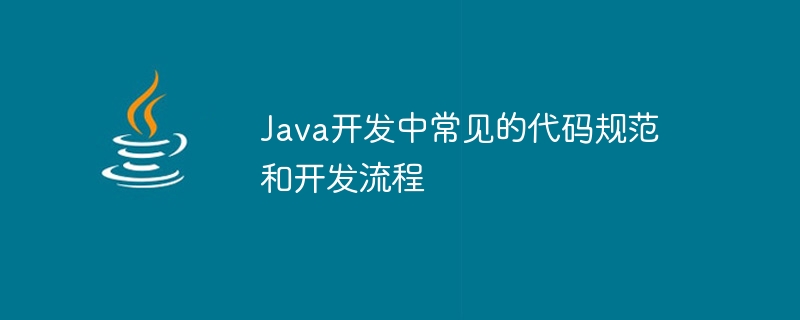
Java is a commonly used object-oriented programming language that is widely used in software development. In Java development, following certain code specifications and development processes can improve the maintainability of the code and the efficiency of team collaboration. This article will introduce some common Java code specifications and development processes, and give specific code examples.
1. Code specifications
- Naming specifications
In Java development, consistent naming specifications contribute to the readability and understandability of the code. Common naming conventions include:
(1) Class names and interface names use camel case naming starting with an uppercase letter, such as UserInfo
.
(2) Variable names, method names and parameter names use camel case naming starting with lowercase letters, such as userName
.
(3) Constant names are all in capital letters, and multiple words are separated by underscores, such as MAX_SIZE
.
- Coding style
Good coding style helps improve the readability of the code. In Java development, some common code style specifications include:
(1) Indentation and alignment: Use tabs or spaces to indent code and align it in a consistent manner.
(2) Code block wrapping: Follow the principle that curly braces occupy a separate line, so that the hierarchical structure of the code can be seen more clearly.
(3) Comments: Comment key code to help other developers understand the intent of the code.
2. Development process
- Requirements analysis and design
In Java development, requirements analysis and design are an important link. The development team needs to fully communicate with the demander, clarify the requirements, and formulate a reasonable design plan. For example, if the demander wants to develop a user management system, we can design the following classes and interfaces:
public interface UserService {
User getUserById(int id);
void saveUser(User user);
// ...
}
public class UserServiceImpl implements UserService {
@Override
public User getUserById(int id) {
// 根据id查找用户
}
@Override
public void saveUser(User user) {
// 保存用户信息
}
// ...
}
Copy after login
- Coding and unit testing
Before coding, we can design according to the requirements and , formulate detailed development plans and task assignments. In the coding stage, we can code according to the code specifications and write corresponding unit tests for each functional module. For example, we can write the following unit test code:
import org.junit.Test;
public class UserServiceTest {
private UserService userService = new UserServiceImpl();
@Test
public void testGetUserById() {
User user = userService.getUserById(1);
// 断言用户id为1
}
@Test
public void testSaveUser() {
User user = new User();
user.setId(1);
user.setName("Tom");
userService.saveUser(user);
// 断言保存成功
}
}
Copy after login
- Integration and system testing
After completing the coding and unit testing, we can integrate each functional module and perform System test. System testing can check whether the cooperation of various modules is normal and find potential problems. For example, we can write an integration test class:
import org.junit.Test;
public class SystemTest {
private UserService userService = new UserServiceImpl();
@Test
public void testGetUserById() {
User user = userService.getUserById(1);
// 断言用户id为1
}
@Test
public void testSaveUser() {
User user = new User();
user.setId(1);
user.setName("Tom");
userService.saveUser(user);
// 断言保存成功
}
}
Copy after login
- Online and maintenance
After passing the system test and fixing all problems, we can deploy the system to the production environment, and perform operation and maintenance. It can also be optimized and improved based on user feedback and new needs.
Summary:
In Java development, following certain code specifications and development processes can improve the maintainability of the code and the efficiency of team collaboration. Code specifications can make the code easy to read and understand, and the development process can make the development process orderly. A good code specification and development process are the cornerstones of successful Java development.
The above is the detailed content of Common code specifications and development processes in Java development. For more information, please follow other related articles on the PHP Chinese website!