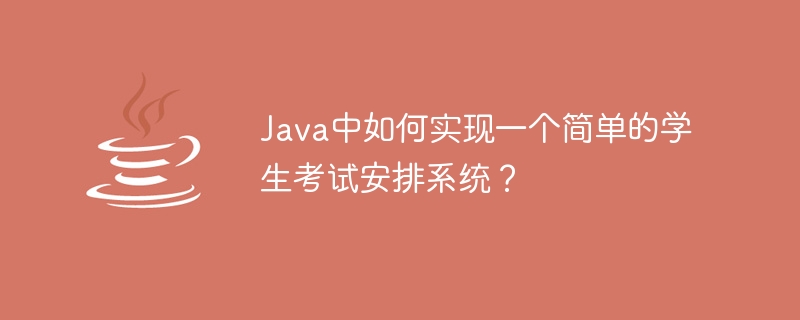
How to implement a simple student exam arrangement system in Java?
With the popularization of education and the expansion of school scale, student examination arrangements have become a tedious and complex task. In order to improve efficiency and reduce labor costs, many schools have begun to adopt computer-assisted examination scheduling systems. This article will introduce how to use the Java programming language to implement a simple student exam scheduling system.
- Requirements Analysis
Before we start writing code, we first need to conduct a requirements analysis. The main functions of the student examination arrangement system include:
- Login to the system: Students and teachers can log in to the system through username and password.
- Test arrangement: Teachers can create exams and specify the exam time, location and related courses.
- Student course selection: Students can choose to take the exams they are interested in and register.
- Exam query: Students and teachers can query scheduled exam information.
- Create database
We can use relational databases such as MySQL to store system user and exam information. First, create the following tables in the database:
- User table: stores username, password and user type (student or teacher).
- Course Schedule: Stores course information, including course name and related information.
- Examination table: stores examination information, including examination time, location, courses and related teachers.
- Student course selection table: stores student course selection information, including student ID and related examination ID.
-
Writing Java Code
Next, we start writing Java code. First, create a Java class to handle user login:
public class UserLogin {
public boolean login(String username, String password) {
// 在数据库中查询用户信息,并验证用户名和密码
// 返回登录结果(成功或失败)
}
}
Copy after login
Then, create a Java class to handle exam scheduling:
public class ExamSchedule {
public void createExam(String time, String location, String course, String teacher) {
// 将考试信息存储到数据库中
}
}
Copy after login
Next, create a Java class to handle Student course selection:
public class CourseSelection {
public void chooseCourse(String studentID, String examID) {
// 将选课信息存储到数据库中
}
}
Copy after login
Finally, create a Java class to handle the exam query:
public class ExamQuery {
public void queryExam(String keyword) {
// 在数据库中查询相关考试信息,并显示在界面上
}
}
Copy after login
- Build the user interface
In order to provide a friendly user interface, we can use the Java graphical user Interface (GUI) library such as Swing or JavaFX. According to needs, functional buttons such as login, exam arrangement, student course selection, and exam inquiry are added to the interface to enable users to use the system conveniently.
- Test the system
After writing the code and interface, we need to comprehensively test the system. Make sure all functions are working properly and the user interface is friendly and easy to use. During the test, you can simulate different users logging in, trying to create exams, select courses, and query exam information to ensure that the system is running normally.
The student examination arrangement system is a relatively complex project. This article only provides a simple implementation plan. In actual use, other factors may need to be considered, such as security, performance optimization, and data backup. However, with the above steps, you can start building and implementing a basic student exam scheduling system.
The above is the detailed content of How to implement a simple student exam scheduling system in Java?. For more information, please follow other related articles on the PHP Chinese website!