요소를 비연속적으로 저장하는 선형 데이터 구조를 LinkedList라고 하며, 포인터를 사용하여 연결된 목록의 요소를 서로 연결하고 System.Collections.Generic 네임스페이스는 LinkedList< T> 요소를 제거하거나 매우 빠른 방식으로 삽입할 수 있는 C#의 클래스로 클래식 연결 목록을 구현하고 각 개체의 할당이 연결 목록에서 분리되어 있으며 특정 작업을 수행하기 위해 전체 컬렉션을 복사할 필요가 없습니다. 링크드 리스트에 있습니다.
구문:
C#의 LinkedList 클래스 구문은 다음과 같습니다.
LinkedList<Type> linkedlist_name = new LinkedList <Type>();
로그인 후 복사
여기서 Type은 연결 목록의 유형을 나타냅니다.
C#에서 LinkedList 클래스 작업
- 연결된 목록에는 노드가 있으며 모든 노드는 데이터 필드와 연결 목록에서 다음 노드에 대한 링크라는 두 부분으로 구성됩니다.
- 연결된 목록의 모든 노드 유형은 LinkedListNode 입력하세요.
- 연결된 목록에서 노드를 제거하고 동일한 연결 목록에 다시 삽입하거나 다른 연결 목록에 Cab을 삽입할 수 있으므로 힙에 추가 할당이 없습니다.
- 연결된 목록에 요소를 삽입하는 것, 연결 목록에서 요소를 제거하는 것, 좋아요 목록이 유지하는 내부 속성인 count의 속성을 얻는 것은 모두 O(1) 작업입니다.
- 열거자는 범용 연결 목록이므로 연결 목록 클래스에서 지원됩니다.
- 연결된 목록을 일관성 없게 만드는 것은 연결 목록에서 지원되지 않습니다.
- 연결된 목록이 이중 연결 목록인 경우 각 노드에는 두 개의 포인터가 있습니다. 하나는 목록의 이전 노드를 가리키고 다른 하나는 목록의 다음 노드를 가리킵니다.
LinkedList 클래스 생성자
C#의 LinkedList 클래스에는 여러 생성자가 있습니다. 그들은:
-
LinkedList(): 연결된 목록 클래스의 새 인스턴스가 비어 있는 초기화됩니다.
-
LinkedList(IEnumerable): 복사된 모든 요소를 누적할 만큼 용량이 충분한 IEnumerable의 지정된 구현에서 가져온 연결 목록 클래스의 새 인스턴스가 초기화됩니다.
-
LinkedList(SerializationInfo, StreamingContext): 연결된 목록 클래스의 새 인스턴스가 초기화되며 매개변수로 지정된 serializationInfo 및 StreamingContext를 사용하여 직렬화될 수 있습니다.
C#의 LinkedList 클래스 메서드
C#의 LinkedList 클래스에는 여러 가지 메서드가 있습니다. 그들은:
-
AddAfter: A value or new node is added after an already present node in the linked list using the AddAfter method.
-
AddFirst: A value or new node is added at the beginning of the linked list using the AddFirst method.
-
AddBefore: A value or new node is added before an already present node in the linked list using the AddBefore method.
-
AddLast: A value or new node is added at the end of the linked list using the AddLast method.
-
Remove(LinkedListNode): A node specified as a parameter will be removed from the linked list using Remove(LinkedListNode) method.
-
RemoveFirst(): A node at the beginning of the linked list will be removed from the linked list using RemoveFirst() method.
-
Remove(T): The first occurrence of the value specified as a parameter in the linked list will be removed from the linked list using the Remove(T) method.
-
RemoveLast(): A node at the end of the linked list will be removed from the linked list using the RemoveLast() method.
-
Clear(): All the nodes from the linked list will be removed using the Clear() method.
-
Find(T): The value specified as the parameter present in the very first node will be identified by using the Find(T) method.
-
Contains(T): We can use the Contains(T) method to find out if a value is present in the linked list or not.
-
ToString(): A string representing the current object is returned by using the ToString() method.
-
CopyTo(T[], Int32): The whole linked list is copied to an array which is one dimensional and is compatible with the linked list and the linked list begins at the index specified in the array to be copied to using CopyTo(T[], Int32) method.
-
OnDeserialization(Object): After the completion of deserialization, an event of deserialization is raised and the ISerializable interface is implemented using OnDeserialization(Object) method.
-
Equals(Object): If the object specified as the parameter is equal to the current object or not is identified using Equals(Object) method.
-
FindLast(T): The value specified as the parameter present in the last node will be identified by using FindLast(T) method.
-
MemberwiseClone(): A shallow copy of the current object is created using MemeberwiseClone() method.
-
GetEnumerator(): An enumerator is returned using GetEnumerator() method and the returned enumerator loops through the linked list.
-
GetType(): The type of the current instance is returned using GetType() method.
-
GetHashCode(): The GetHashCode() method is the hash function by default.
-
GetObjectData(SerializationInfo, StreamingContext): The data which is necessary to make the linked list serializable is returned by using GetObjectData(SerializationInfo, StreamingContext) method along with implementing the ISerializable interface.
Example of LinkedList Class in C#
C# program to demonstrate AddLast() method, Remove(LinkedListNode) method, Remove(T) method, RemoveFirst() method, RemoveLast() method and Clear() method in Linked List class:
Code:
using System;
using System.Collections.Generic;
//a class called program is defined
public class program
{
// Main Method is called
static public void Main()
{
//a new linked list is created
LinkedList<String> list = new LinkedList<String>();
//AddLast() method is used to add the elements to the newly created linked list
list.AddLast("Karnataka");
list.AddLast("Mumbai");
list.AddLast("Pune");
list.AddLast("Hyderabad");
list.AddLast("Chennai");
list.AddLast("Delhi");
Console.WriteLine("The states in India are:");
//Using foreach loop to display the elements of the newly created linked list
foreach(string places in list)
{
Console.WriteLine(places);
}
Console.WriteLine("The places after using Remove(LinkedListNode) method are:");
//using Remove(LinkedListNode) method to remove a node from the linked list
list.Remove(list.First);
foreach(string place in list)
{
Console.WriteLine(place);
}
Console.WriteLine("The places after using Remove(T) method are:");
//using Remove(T) method to remove a node from the linked list
list.Remove("Chennai");
foreach(string plac in list)
{
Console.WriteLine(plac);
}
Console.WriteLine("The places after using RemoveFirst() method are:");
//using RemoveFirst() method to remove the first node from the linked list
list.RemoveFirst();
foreach(string pla in list)
{
Console.WriteLine(pla);
}
Console.WriteLine("The places after using RemoveLast() method are:");
//using RemoveLast() method to remove the last node from the linked list
list.RemoveLast();
foreach(string pl in list)
{
Console.WriteLine(pl);
}
//using Clear() method to remove all the nodes from the linked list
list.Clear();
Console.WriteLine("The count of places after using Clear() method is: {0}",
list.Count);
}
}
로그인 후 복사
The output of the above program is as shown in the snapshot below:
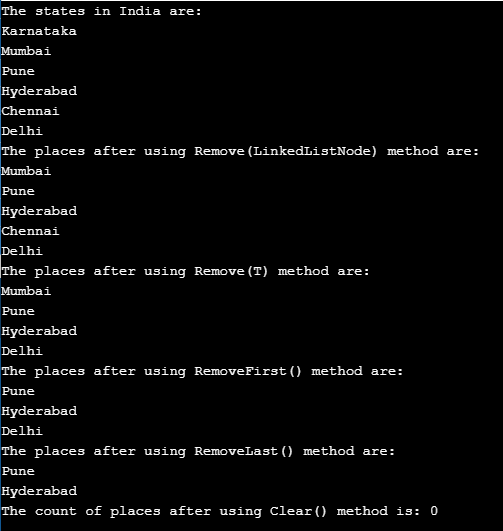
In the above program, a class called program is defined. Then the main method is called. Then a new linked list is created. Then AddLast() method is used to add the elements to the newly created linked list. Then foreach loop is used to display the elements of the newly created linked list. Then Remove(LinkedListNode) method is used to remove a node from the linked list. Then Remove(T) method is used to remove a node from the linked list. Then RemoveFirst() method is used to remove the first node from the linked list. Then RemoveLast() method is used to remove the last node from the linked list. Then Clear() method is used to remove all the nodes from the linked list. The output of the program is shown in the snapshot above.
위 내용은 C#링크드리스트의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!