


Best Practices for Deep Copying PHP Arrays: Discover Efficient Methods
The best practice for performing an array deep copy in PHP is to use json_decode(json_encode($arr)) to convert the array to a JSON string and then convert it back to an array. Use unserialize(serialize($arr)) to serialize the array to a string and then deserialize it to a new array. Use the RecursiveIteratorIterator to recursively traverse multidimensional arrays.
Best practices for deep copying PHP arrays: exploring efficient methods
In PHP, arrays are a widely used Commonly used data structures for storing and processing data. In some cases, you may want to create a copy of an array so that you can operate on the copy without affecting the original array. This is called a deep copy.
Why choose deep copy?
One of the main benefits of deep copying is that it ensures that the original array and the copy are independent of each other. Modifications made to the copy do not affect the original array. This is useful in situations such as caching and concurrency scenarios.
Deep copy method
In PHP, there are several ways to perform deep copy:
- ##json_decode(json_encode( $arr));: Use json_encode()
to convert the array to a JSON string, and then use
json_decode()to convert it to a new array.
- unserialize(serialize($arr));: Serialize the array into a string, then use unserialize()
to deserialize it into a new array.
- RecursiveIteratorIterator: This is an SPL iterator that supports recursive traversal of multidimensional arrays.
Real Case
Consider the following example:$original_array = ['name' => 'John', 'age' => 30, 'scores' => [95, 85, 90]]; // 使用 json_decode() 进行深度复制 $copied_array = json_decode(json_encode($original_array), true); // 修改副本 $copied_array['name'] = 'Jane'; // 输出原始和复制的数组 echo '原始数组:'; var_dump($original_array); echo '复制的数组:'; var_dump($copied_array);In the above example,
json_encode() will The original array is converted to a JSON string,
json_decode() converts it to a new array. Then, the copied array is modified without affecting the original array.
The above is the detailed content of Best Practices for Deep Copying PHP Arrays: Discover Efficient Methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undress AI Tool
Undress images for free

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
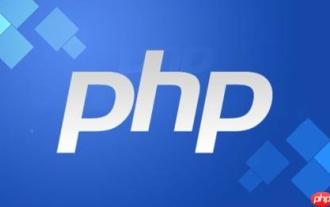
User voice input is captured and sent to the PHP backend through the MediaRecorder API of the front-end JavaScript; 2. PHP saves the audio as a temporary file and calls STTAPI (such as Google or Baidu voice recognition) to convert it into text; 3. PHP sends the text to an AI service (such as OpenAIGPT) to obtain intelligent reply; 4. PHP then calls TTSAPI (such as Baidu or Google voice synthesis) to convert the reply to a voice file; 5. PHP streams the voice file back to the front-end to play, completing interaction. The entire process is dominated by PHP to ensure seamless connection between all links.
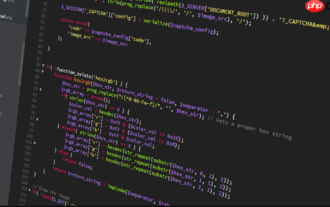
The core method of building social sharing functions in PHP is to dynamically generate sharing links that meet the requirements of each platform. 1. First get the current page or specified URL and article information; 2. Use urlencode to encode the parameters; 3. Splice and generate sharing links according to the protocols of each platform; 4. Display links on the front end for users to click and share; 5. Dynamically generate OG tags on the page to optimize sharing content display; 6. Be sure to escape user input to prevent XSS attacks. This method does not require complex authentication, has low maintenance costs, and is suitable for most content sharing needs.
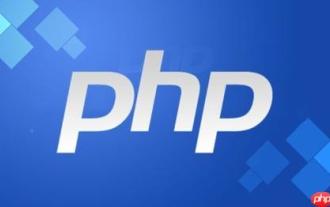
To realize text error correction and syntax optimization with AI, you need to follow the following steps: 1. Select a suitable AI model or API, such as Baidu, Tencent API or open source NLP library; 2. Call the API through PHP's curl or Guzzle and process the return results; 3. Display error correction information in the application and allow users to choose whether to adopt it; 4. Use php-l and PHP_CodeSniffer for syntax detection and code optimization; 5. Continuously collect feedback and update the model or rules to improve the effect. When choosing AIAPI, focus on evaluating accuracy, response speed, price and support for PHP. Code optimization should follow PSR specifications, use cache reasonably, avoid circular queries, review code regularly, and use X
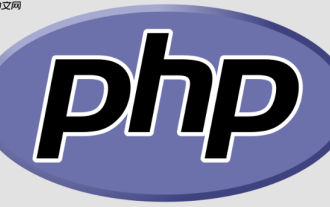
PHP ensures inventory deduction atomicity through database transactions and FORUPDATE row locks to prevent high concurrent overselling; 2. Multi-platform inventory consistency depends on centralized management and event-driven synchronization, combining API/Webhook notifications and message queues to ensure reliable data transmission; 3. The alarm mechanism should set low inventory, zero/negative inventory, unsalable sales, replenishment cycles and abnormal fluctuations strategies in different scenarios, and select DingTalk, SMS or Email Responsible Persons according to the urgency, and the alarm information must be complete and clear to achieve business adaptation and rapid response.
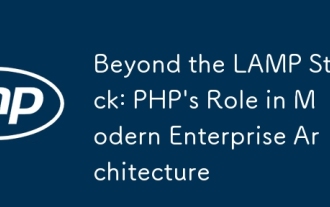
PHPisstillrelevantinmodernenterpriseenvironments.1.ModernPHP(7.xand8.x)offersperformancegains,stricttyping,JITcompilation,andmodernsyntax,makingitsuitableforlarge-scaleapplications.2.PHPintegrateseffectivelyinhybridarchitectures,servingasanAPIgateway
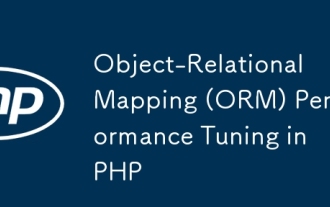
Avoid N 1 query problems, reduce the number of database queries by loading associated data in advance; 2. Select only the required fields to avoid loading complete entities to save memory and bandwidth; 3. Use cache strategies reasonably, such as Doctrine's secondary cache or Redis cache high-frequency query results; 4. Optimize the entity life cycle and call clear() regularly to free up memory to prevent memory overflow; 5. Ensure that the database index exists and analyze the generated SQL statements to avoid inefficient queries; 6. Disable automatic change tracking in scenarios where changes are not required, and use arrays or lightweight modes to improve performance. Correct use of ORM requires combining SQL monitoring, caching, batch processing and appropriate optimization to ensure application performance while maintaining development efficiency.
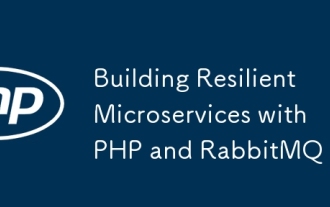
To build a flexible PHP microservice, you need to use RabbitMQ to achieve asynchronous communication, 1. Decouple the service through message queues to avoid cascade failures; 2. Configure persistent queues, persistent messages, release confirmation and manual ACK to ensure reliability; 3. Use exponential backoff retry, TTL and dead letter queue security processing failures; 4. Use tools such as supervisord to protect consumer processes and enable heartbeat mechanisms to ensure service health; and ultimately realize the ability of the system to continuously operate in failures.
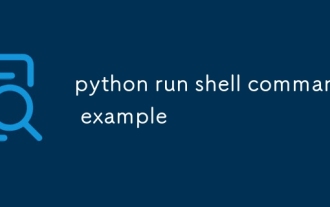
Use subprocess.run() to safely execute shell commands and capture output. It is recommended to pass parameters in lists to avoid injection risks; 2. When shell characteristics are required, you can set shell=True, but beware of command injection; 3. Use subprocess.Popen to realize real-time output processing; 4. Set check=True to throw exceptions when the command fails; 5. You can directly call chains to obtain output in a simple scenario; you should give priority to subprocess.run() in daily life to avoid using os.system() or deprecated modules. The above methods override the core usage of executing shell commands in Python.
