\ n'과 같은 특수 문자를 나타 내기 위해 탈출 시퀀스 또는 문자 상수를 사용하십시오. 백 슬래시는 두 번 탈출해야합니다. 다른 플랫폼과 컴파일러마다 다른 탈출 시퀀스가있을 수 있습니다. 문서를 참조하십시오.
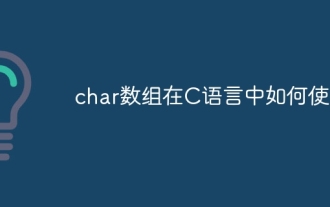
char 어레이는 문자 시퀀스를 C 언어로 저장하고 char array_name [size]로 선언됩니다. 액세스 요소는 첨자 연산자를 통해 전달되며 요소는 문자열의 끝점을 나타내는 널 터미네이터 '\ 0'으로 끝납니다. C 언어는 strlen (), strcpy (), strcat () 및 strcmp ()와 같은 다양한 문자열 조작 함수를 제공합니다.
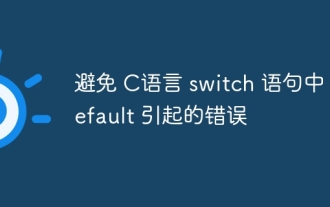
C 스위치 문에서 기본적으로 발생하는 오류를 피하기위한 전략 : 상수 대신 열거를 사용하여 사례 문의 값을 열거의 유효한 멤버로 제한합니다. 마지막 사례 명령문에서 러프를 사용하여 프로그램이 다음 코드를 계속 실행할 수 있도록하십시오. 스위치가없는 스위치 문의 경우 항상 오류 처리에 대한 기본 문을 추가하거나 기본 동작을 제공하십시오.
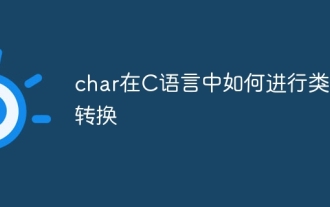
C 언어에서 숯 유형 변환은 다른 유형으로 직접 변환 할 수 있습니다. 캐스팅 : 캐스팅 캐릭터 사용. 자동 유형 변환 : 한 유형의 데이터가 다른 유형의 값을 수용 할 수 있으면 컴파일러가 자동으로 변환됩니다.
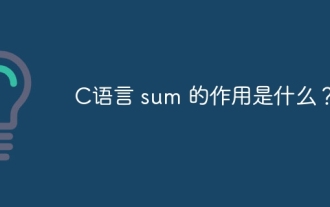
C 언어에는 내장 합계 기능이 없으므로 직접 작성해야합니다. 합계는 배열 및 축적 요소를 가로 질러 달성 할 수 있습니다. 루프 버전 : 루프 및 배열 길이를 사용하여 계산됩니다. 포인터 버전 : 포인터를 사용하여 배열 요소를 가리키며 효율적인 합계는 자체 증가 포인터를 통해 달성됩니다. 동적으로 배열 버전을 할당 : 배열을 동적으로 할당하고 메모리를 직접 관리하여 메모리 누출을 방지하기 위해 할당 된 메모리가 해제되도록합니다.
