Conditional control structures in PHP
Conditional control structure in PHP
In PHP programming, conditional control structure is a very important syntax, which allows the program to execute according to different conditions different code blocks. By using conditional control structures, we can implement the branching logic of the program and determine the execution path of the program based on the result of the condition. This article will introduce the commonly used conditional control structures in PHP, including if statements, else statements, elseif statements and switch statements, and give specific code examples.
The if statement is the most basic conditional control structure in PHP, used to execute code blocks based on conditions. The basic syntax is as follows:
if (条件) { // 如果条件为真,执行这里的代码 }
Specific example:
$score = 80; if ($score >= 60) { echo "成绩及格"; }
In the above example, if $score is greater than or equal to 60, "passing grade" will be output.
In addition to the if statement, we can also use the else statement to execute the specified code block when the condition is not true. The syntax is as follows:
if (条件) { // 如果条件为真,执行这里的代码 } else { // 如果条件为假,执行这里的代码 }
Specific example:
$score = 50; if ($score >= 60) { echo "成绩及格"; } else { echo "成绩不及格"; }
In the above example, if $score is less than 60, "Failed Grade" will be output.
When we need to select and execute different code blocks under multiple conditions, we can use the elseif statement. The syntax is as follows:
if (条件1) { // 如果条件1为真,执行这里的代码 } elseif (条件2) { // 如果条件2为真,执行这里的代码 } else { // 如果以上条件都不成立,执行这里的代码 }
Specific example:
$score = 70; if ($score >= 90) { echo "优秀"; } elseif ($score >= 80) { echo "良好"; } elseif ($score >= 60) { echo "及格"; } else { echo "不及格"; }
In the above example, different levels are output based on the different scores of $score.
In addition to the if statement series, PHP also provides switch statements to handle multiple conditions. The switch statement is suitable for making selections among a series of fixed values. The syntax is as follows:
switch (表达式) { case 值1: // 如果表达式等于值1,执行这里的代码 break; case 值2: // 如果表达式等于值2,执行这里的代码 break; default: // 如果以上所有情况都不符合,执行这里的代码 }
Specific example:
$fruit = 'apple'; switch ($fruit) { case 'apple': echo "苹果"; break; case 'banana': echo "香蕉"; break; default: echo "其他水果"; }
In the above example, different fruit names are output based on the value of $fruit.
In short, the conditional control structure plays an important role in PHP programming. By rationally using if, else, elseif and switch statements, we can control the execution flow of the program according to different conditions and achieve flexible logic. branch. I hope that through the introduction of this article, readers can better understand the usage of conditional control structures in PHP and be able to flexibly apply them to actual programming.
The above is the detailed content of Conditional control structures in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undress AI Tool
Undress images for free

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
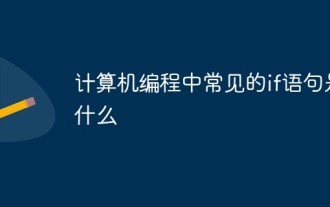
A common if statement in computer programming is a conditional judgment statement. The if statement is a selective branch structure. It selects the execution path based on clear conditions, rather than strictly following the order. In actual programming, the appropriate branch statement must be selected according to the program flow. It changes the execution according to the result of the condition. program; the simple syntax of the if statement is "if (conditional expression) {//code to be executed;}".
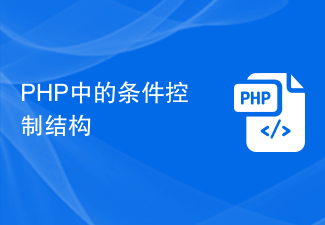
Conditional control structure in PHP In PHP programming, conditional control structure is a very important syntax, which allows the program to execute different code blocks based on different conditions. By using conditional control structures, we can implement the branching logic of the program and determine the execution path of the program based on the result of the condition. This article will introduce the commonly used conditional control structures in PHP, including if statements, else statements, elseif statements and switch statements, and give specific code examples. The if statement is the most basic conditional control in PHP
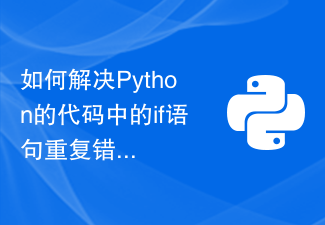
Python is a very powerful and popular programming language that is widely used in fields such as data analysis, machine learning, and web development. However, when writing Python code, we will inevitably encounter repeated if statements, which may lead to problems such as inefficient code and complicated maintenance. Therefore, this article will introduce some methods and techniques to solve repeated if statement errors in Python code. Simplify if statements using Boolean operators In many cases, repeated logic in an if statement can be simplified into Boolean operations. example
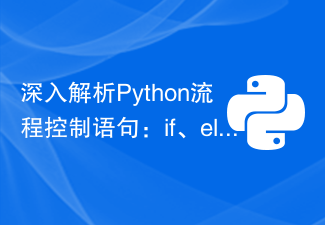
Detailed explanation of Python flow control statements: if, else, elif, while, for In programming, flow control statements are essential. They are used to determine the execution flow of the program based on conditions. Python provides several commonly used flow control statements, including if, else, elif, while and for. This article explains these statements in detail and provides specific code examples. if statement The if statement is used to determine whether a certain condition is true. If the condition is true, execute the if code block.
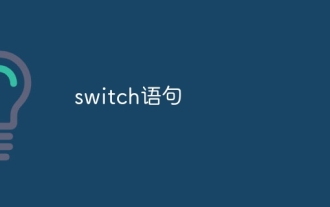
The switch statement is a control structure commonly used in programming, which allows the program to execute different code blocks based on different condition values. It can replace multiple if-else statements to improve the readability and maintainability of the code. Although it has some limitations, under the right circumstances, using the Switch statement can make programs more concise and efficient.
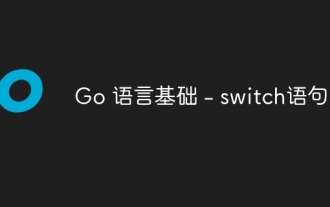
switch is a conditional statement that is used to calculate the value of a conditional expression to determine whether the value satisfies the case statement. If it matches, the corresponding code block will be executed. Is a common way to replace complex if-else statements.
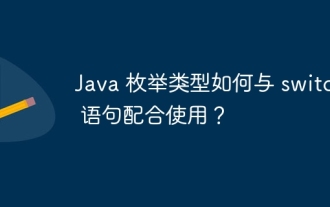
Enumeration type is a data type in Java that defines a collection of constants. With the switch statement, the following functions can be achieved: Clearly represent the value range: The enumeration type is used to define a set of immutable constant values to improve code readability. Matching different enumeration constants: The switch statement allows different operations to be performed based on the enumeration constants to achieve refined control. Handling different scenarios: Through enumeration types and switch statements, various situations can be flexibly handled in actual scenarios, such as sending different email contents according to different notification types.
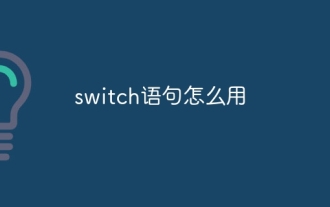
Switch statement usage: 1. The Switch statement can only be used for integer types, enumeration types and String types, and cannot be used for floating point types and Boolean types; 2. Each case statement must be followed by a break statement to prevent other cases from being executed. The code block without a break statement will continue to execute the code block of the next case; 3. Multiple values can be matched in one case statement, separated by commas; 4. The default code block in the Switch statement is optional, etc. wait.
