How to use define to define constants
define definition constant usage: 1. Define numeric constants, "#define PI value"; 2. Define string constants, "#define GREETING "string""; 3. Define expression constants, " #define MAX(a, b) ((a) > (b) ? (a) : (b))".
`#define` can be used to define constants, making it more convenient and readable when using this constant in code. Common usages are as follows:
1. Define numeric constants:
#define PI 3.14159
When using `PI` in the code, the preprocessor will replace it with `3.14159`. In this way, using `PI` in your code is equivalent to using `3.14159` directly.
2. Define string constants:
#define GREETING "Hello, World!"
When using `GREETING` in the code, the preprocessor will replace it with `"Hello, World!"`. In this way, using `GREETING` in your code is equivalent to using `"Hello, World!"` directly.
3. Define expression constants:
#define MAX(a, b) ((a) > (b) ? (a) : (b))
When using `MAX(5, 10)` in the code, the preprocessor will replace it with `((5) > ( 10) ? (5) : (10))`, that is, `10`. In this way, you can easily use macros to define some commonly used expressions, such as maximum value, minimum value, etc.
It should be noted that the constants defined by `#define` are global and have no scope restrictions. Throughout the code, whenever the preprocessor encounters a macro name, it replaces it with the corresponding replacement text. Therefore, when using macros to define constants, avoid conflicts with other identifiers and carefully consider possible side effects.
The above is the detailed content of How to use define to define constants. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undress AI Tool
Undress images for free

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
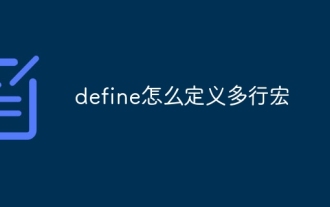
define defines a multi-line macro by using `\` to divide `do { \ printf("%d\n", x); \ } while (0)` into multiple lines for definition. In a macro definition, the backslash `\` must be the last character of the macro definition and cannot be followed by spaces or comments. When using `\` for line continuation, be careful to keep the code readable and make sure there is a `\` at the end of each line.
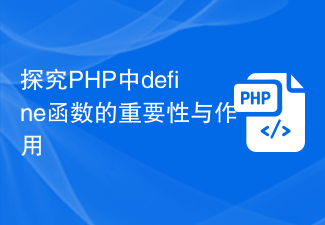
The importance and role of the define function in PHP 1. Basic introduction to the define function In PHP, the define function is a key function used to define constants. Constants will not change their values during the running of the program. Constants defined using the define function can be accessed throughout the script and are global. 2. The syntax of define function The basic syntax of define function is as follows: define("constant name","constant value&qu
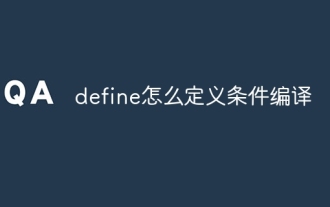
defineConditional compilation can be achieved using the `#ifdef`, `#ifndef`, `#if`, `#elif`, `#else` and `#endif` preprocessing directives.
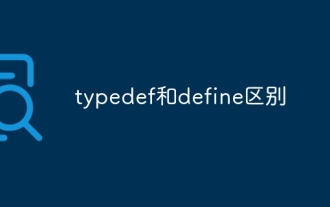
The difference between typedef and define lies in type checking, scope, readability, error handling, memory usage, etc. Detailed introduction: 1. Type checking, the type alias defined by typedef is a real type, and type checking will be performed, while the macro defined by define is just a simple text replacement, and type checking will not be performed; 2. Scope, the type alias defined by typedef The scope of is local and only valid within the current scope, while the macro defined by define is global and can be used anywhere; 3. Readability, etc.

The usage of define function macro: 1. Define a simple calculation macro, "#define SQUARE(x) ((x) * (x))"; 2. Define a macro with multiple parameters, "#define MAX(a , b) ((a) > (b) ? (a) : (b))"; 3. Define macros with complex expressions, "#define ABS(x) ((x) < 0 ? -(x ) : (x))”.
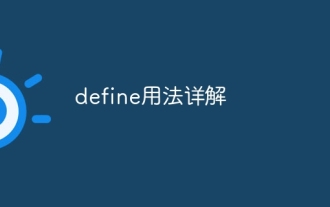
define usage: 1. Define constants; 2. Define function macros: 3. Define conditional compilation; 4. Define multi-line macros.
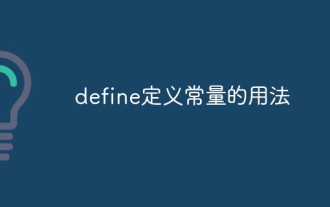
Usage of define constants: 1. Define numeric constants, "#define PI value"; 2. Define string constants, "#define GREETING "string""; 3. Define expression constants, "#define MAX(a, b) ((a) > (b) ? (a) : (b))”.
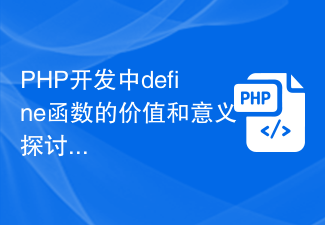
In PHP development, we often encounter situations where we need to define constants. In order to better manage constants and ensure their consistency and maintainability throughout the application, PHP provides the define function to define constants. This article will delve into the value and significance of the define function and provide specific code examples to help readers better understand. 1. Basic syntax and usage of define function In PHP, define function is used to define constants. Its basic syntax is as follows: define(name,
