
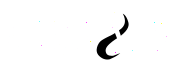
PHP 8.3 は、PHP 言語のメジャー アップデートです。
これには、クラス定数の明示的な型指定、読み取り専用プロパティのディープクローン作成、ランダム性機能の追加など、多くの新機能が含まれています。いつものように、パフォーマンスの向上、バグ修正、一般的なクリーンアップも含まれています。
今すぐ PHP 8.3 にアップグレードしてください。型付きクラス定数
interface
I
{
// We may naively assume that the PHP constant is always a string.
const
PHP
=
'PHP 8.2'
;
}
class
Foo
implements
I
{
// But implementing classes may define it as an array.
const
PHP
=
[];
}

interface
I
{
const
string PHP
=
'PHP 8.2'
;
}
class
Foo
implements
I
{
const
string PHP
=
[];
}
// Fatal error: Cannot use array as value for class constant
// Foo::PHP of type string
動的クラス定数フェッチ
class
Foo
{
const
PHP
=
'PHP 8.2'
;
}
$searchableConstant
=
'PHP'
;
var_dump
(
constant
(
Foo
::class .
"::
{
$searchableConstant
}
"
));

class
Foo
{
const
PHP
=
'PHP 8.3'
;
}
$searchableConstant
=
'PHP'
;
var_dump
(
Foo
::
{
$searchableConstant
}
);
New #[Override] attribute
use
PHPUnit\Framework\TestCase
;
final class
MyTest
extends
TestCase
{
protected
$logFile
;
protected function
setUp
():
void
{
$this
->
logFile
=
fopen
(
'/tmp/logfile'
,
'w'
);
}
protected function
taerDown
():
void
{
fclose
(
$this
->
logFile
);
unlink
(
'/tmp/logfile'
);
}
}
// The log file will never be removed, because the
// method name was mistyped (taerDown vs tearDown).

use
PHPUnit\Framework\TestCase
;
final class
MyTest
extends
TestCase
{
protected
$logFile
;
protected function
setUp
():
void
{
$this
->
logFile
=
fopen
(
'/tmp/logfile'
,
'w'
);
}
#[
\Override
]
protected function
taerDown
():
void
{
fclose
(
$this
->
logFile
);
unlink
(
'/tmp/logfile'
);
}
}
// The log file will never be removed, because the
// method name was mistyped (taerDown vs tearDown).
By adding the #[Override] attribute to a method, PHP will ensure that a method with the same name exists in a parent class or in an implemented interface. Adding the attribute makes it clear that overriding a parent method is intentional and simplifies refactoring, because the removal of an overridden parent method will be detected.
読み取り専用プロパティのディープクローン作成
class
PHP
{
public
string $version
=
'8.2'
;
}
readonly class
Foo
{
public function
__construct
(
public
PHP $php
) {}
public function
__clone
():
void
{
$this
->
php
= clone
$this
->
php
;
}
}
$instance
= new
Foo
(new
PHP
());
$cloned
= clone
$instance
;
// Fatal error: Cannot modify readonly property Foo::$php

class
PHP
{
public
string $version
=
'8.2'
;
}
readonly class
Foo
{
public function
__construct
(
public
PHP $php
) {}
public function
__clone
():
void
{
$this
->
php
= clone
$this
->
php
;
}
}
$instance
= new
Foo
(new
PHP
());
$cloned
= clone
$instance
;
$cloned
->
php
->
version
=
'8.3'
;
readonly プロパティをマジック __clone メソッド内で 1 回変更することで、readonly プロパティのディープ クローン作成が可能になるようになりました。
新しい json_validate() 関数
function
json_validate
(
string $string
):
bool
{
json_decode
(
$string
);
return
json_last_error
() ===
JSON_ERROR_NONE
;
}
var_dump
(
json_validate
(
'{ "test": { "foo": "bar" }
}'
));
// true

var_dump
(
json_validate
(
'{ "test": { "foo": "bar" }
}'
));
// true
json_validate() を使用すると、文字列が構文的に有効な JSON かどうかをチェックでき、json_decode() よりも効率的です。
新しい Randomizer::getBytesFromString() メソッド
// This function needs to be manually implemented.
function
getBytesFromString
(
string $string
,
int
$length
) {
$stringLength
=
strlen
(
$string
);
$result
=
''
;
for (
$i
=
0
;
$i
<
$length
;
$i
++) {
// random_int is not seedable for testing, but secure.
$result
.=
$string
[
random_int
(
0
,
$stringLength
-
1
)];
}
return
$result
;
}
$randomDomain
=
sprintf
(
"%s.example.com"
,
getBytesFromString
(
'abcdefghijklmnopqrstuvwxyz0123456789'
,
16
,
),
);
echo
$randomDomain
;

// A \Random\Engine may be passed for seeding,
// the default is the secure engine.
$randomizer
= new
\Random\Randomizer
();
$randomDomain
=
sprintf
(
"%s.example.com"
,
$randomizer
->
getBytesFromString
(
'abcdefghijklmnopqrstuvwxyz0123456789'
,
16
,
),
);
echo
$randomDomain
;
PHP 8.2 で追加された Random Extension は、特定のバイトのみで構成されるランダムな文字列を生成する新しいメソッドによって拡張されました。この方法を使用すると、開発者はドメイン名などのランダムな識別子や任意の長さの数値文字列を簡単に生成できます。
新しい Randomizer::getFloat() メソッドと Randomizer::nextFloat() メソッド
// Returns a random float between $min and $max, both including.
function
getFloat
(
float $min
,
float $max
) {
// This algorithm is biased for specific inputs and may
// return values outside the given range. This is impossible
// to work around in userland.
$offset
=
random_int
(
0
,
PHP_INT_MAX
) /
PHP_INT_MAX
;
return
$offset
* (
$max
-
$min
) +
$min
;
}
$temperature
=
getFloat
(-
89.2
,
56.7
);
$chanceForTrue
=
0.1
;
// getFloat(0, 1) might return the upper bound, i.e. 1,
// introducing a small bias.
$myBoolean
=
getFloat
(
0
,
1
) <
$chanceForTrue
;

$randomizer
= new
\Random\Randomizer
();
$temperature
=
$randomizer
->
getFloat
(
-89.2
,
56.7
,
\Random\IntervalBoundary
::
ClosedClosed
,
);
$chanceForTrue
=
0.1
;
// Randomizer::nextFloat() is equivalent to
// Randomizer::getFloat(0, 1, \Random\IntervalBoundary::ClosedOpen).
// The upper bound, i.e. 1, will not be returned.
$myBoolean
=
$randomizer
->
nextFloat
() <
$chanceForTrue
;
浮動小数点数の精度の制限と暗黙的な丸めのため、特定の間隔内にある不偏浮動小数点数を生成することは簡単ではなく、一般的に使用されるユーザーランド ソリューションでは偏った結果や要求された範囲外の数値が生成される可能性があります。
Randomizer も拡張され、不偏な方法でランダムな浮動小数点を生成する 2 つのメソッドが追加されました。 Randomizer::getFloat() メソッドは、「間隔からランダムな浮動小数点数を描画する」で公開された γ セクション アルゴリズムを使用します。フレデリック・グアラール、ACM トランスモデル。計算します。サイマル、32:3、2022。
コマンドラインリンターは複数のファイルをサポートします
php -l foo.php bar.php
No syntax errors detected in foo.php

php -l foo.php bar.php
No syntax errors detected in foo.php
No syntax errors detected in bar.php
コマンド ライン リンターは、lint するファイル名の可変長入力を受け入れるようになりました。
新しいクラス、インターフェイス、関数
- 新しい DOMElement::getAttributeNames()、DOMElement::insertAdjacentElement()、DOMElement::insertAdjacentText()、DOMElement::toggleAttribute()、DOMNode::contains()、DOMNode::getRootNode()、DOMNode::isEqualNode()、 DOMNameSpaceNode::contains() メソッドと DOMParentNode::replaceChildren() メソッド。
- 新しい IntlCalendar::setDate()、IntlCalendar::setDateTime()、IntlGregorianCalendar::createFromDate()、および IntlGregorianCalendar::createFromDateTime() メソッド。
- 新しい ldap_connect_wallet() および ldap_exop_sync() 関数。
- 新しい mb_str_pad() 関数。
- 新しい posix_sysconf()、posix_pathconf()、posix_fpathconf()、および posix_eaccess() 関数。
- 新しい ReflectionMethod::createFromMethodName() メソッド。
- 新しいsocket_atmark()関数。
- 新しい str_increment()、str_decrement()、および stream_context_set_options() 関数。
- 新しい ZipArchive::getArchiveFlag() メソッド。
- Support for generation EC keys with custom EC parameters in OpenSSL extension.
- 新しい INI 設定 zend.max_allowed_stack_size は、最大許容スタック サイズを設定します。
- php.ini は、フォールバック/デフォルト値構文をサポートするようになりました。
- 匿名クラスを読み取り専用にできるようになりました。
非推奨と下位互換性の中断
- より適切な日付/時刻の例外。
- 空の配列に負のインデックス n を代入すると、次のインデックスは 0 ではなく n 1 になります。
- range() 関数に変更します。
- Changes in re-declaration of static properties in traits.
- U_MULTIPLE_DECIMAL_SEPERATORS 定数は非推奨になり、U_MULTIPLE_DECIMAL_SEPARATORS が優先されます。
- MT_RAND_PHP Mt19937 バリアントは非推奨になりました。
- ReflectionClass::getStaticProperties() は null 可能ではなくなりました。
- INI 設定のassert.active、assert.bail、assert.callback、assert.Exception、およびassert.warningは非推奨になりました。
- 引数なしで get_class() および get_parent_class() を呼び出すことは非推奨です。
- SQLite3: デフォルトのエラー モードは例外に設定されています。
移行ガイドは PHP マニュアルで入手できます。新機能と下位互換性のない変更の詳細なリストについては、このガイドを参照してください。
以前のバージョンをダウンロードする必要がある場合は、以下を参照してください。 その他の変更ログ