En Java, Scanner est une classe utilisée pour obtenir l'entrée de chaînes et de différents types primitifs tels que int, double, etc. La classe scanner se trouve dans le package java. Il étend la classe Object et implémente les interfaces Closeable et Iterator. Les entrées sont divisées en classes à l’aide d’un délimiteur d’espaces. La déclaration, la syntaxe, le fonctionnement et plusieurs autres aspects de la classe Scanner en Java seront abordés dans les sections suivantes.
Commencez votre cours de développement de logiciels libres
Développement Web, langages de programmation, tests de logiciels et autres
Déclaration de classe Java Scanner
La classe Java Scanner est déclarée comme indiqué ci-dessous.
public final class Scanner extends Object implements Iterator<String>
Copier après la connexion
Syntaxe :
La classe Scanner peut être utilisée dans la syntaxe ci-dessous dans les programmes Java.
Scanner sc = new Scanner(System.in);
Copier après la connexion
Ici, sc est l'objet scanner et System.in indique à Java que l'entrée sera celle-ci.
Comment fonctionne la classe Java Scanner ?
Voyons maintenant comment fonctionne la classe Scanner.
- Importez la classe java.util.Scanner ou l'ensemble du package java.util.
import java.util.*;
import java.util.Scanner;
Copier après la connexion
- Créez un objet scanner comme indiqué dans la syntaxe.
Scanner s = new Scanner(System.in);
Copier après la connexion
- Déclarez un type int, char ou string.
int n = sc.nextInt();
Copier après la connexion
- Effectuez les opérations sur l'entrée en fonction des exigences.
Constructeurs
La classe scanner en Java a différents constructeurs. Ce sont :
-
Scanner (File src) : Un nouveau scanner sera construit qui génère de la valeur à partir du fichier mentionné.
-
Scanner(File src, String charsetName) : Un nouveau scanner sera construit qui génère une valeur numérisée à partir du fichier mentionné.
-
Scanner (InputStream src) : Un nouveau scanner sera construit qui génère de la valeur à partir du flux d'entrée mentionné.
-
Scanner(InputStream src, String charsetName) : Un nouveau scanner sera construit qui génère de la valeur à partir du flux d'entrée mentionné.
-
Scanner (Path src) : Un nouveau scanner sera construit qui génère de la valeur à partir du fichier mentionné.
-
Scanner(Path src, String charsetName) : Un nouveau scanner sera construit et générera de la valeur à partir du fichier mentionné.
-
Scanner (Readable src) : Un nouveau scanner sera construit qui génère de la valeur à partir de la source mentionnée.
-
Scanner (ReadableByteChannel src) : Un nouveau scanner sera construit qui génère de la valeur à partir du canal mentionné.
-
Scanner (ReadableByteChannel src, String charsetName) : Un nouveau scanner sera construit qui génère de la valeur à partir du canal mentionné.
-
Scanner(String src) : Un nouveau scanner sera construit qui génère de la valeur à partir de la chaîne mentionnée.
Méthodes de la classe Java Scanner
Voici les méthodes qui exécutent différentes fonctionnalités.
-
close(): Scanner gets closed on calling this method.
-
findInLine(Pattern p): Next occurrence of the mentioned pattern p will be attempted to find out, ignoring the delimiters.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
delimiter(): Pattern that is currently used by the scanner to match delimiters will be returned.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
findWithinHorizon(Pattern p, int horizon): Tries to identify the mentioned pattern’s next occurrence.
-
hasNext(): If the scanner has another token in the input, true will be returned.
-
findWithinHorizon(String p, int horizon): Tries to identify the next occurrence of the mentioned pattern made from the string p, ignoring delimiters.
-
hasNext(Pattern p): If the next token matches the mentioned pattern p, true will be returned.
-
hasNext(String p): If the next token matches the mentioned pattern p made from the string p, true will be returned.
-
next(Pattern p): Next token will be returned, which matches the mentioned pattern p.
-
next(String p): Next token will be returned, which matches the mentioned pattern p made from the string p.
-
nextBigDecimal(): Input’s next token will be scanned as a BigDecimal.
-
nextBigInteger(): Input’s next token will be scanned as a BigInteger.
-
nextBigInteger(int rad): Input’s next token will be scanned as a BigInteger.
-
nextBoolean(): Input’s next token will be scanned as a Boolean, and it will be returned.
-
nextByte(): Input’s next token will be scanned as a byte.
-
nextByte(int rad): Input’s next token will be scanned as a byte.
-
nextDouble(): Input’s next token will be scanned as a double.
-
nextFloat(): Input’s next token will be scanned as a float.
-
nextInt(): Input’s next token will be scanned as an integer.
-
nextInt(int rad): Input’s next token will be scanned as an integer.
-
nextLong(int rad): Input’s next token will be scanned as a long.
-
nextLong(): Input’s next token will be scanned as a long.
-
nextShort(int rad): Input’s next token will be scanned as a short.
-
nextShort(): Input’s next token will be scanned as a short.
-
radix(): The default radix of the scanner will be returned.
-
useDelimiter(Pattern p): Delimiting pattern of the scanner will be set to the mentioned pattern.
-
useDelimiter(String p): Delimiting pattern of the scanner will be set to the mentioned pattern made from the string p.
-
useRadix(int rad): The scanner’s default radix will be set to the mentioned radix.
-
useLocale(Locale loc): The locale of the scanner will be set to the mentioned locale.
Examples of Java Scanner Class
Now, let us see some sample programs of the Scanner class in Java.
Example #1
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter the username");
String obj = sc.nextLine();
System.out.println("The name you entered is: " + obj);
}
}
Copier après la connexion
Output:
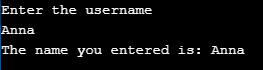
On executing the program, the user will be asked to enter a name. As the input is a string, the nextLine() method will be used. Once it is entered, a line will be printed displaying the name user given as input.
Example #2
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter age");
int obj = sc.nextInt();
System.out.println("The age you entered is: " + obj);
}
}
Copier après la connexion
Output:
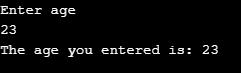
On executing the program, the user will be asked to enter the age. As the input is a number, the nextInt() method will be used. Once it is entered, a line will be printed displaying the age user given as input.
Ce qui précède est le contenu détaillé de. pour plus d'informations, suivez d'autres articles connexes sur le site Web de PHP en chinois!