Methods to use the compiler to check C code errors include: using the GCC/Clang compiler and enabling warning options (-Wall, -Wextra); using the -g flag to generate debugging information; adding assertions to check runtime conditions ;Use setjmp()/longjmp() to handle errors; use debuggers such as gdb/lldb. Grammatical errors include missing semicolons, mismatched brackets, and misspelled keywords. Semantic errors include type mismatches, function declaration issues, and pointer errors. Other tips: Use lint tools, pair programming, and unit testing.
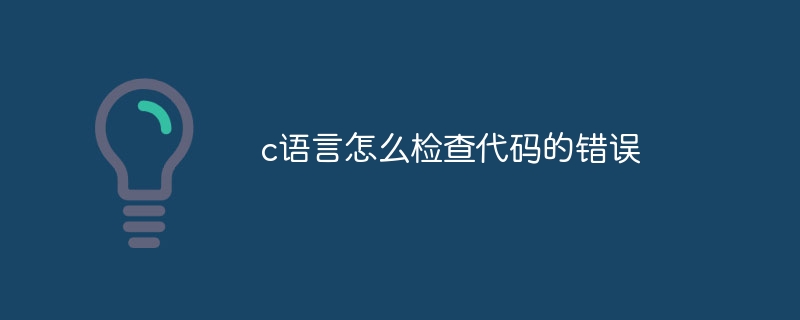
How to check C language code for errors
Compiler errors
-
Use a compiler: Use a compiler such as GCC or Clang to check for syntax errors and semantic errors. They generate a list of error messages indicating the error line number and description.
-
Compile options: Use the -Wall and -Wextra options when compiling to enable additional warnings to help identify potential problems.
-
Compiler flags: Compiling code with the -g flag generates debugging information for debugging the code at runtime.
Runtime Error
-
Assertion: Use the assert() function to check the runtime condition if the condition is false , the program is terminated and an error message is printed.
-
Error handling: Use the setjmp() and longjmp() functions to capture and handle errors.
-
Debugger: Use a debugger such as gdb or lldb to step through code and inspect variable values at runtime.
Syntax error
-
Missing semicolon: There must be a semicolon at the end of each C statement.
-
Bracket mismatch: All opening brackets must have corresponding closing brackets.
-
Keyword misspelling: Check carefully whether the C keyword is spelled correctly.
Semantic Error
-
Type mismatch: Variables and function calls must have the correct type.
-
Function declaration: Function must be declared before calling.
-
Pointer error: Ensure that access to the pointer is valid and does not cause a segfault.
Other tips
-
Use lint tools: You can use tools such as lint to identify potential errors and code smells.
-
Pair Programming: Reviewing code with others can help find errors.
-
Unit testing: Writing unit tests can automatically check the correctness of the code.
The above is the detailed content of How to check code errors in C language. For more information, please follow other related articles on the PHP Chinese website!