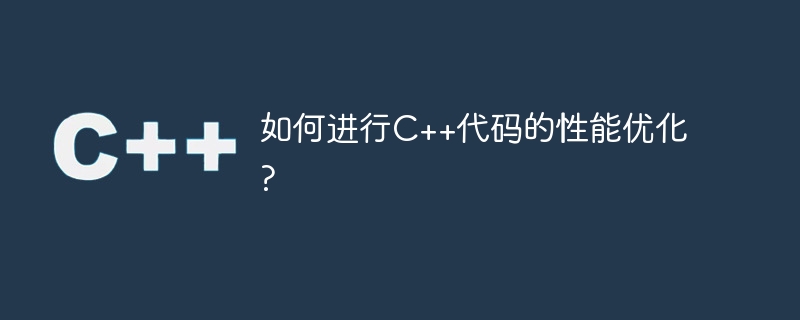
How to optimize the performance of C code?
Performance optimization is a very important part of the software development process. It aims to improve the running speed and efficiency of the program and reduce resource consumption. For performance optimization of C code, it is an even more crucial step. The following will introduce some common and practical C code performance optimization techniques.
- Choose the right data structure:
Choosing the right data structure is crucial to the performance of your C code. For example, if you need to perform frequent lookup operations, you can choose to use a hash table instead of an array; if you need to perform frequent insertion and deletion operations, you can use a linked list instead of an array.
- Reduce the number of memory allocations:
Frequent memory allocation and release will reduce the performance of the program. You can reduce the number of memory allocations by reusing blocks of memory, such as using object pools or memory pools. In addition, try to use variables on the stack instead of variables on the heap.
- Use a more efficient algorithm:
Choosing to use a more efficient algorithm can significantly improve the performance of the program. For example, when searching for elements, you can use binary search instead of linear search; when sorting, you can choose quick sort or merge sort instead of bubble sort.
- Reduce the overhead of function calls:
Function calls will generate a certain overhead, so performance can be improved by reducing the number of function calls. Some commonly used functions or calculation logic can be expanded inline to avoid the overhead of function calls.
- Use more efficient loops:
Loops are common structures in programs, so performance optimization of loops is also a very effective way. For example, you can reduce the amount of calculation inside the loop, reduce unnecessary judgment statements, and use simpler loop structures as much as possible.
- Avoid unnecessary copies and assignments:
Using technologies such as move semantics and references to avoid unnecessary copies and assignments can greatly improve program performance. When implementing a custom class, you can overload the move constructor and move assignment operator.
- Use multi-threaded concurrent programming:
For systems with multi-core processors, using multi-threaded concurrent programming can make full use of system resources and improve program performance. You can use the std::thread library in C++11 or use a third-party concurrency library for multi-threaded programming.
- Perform performance testing and profiling analysis:
For performance optimization, performance testing and profiling analysis must be performed to determine where the performance bottleneck is. You can use the profiler tool to perform performance profiling analysis, find performance bottlenecks in the program, and then optimize them in a targeted manner.
- Avoid over-optimization:
Performance optimization is an art and needs to be carried out while ensuring code quality. Over-optimization can lead to code that is difficult to understand and maintain, so there is a trade-off between performance and code quality.
Performance optimization is an ongoing process that requires constant testing and adjustments. I hope the above C code performance optimization tips can help you improve the performance and efficiency of your program. However, it should be noted that when optimizing performance, various factors must be comprehensively considered according to the specific situation to avoid over-optimization or blind optimization.
The above is the detailed content of How to optimize the performance of C++ code?. For more information, please follow other related articles on the PHP Chinese website!