Une structure de données linéaire pour stocker les éléments de manière non contiguë est appelée LinkedList dans laquelle les pointeurs sont utilisés pour relier les éléments de la liste chaînée les uns aux autres et l'espace de noms System.Collections.Generic se compose de LinkedList< T> classe en C# dont les éléments peuvent être supprimés ou insérés de manière très rapide en implémentant une liste chaînée classique et l'allocation de chaque objet est séparée dans la liste chaînée et il n'est pas nécessaire de copier la collection entière pour effectuer certaines opérations sur la liste chaînée.
Syntaxe :
La syntaxe de la classe LinkedList en C# est la suivante :
LinkedList<Type> linkedlist_name = new LinkedList <Type>();
Copier après la connexion
Où Type représente le type de liste chaînée.
Fonctionnement de la classe LinkedList en C#
- Il y a des nœuds présents dans la liste chaînée et chaque nœud se compose de deux parties, à savoir un champ de données et un lien vers le nœud qui vient ensuite dans la liste chaînée.
- Le type de chaque nœud de la liste chaînée est LinkedListNode tapez.
- Un nœud peut être supprimé de la liste chaînée et peut être réinséré dans la même liste chaînée ou peut être inséré dans une autre liste chaînée et il n'y a donc pas d'allocation supplémentaire sur le tas.
- Insérer les éléments dans une liste chaînée, supprimer les éléments de la liste chaînée et obtenir la propriété de count qui est une propriété interne maintenue par la liste aimée sont toutes des opérations O(1).
- Les énumérateurs sont pris en charge par la classe de liste chaînée car il s'agit d'une liste chaînée à usage général.
- Rien qui rend la liste chaînée incohérente n'est pris en charge par la liste chaînée.
- Si la liste chaînée est une liste doublement chaînée, alors chaque nœud a deux pointeurs, l'un pointant vers le nœud précédent de la liste et l'autre pointant vers le nœud suivant de la liste.
Constructeurs de classe LinkedList
Il existe plusieurs constructeurs dans la classe LinkedList en C#. Ce sont :
-
LinkedList() : Une nouvelle instance de la classe de liste chaînée est initialisée et est vide.
-
LinkedList(IEnumerable) : Une nouvelle instance de la classe de liste chaînée est initialisée et est extraite de l'implémentation spécifiée de IEnumerable dont la capacité est suffisante pour accumuler tous les éléments copiés.
-
LinkedList(SerializationInfo, StreamingContext) : Une nouvelle instance de la classe de liste chaînée est initialisée et peut être sérialisée avec SerializationInfo et StreamingContext spécifiés comme paramètres.
Méthodes de classe LinkedList en C#
Il existe plusieurs méthodes dans la classe LinkedList en C#. Ce sont :
-
AddAfter: A value or new node is added after an already present node in the linked list using the AddAfter method.
-
AddFirst: A value or new node is added at the beginning of the linked list using the AddFirst method.
-
AddBefore: A value or new node is added before an already present node in the linked list using the AddBefore method.
-
AddLast: A value or new node is added at the end of the linked list using the AddLast method.
-
Remove(LinkedListNode): A node specified as a parameter will be removed from the linked list using Remove(LinkedListNode) method.
-
RemoveFirst(): A node at the beginning of the linked list will be removed from the linked list using RemoveFirst() method.
-
Remove(T): The first occurrence of the value specified as a parameter in the linked list will be removed from the linked list using the Remove(T) method.
-
RemoveLast(): A node at the end of the linked list will be removed from the linked list using the RemoveLast() method.
-
Clear(): All the nodes from the linked list will be removed using the Clear() method.
-
Find(T): The value specified as the parameter present in the very first node will be identified by using the Find(T) method.
-
Contains(T): We can use the Contains(T) method to find out if a value is present in the linked list or not.
-
ToString(): A string representing the current object is returned by using the ToString() method.
-
CopyTo(T[], Int32): The whole linked list is copied to an array which is one dimensional and is compatible with the linked list and the linked list begins at the index specified in the array to be copied to using CopyTo(T[], Int32) method.
-
OnDeserialization(Object): After the completion of deserialization, an event of deserialization is raised and the ISerializable interface is implemented using OnDeserialization(Object) method.
-
Equals(Object): If the object specified as the parameter is equal to the current object or not is identified using Equals(Object) method.
-
FindLast(T): The value specified as the parameter present in the last node will be identified by using FindLast(T) method.
-
MemberwiseClone(): A shallow copy of the current object is created using MemeberwiseClone() method.
-
GetEnumerator(): An enumerator is returned using GetEnumerator() method and the returned enumerator loops through the linked list.
-
GetType(): The type of the current instance is returned using GetType() method.
-
GetHashCode(): The GetHashCode() method is the hash function by default.
-
GetObjectData(SerializationInfo, StreamingContext): The data which is necessary to make the linked list serializable is returned by using GetObjectData(SerializationInfo, StreamingContext) method along with implementing the ISerializable interface.
Example of LinkedList Class in C#
C# program to demonstrate AddLast() method, Remove(LinkedListNode) method, Remove(T) method, RemoveFirst() method, RemoveLast() method and Clear() method in Linked List class:
Code:
using System;
using System.Collections.Generic;
//a class called program is defined
public class program
{
// Main Method is called
static public void Main()
{
//a new linked list is created
LinkedList<String> list = new LinkedList<String>();
//AddLast() method is used to add the elements to the newly created linked list
list.AddLast("Karnataka");
list.AddLast("Mumbai");
list.AddLast("Pune");
list.AddLast("Hyderabad");
list.AddLast("Chennai");
list.AddLast("Delhi");
Console.WriteLine("The states in India are:");
//Using foreach loop to display the elements of the newly created linked list
foreach(string places in list)
{
Console.WriteLine(places);
}
Console.WriteLine("The places after using Remove(LinkedListNode) method are:");
//using Remove(LinkedListNode) method to remove a node from the linked list
list.Remove(list.First);
foreach(string place in list)
{
Console.WriteLine(place);
}
Console.WriteLine("The places after using Remove(T) method are:");
//using Remove(T) method to remove a node from the linked list
list.Remove("Chennai");
foreach(string plac in list)
{
Console.WriteLine(plac);
}
Console.WriteLine("The places after using RemoveFirst() method are:");
//using RemoveFirst() method to remove the first node from the linked list
list.RemoveFirst();
foreach(string pla in list)
{
Console.WriteLine(pla);
}
Console.WriteLine("The places after using RemoveLast() method are:");
//using RemoveLast() method to remove the last node from the linked list
list.RemoveLast();
foreach(string pl in list)
{
Console.WriteLine(pl);
}
//using Clear() method to remove all the nodes from the linked list
list.Clear();
Console.WriteLine("The count of places after using Clear() method is: {0}",
list.Count);
}
}
Copier après la connexion
The output of the above program is as shown in the snapshot below:
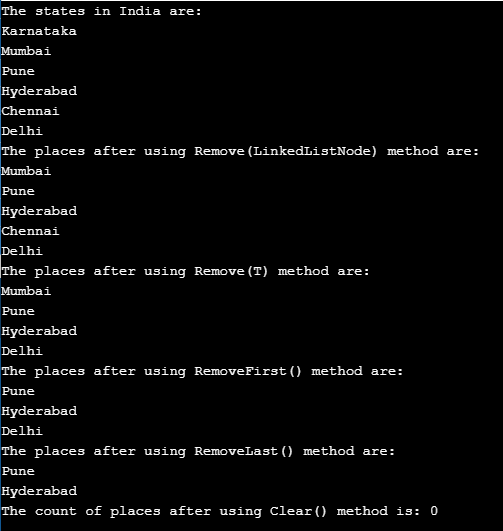
In the above program, a class called program is defined. Then the main method is called. Then a new linked list is created. Then AddLast() method is used to add the elements to the newly created linked list. Then foreach loop is used to display the elements of the newly created linked list. Then Remove(LinkedListNode) method is used to remove a node from the linked list. Then Remove(T) method is used to remove a node from the linked list. Then RemoveFirst() method is used to remove the first node from the linked list. Then RemoveLast() method is used to remove the last node from the linked list. Then Clear() method is used to remove all the nodes from the linked list. The output of the program is shown in the snapshot above.
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!