datetime_replace = datetime.datetime(year=2022, month=7, day=9, hour=19, minute=14, second=27, microsecond=123)
print(datetime_replace)
ctime_datetime = datetime.datetime.ctime(datetime_replace)
print(ctime_datetime, type(ctime_datetime))
```
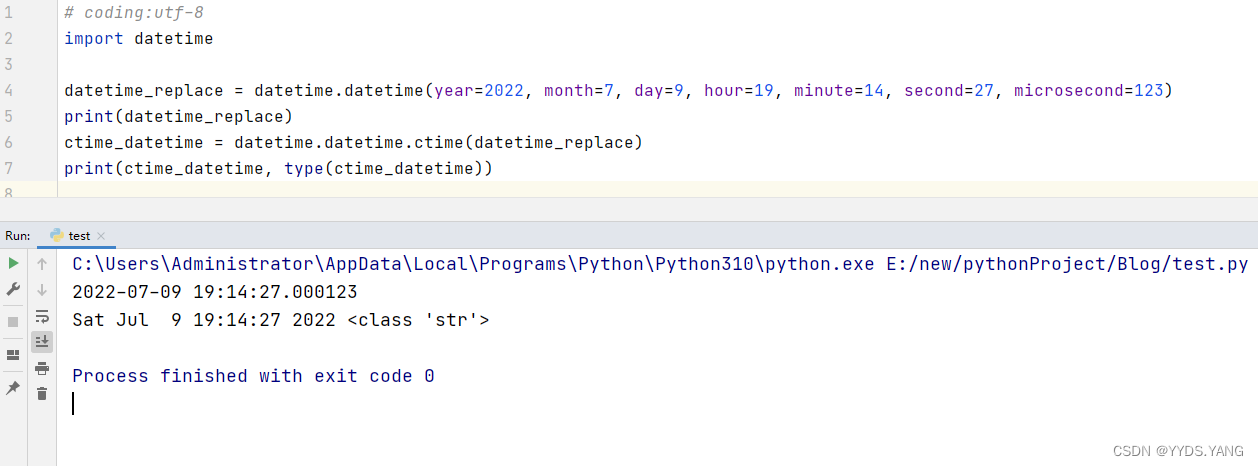
## 7、日期时间对象转换为时间戳
```python
datetime_timestamp = datetime.datetime.timestamp(datetime_replace)
print(datetime_timestamp, type(datetime_timestamp)) # 1657365267.000123
```
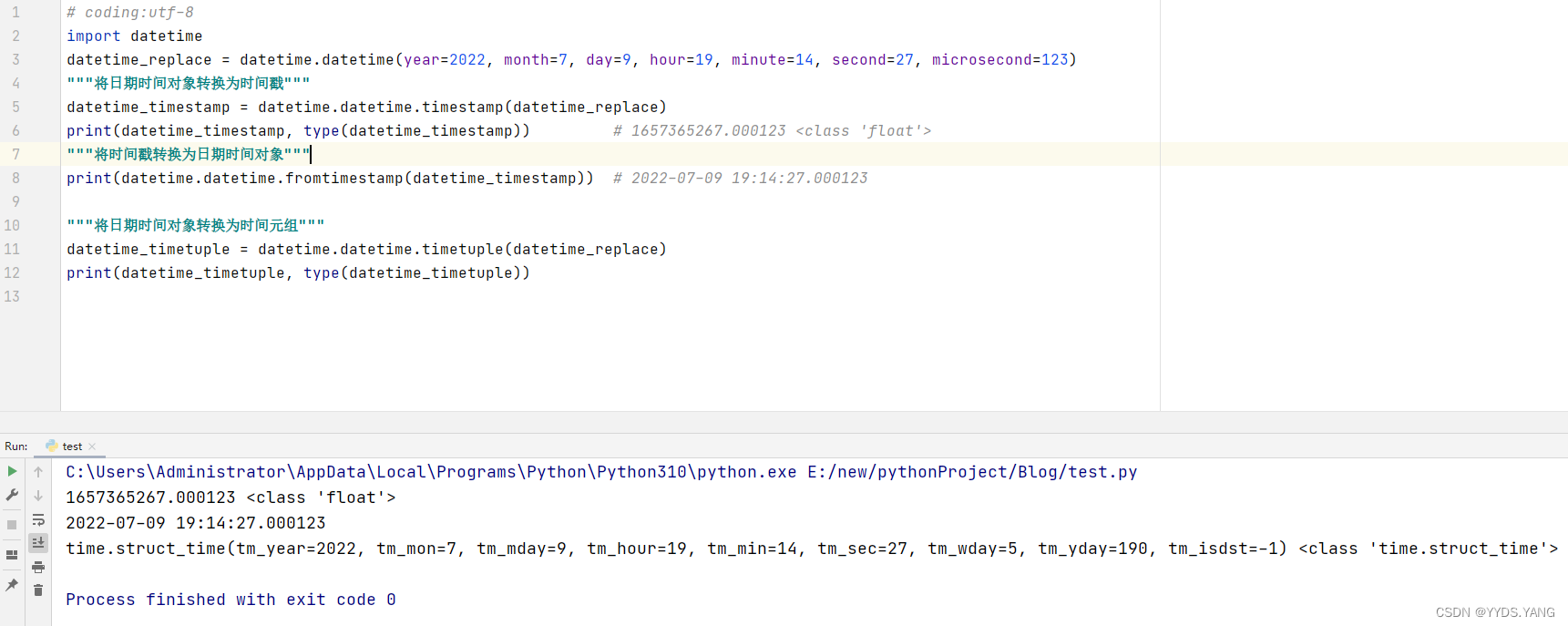
## 8、时间戳转换为日期时间对象
```python
print(datetime.datetime.fromtimestamp(datetime_timestamp)) # 2022-07-09 19:14:27.000123
```
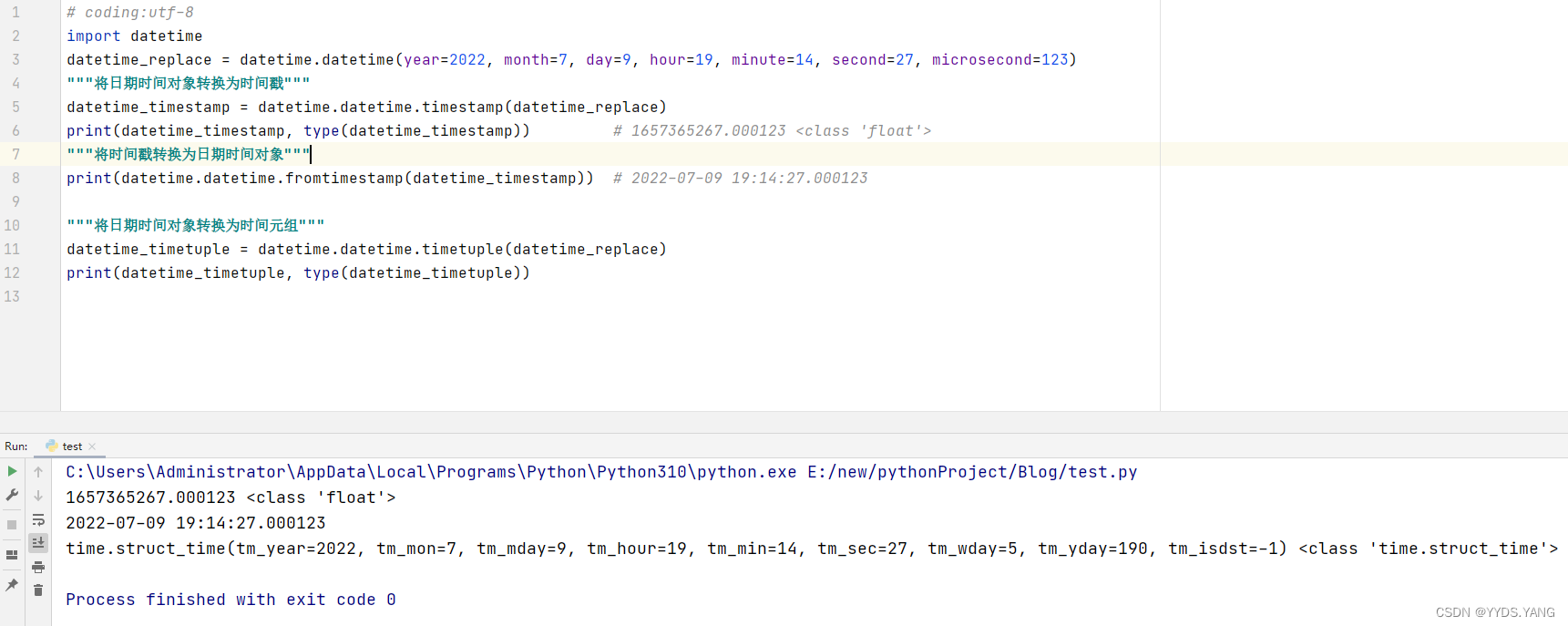
## 9、日期时间对象转换为时间元组
```python
datetime_timetuple = datetime.datetime.timetuple(datetime_replace)
print(datetime_timetuple, type(datetime_timetuple))
```
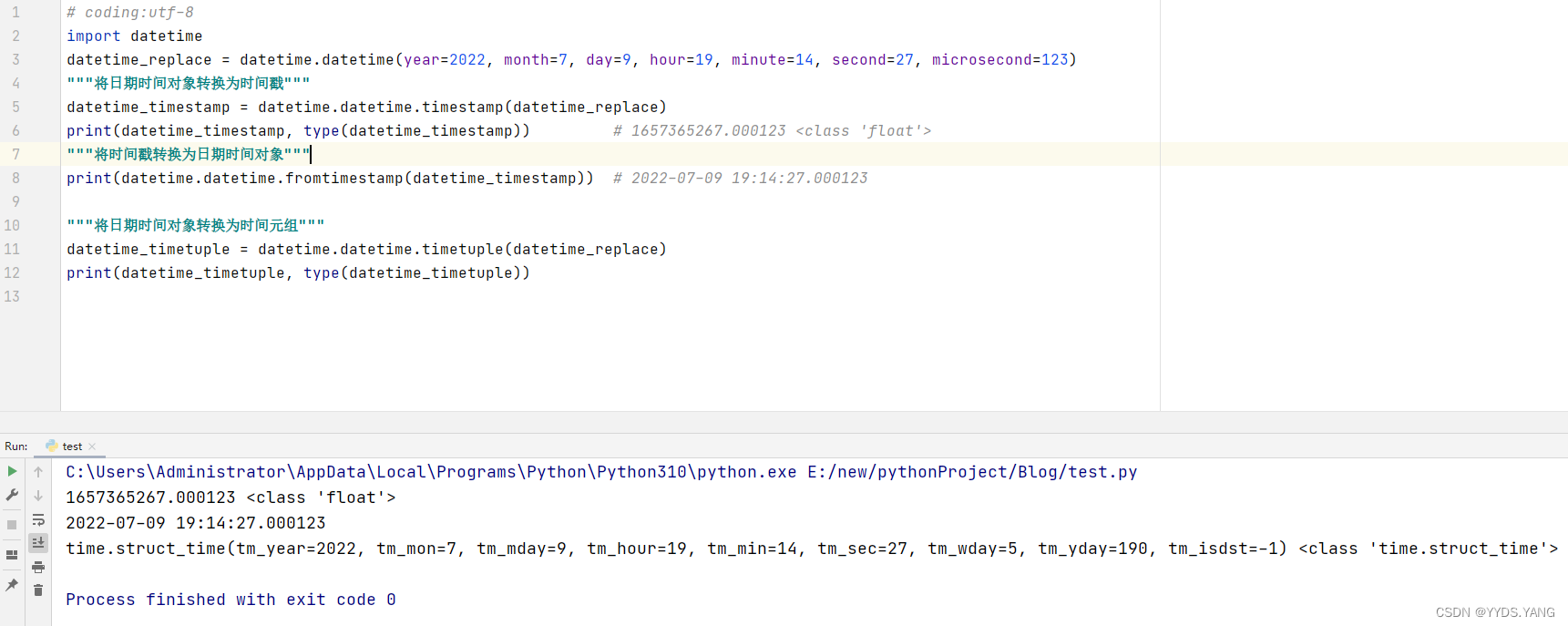
## 10、ISO标准日期时间格式
ISO标准的日历时间,Calendar中文释义为日历
* 各个值的含义为(年份、周数、周内的第N天)即(year, week, weekday);
* weekday的值为[1,7],1代表周一,7代表周日
* 示例:datetime.IsoCalendarDate(year=2022, week=27, weekday=7)
```python
datetime_replace = datetime.datetime(year=2022, month=7, day=9, hour=19, minute=14, second=27, microsecond=123)
UTCDateTime = datetime.datetime(year=2022, month=7, day=10, hour=19, minute=14, second=27, microsecond=1235)
# ISO标准日期时间格式
print(datetime.datetime.utcoffset(UTCDateTime))
# 将日期时间对象转换为ISO标准日期时间格式的字符串
UTC_ISO_DateTime = datetime.datetime.isoformat(UTCDateTime)
print(UTC_ISO_DateTime, type(UTC_ISO_DateTime)) # 2022-07-10T19:14:27.001235
# 将ISO标准日期时间格式的字符串转换为日期时间类型
From_UTC_ISO_DateTime = datetime.datetime.fromisoformat('9999-12-31T23:59:59.999999') #
print(From_UTC_ISO_DateTime, type(From_UTC_ISO_DateTime))
# ISO标准的周内第N天
# 值的范围是[1,7],1代表周一,7代表周日
UTC_ISO_WeekDateTime = datetime.datetime.isoweekday(UTCDateTime)
print(UTC_ISO_WeekDateTime, type(UTC_ISO_WeekDateTime)) # 7
# ISO标准的日历时间,Calendar中文释义为日历
# 各个值的含义为(年份、周数、周内的第N天)即(year, week, weekday);
# weekday的值为[1,7],1代表周一,7代表周日
# 示例:datetime.IsoCalendarDate(year=2022, week=27, weekday=7)
UTC_ISO_CalendarDateTime = datetime.datetime.isocalendar(UTCDateTime)
print(UTC_ISO_CalendarDateTime, type(UTC_ISO_CalendarDateTime))
# 将ISO标准日历格式的字符串转换为时间日期型
From_UTC_ISO_CalendarDateTime = datetime.datetime.fromisocalendar(year=2022, week=27, day=7)
print(From_UTC_ISO_CalendarDateTime) # 2022-07-10 00:00:00
print(type(From_UTC_ISO_CalendarDateTime)) #
```
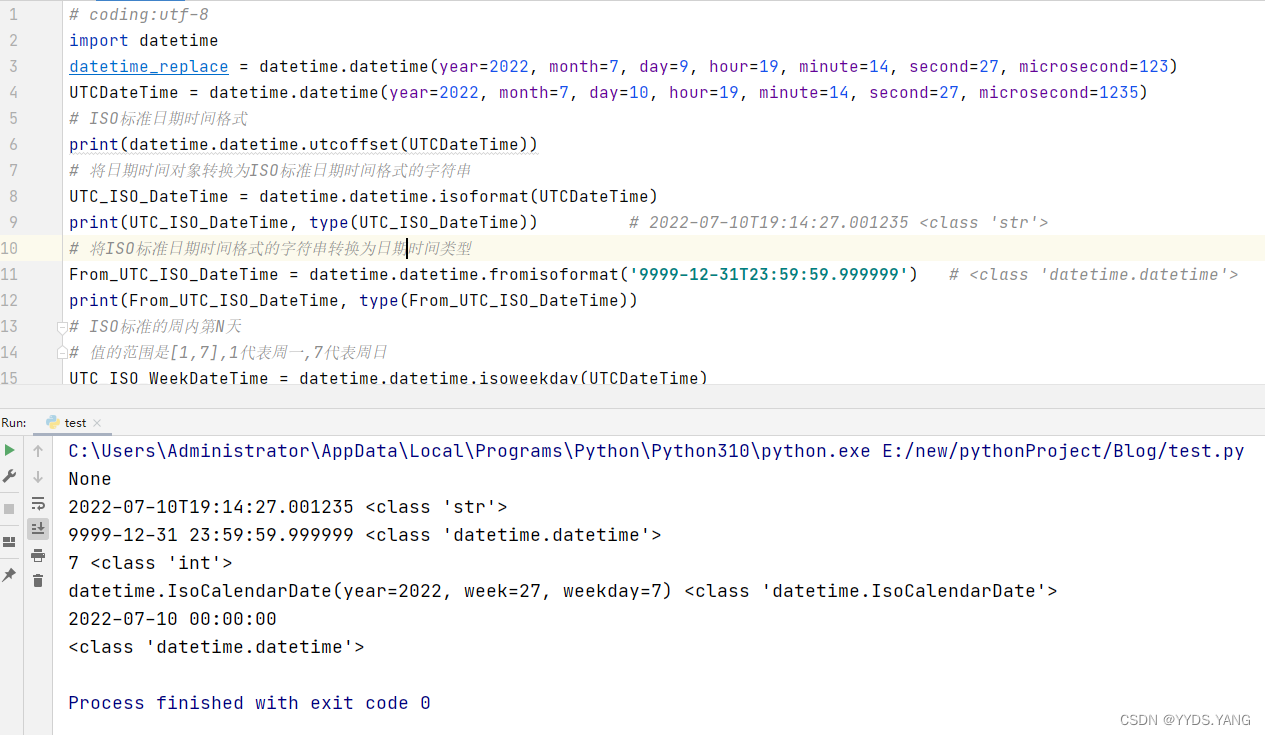
## 11、日期时间替换函数replace()
* replace()可以只替换日期时间属性的某一项
* replace()函数的第一个参数必传
* replace()函数的第一个参数是一个日期时间类型(datetime.datetime)的对象
* 按关键字传参替换
* 按位置传参体换
```python
datetime_replace = datetime.datetime(year=2022, month=7, day=9, hour=19, minute=14, second=27, microsecond=123)
# 初始值
print(f"datetime_replace的原值为:{datetime_replace}", f"类型是:{type(datetime_replace)}")
# 不传参数
print(datetime.datetime.replace(datetime_replace)) # 2022-07-09 19:14:27.000123
# 只替换年份
print(datetime.datetime.replace(datetime_replace, 2019)) # 2019-07-09 19:14:27.000123
print(datetime.datetime.replace(datetime_replace, year=2019)) # 2019-07-09 19:14:27.000123
# 只替换月份, 替换其他参数同理
print(datetime.datetime.replace(datetime_replace, month=12)) # 2022-12-09 19:14:27.000123
print(datetime.datetime.replace(datetime_replace, datetime_replace.year, 12)) # 2022-12-09 19:14:27.000123
# 替换其他参数同理
print(datetime.datetime.replace(datetime_replace, year=2019, month=12, day=31, hour=15,
minute=13, second=15, microsecond=9999)) # 2019-12-31 15:13:15.009999
```
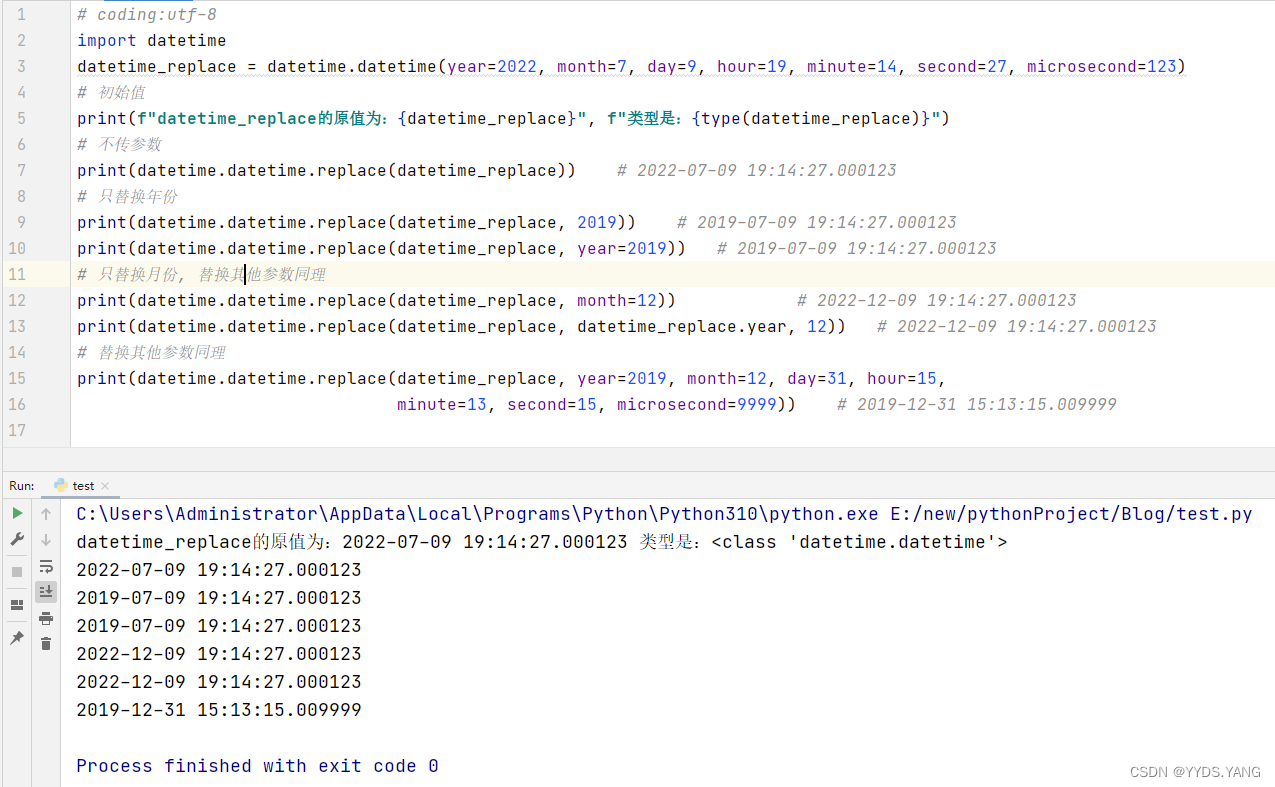
## 12、日期时间对象格式化strftime()
* 日期时间对象格式化常用的格式如下
* %H(两位数的小时)
* %M(两位数的分钟)
* %S(两位数的秒)
* %f(6位数的微秒)
* %h(简写的月份名,一般为英文简写)
* %y(两位数的年份)
* %Y(四位数的年份)
* %m(两位数的月份)
* %d(两位数的天数)
* 可以只格式化部分属性
```python
datetime_replace = datetime.datetime(year=2022, month=7, day=9, hour=19, minute=14, second=27, microsecond=123)
# 可以只格式化部分属性
datetime_str = datetime.datetime.strftime(datetime_replace, "%Y-%m-%d %H:%M:%S.%f")
print(f"格式化后是:{datetime_str}", type(datetime_str)) # 2022-07-09 19:14:27.000123
# 格式化日期属性
datetime_str_date = datetime.datetime.strftime(datetime_replace, "%Y-%m-%d")
print(f"格式化日期的值为:{datetime_str_date}") # 2022-07-09
# 格式时间属性
datetime_str_time = datetime.datetime.strftime(datetime_replace, "%H:%M:%S.%f")
print(f"格式化时间的值为:{datetime_str_time}") # 19:14:27.000123
```
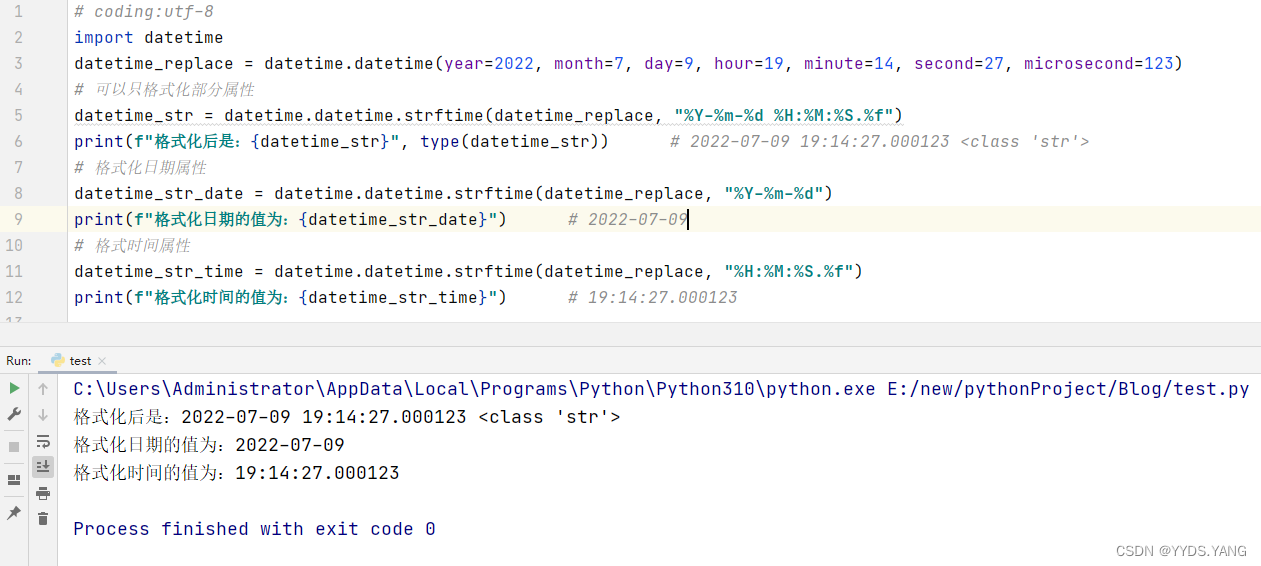
## 附录、完整代码
```python
# coding:utf-8
import datetime
# 日期时间对象
# 日期时间对象是指具有日期(年月日)和时间(时分秒)双重属性的实例
# 日期时间对象的类型为datetime.datetime
# 日期时间对象常用的属性有年、月、日、时、分、秒、微秒等
# 日期时间对象可以指定时间创建,也可以通过获取当前时间来创建
# 日期时间对象指定时间创建时可按位置传参创建,也可关键字传参创建
# 日期时间对象的创建函数有datetime.datetime(),datetime.datetime.now()、datetime.datetime.today()、datetime.datetime.utcnow()
# 日期时间对象通过datetime.datetime()创建时的参数依次为:year,month,day,hour,minute,second,microsecond
# 日期时间对象通过datetime.datetime.now()函数创建不需要参数
# 日期时间对象通过datetime.datetime.today()函数创建不需要参数
# 日期时间对象通过datetime.datetime.utcnow()函数创建不需要参数
# 日期时间对象通过datetime.datetime()创建时至少应该包含年月日三个参数
# 日期时间对象通过datetime.datetime()创建时的参数范围如下
# {year[1~9999]、month[1~12]、day[1~31]、hour[0~23]、minute[0~59]、second[0~59]、microsecond[1~999999]}
# 通过datetime.datetime.utcnow()创建
datetime_zero = datetime.datetime.utcnow()
# 通过datetime.datetime.today()函数创建
datetime_first = datetime.datetime.today()
# 通过datetime.datetime.now()创建
datetime_second = datetime.datetime.now()
# 通过datetime.datetime()函数指定日期时间、关键字传参创建
datetime_three = datetime.datetime(year=1, month=1, day=1, hour=0, minute=0, second=0, microsecond=1)
datetime_four = datetime.datetime(year=9999, month=12, day=31, hour=23, minute=59, second=59, microsecond=999999)
# 通过datetime.datetime()函数指定日期时间、按位置传参创建,顺序不可变且参数值必须在规定的范围内
datetime_five = datetime.datetime(9999, 12, 31, 23, 59, 59, 999999)
print(datetime_zero, type(datetime_zero)) # 2022-07-09 18:12:43.486469
print(datetime_first, type(datetime_first)) # 2022-07-09 18:12:43.486469
print(datetime_second, type(datetime_second)) # 2022-07-09 18:12:43.486469
print(datetime_three, type(datetime_three)) # 0001-01-01 00:00:00.000001
print(datetime_four, type(datetime_four)) # 9999-12-31 23:59:59.999999
print(datetime_five, type(datetime_five)) # 9999-12-31 23:59:59.999999
# 查看datetime可以处理的最大的日期时间对象及最小的日期时间对象
print(datetime.datetime.min) # 0001-01-01 00:00:00
print(datetime.datetime.max) # 9999-12-31 23:59:59.999999
"""# 从日期时间对象中获取日期属性【年-月-日】"""
new_time = datetime.datetime.date(datetime_first)
print(new_time)
print(type(new_time))
"""# 从日期时间对象中获取时间属性【时:分:秒:微秒】"""
new_time = datetime.datetime.time(datetime_first)
print(new_time)
print(type(new_time))
"""# 从日期时间对象中获取年份"""
datetime_year = datetime_four.year
print(datetime_year, type(datetime_year)) # 9999
"""# 从日期时间对象中获取月份"""
datetime_month = datetime_four.month
print(datetime_month, type(datetime_month)) # 12
"""# 从日期时间对象中获取天"""
datetime_day = datetime_four.day
print(datetime_day, type(datetime_day)) # 31
"""# 从日期时间对象中获取小时"""
datetime_hour = datetime_four.hour
print(datetime_hour, type(datetime_hour)) # 23
"""# 从日期时间对象中获取分钟"""
datetime_minute = datetime_four.minute
print(datetime_minute, type(datetime_minute)) # 59
"""# 从日期时间对象中获取秒数"""
datetime_second = datetime_four.second
print(datetime_second, type(datetime_second)) # 59
"""# 从日期时间对象中获取微秒"""
datetime_microsecond = datetime_four.microsecond
print(datetime_microsecond, type(datetime_microsecond)) # 999999
"""# datetime.datetime.date()函数的参数只能是datetime.datetime类型"""
date_time = datetime.date(2022, 12, 26)
"""# 传入的参数不能为datetime.date类型"""
"""# TypeError: descriptor 'date' for 'datetime.datetime' objects doesn't apply to a 'datetime.date' object"""
"""# print(datetime.datetime.date(date_time))"""
time_time = datetime.time(12, 2, 54, 999999)
"""# 传入的参数不能为datetime.time类型"""
"""# TypeError: descriptor 'date' for 'datetime.datetime' objects doesn't apply to a 'datetime.time' object"""
"""# print(datetime.datetime.date(time_time))"""
"""# 同理,datetime.datetime.time()函数传入的参数亦不能为datetime.date类型和datetime.time类型"""
"""# TypeError: descriptor 'time' for 'datetime.datetime' objects doesn't apply to a 'datetime.date' object"""
"""# print(datetime.datetime.time(date_time))"""
"""# TypeError: descriptor 'time' for 'datetime.datetime' objects doesn't apply to a 'datetime.time' object"""
"""# print(datetime.datetime.time(time_time))"""
# 将日期时间对象转换为时间元组类型
# 时间元组是指具有 年份、月份、日、小时、分钟、秒数、星期中的第N天、年中的第N天、夏令时标志的一个元组对象
# 时间元组示例:(tm_year=2022, tm_mon=7, tm_mday=9, tm_hour=19, tm_min=14, tm_sec=27, tm_wday=5, tm_yday=190, tm_isdst=0)
# 其中tm_wday的值从0开始,范围是:0~6,0为星期一,6为星期日;tm_isdst=0代表未启用夏令时
UTCDateTime = datetime.datetime(year=2022, month=7, day=10, hour=19, minute=14, second=27, microsecond=1235)
datetime_UTCTimeTuple = datetime.datetime.utctimetuple(UTCDateTime)
print(datetime_UTCTimeTuple, type(datetime_UTCTimeTuple)) # 类型为:
# 将日期时间对象转化为公元历开始计数的天数
datetime_replace = datetime.datetime(year=2022, month=7, day=9, hour=19, minute=14, second=27, microsecond=123)
datetime_ordinal = datetime.datetime.toordinal(datetime_replace)
print(datetime_ordinal, type(datetime_ordinal)) # 738345
print(datetime.datetime.fromordinal(1)) # 0001-01-02 00:00:00
print(datetime.datetime.fromordinal(2)) # 0001-01-02 00:00:00
# ctime()是将日期时间类型转换为一个日期之间值的字符串,示例如 Sat Jul 9 19:14:27 2022(2022年7月9日星期六)
# ctime()返回值的第一部分的值代表星期几,第二部分的值代表月份,第三部分的值代表日,第四部分的值代表时间,第五部分的值代表年份
print(datetime_replace)
ctime_datetime = datetime.datetime.ctime(datetime_replace)
print(ctime_datetime, type(ctime_datetime))
# 将日期时间对象转换为时间戳
datetime_timestamp = datetime.datetime.timestamp(datetime_replace)
print(datetime_timestamp, type(datetime_timestamp)) # 1657365267.000123
# 将时间戳转换为日期时间对象
print(datetime.datetime.fromtimestamp(datetime_timestamp)) # 2022-07-09 19:14:27.000123
# 将日期时间对象转换为时间元组
datetime_timetuple = datetime.datetime.timetuple(datetime_replace)
print(datetime_timetuple, type(datetime_timetuple))
# ISO标准日期时间格式
print(datetime.datetime.utcoffset(UTCDateTime))
# 将日期时间对象转换为ISO标准日期时间格式的字符串
UTC_ISO_DateTime = datetime.datetime.isoformat(UTCDateTime)
print(UTC_ISO_DateTime, type(UTC_ISO_DateTime)) # 2022-07-10T19:14:27.001235
# 将ISO标准日期时间格式的字符串转换为日期时间类型
From_UTC_ISO_DateTime = datetime.datetime.fromisoformat('9999-12-31T23:59:59.999999') #
print(From_UTC_ISO_DateTime, type(From_UTC_ISO_DateTime))
# ISO标准的周内第N天
# 值的范围是[1,7],1代表周一,7代表周日
UTC_ISO_WeekDateTime = datetime.datetime.isoweekday(UTCDateTime)
print(UTC_ISO_WeekDateTime, type(UTC_ISO_WeekDateTime)) # 7
# ISO标准的日历时间,Calendar中文释义为日历
# 各个值的含义为(年份、周数、周内的第N天)即(year, week, weekday);
# weekday的值为[1,7],1代表周一,7代表周日
# 示例:datetime.IsoCalendarDate(year=2022, week=27, weekday=7)
UTC_ISO_CalendarDateTime = datetime.datetime.isocalendar(UTCDateTime)
print(UTC_ISO_CalendarDateTime, type(UTC_ISO_CalendarDateTime))
# 将ISO标准日历格式的字符串转换为时间日期型
From_UTC_ISO_CalendarDateTime = datetime.datetime.fromisocalendar(year=2022, week=27, day=7)
print(From_UTC_ISO_CalendarDateTime) # 2022-07-10 00:00:00
print(type(From_UTC_ISO_CalendarDateTime)) #
# 日期时间替换函数replace()
# replace()可以只替换日期时间属性的某一项
# replace()函数的第一个参数必传
# replace()函数的第一个参数是一个日期时间类型(datetime.datetime)的对象
# 按关键字传参替换
# 按位置传参体换
datetime_replace = datetime.datetime(year=2022, month=7, day=9, hour=19, minute=14, second=27, microsecond=123)
# 初始值
print(f"datetime_replace的原值为:{datetime_replace}", f"类型是:{type(datetime_replace)}")
# 不传参数
print(datetime.datetime.replace(datetime_replace)) # 2022-07-09 19:14:27.000123
# 只替换年份
print(datetime.datetime.replace(datetime_replace, 2019)) # 2019-07-09 19:14:27.000123
print(datetime.datetime.replace(datetime_replace, year=2019)) # 2019-07-09 19:14:27.000123
# 只替换月份, 替换其他参数同理
print(datetime.datetime.replace(datetime_replace, month=12)) # 2022-12-09 19:14:27.000123
print(datetime.datetime.replace(datetime_replace, datetime_replace.year, 12)) # 2022-12-09 19:14:27.000123
# 替换其他参数同理
print(datetime.datetime.replace(datetime_replace, year=2019, month=12, day=31, hour=15,
minute=13, second=15, microsecond=9999)) # 2019-12-31 15:13:15.00999
# 日期时间对象格式化strftime()
# 日期时间对象格式化常用的格式如下:
""
%H(两位数的小时)、%M(两位数的分钟)、%S(两位数的秒)、%f(6位数的微秒)、%h(简写的月份名,一般为英文简写)
%y(两位数的年份)、%Y(四位数的年份)、%m(两位数的月份)、%d(两位数的天数)
"""
# 可以只格式化部分属性
datetime_str = datetime.datetime.strftime(datetime_replace, "%Y-%m-%d %H:%M:%S.%f")
print(f"格式化后是:{datetime_str}", type(datetime_str)) # 2022-07-09 19:14:27.000123
# 格式化日期属性
datetime_str_date = datetime.datetime.strftime(datetime_replace, "%Y-%m-%d")
print(f"格式化日期的值为:{datetime_str_date}") # 2022-07-09
# 格式时间属性
datetime_str_time = datetime.datetime.strftime(datetime_replace, "%H:%M:%S.%f")
print(f"格式化时间的值为:{datetime_str_time}") # 19:14:27.000123
```
Copy after login