A brief analysis of how to handle exceptions in Vue3 dynamic components
How to handle exceptions in Vue3 dynamic components? The following article will talk about Vue3 dynamic component exception handling methods. I hope it will be helpful to everyone!
[Related recommendations: vuejs video tutorial]
There are two common scenarios for dynamic components:
The first is dynamic routing:
// 动态路由 export const asyncRouterMap: Array<RouteRecordRaw> = [ { path: '/', name: 'index', meta: { title: '首页' }, component: BasicLayout, // 引用了 BasicLayout 组件 redirect: '/welcome', children: [ { path: 'welcome', name: 'Welcome', meta: { title: '引导页' }, component: () => import('@/views/welcome.vue') }, ... ] } ]
The second is dynamic rendering components, such as switching in Tabs:
<el-tabs :model-value="copyTabName" type="card"> <template v-for="item in tabList" :key="item.key || item.name"> <el-tab-pane :name="item.key" :label="item.name" :disabled="item.disabled" :lazy="item.lazy || true" > <template #label> <span> <component v-if="item.icon" :is="item.icon" /> {{ item.name }} </span> </template> // 关键在这里 <component :key="item.key || item.name" :is="item.component" v-bind="item.props" /> </el-tab-pane> </template> </el-tabs>
will not cause any other problems when used in vue2, but when you wrap the component into a responsive object, in vue3, a warning will appear:
Vue received a Component which was made a reactive object. This can lead to unnecessary performance overhead, and should be avoided by marking the component with markRaw
or using shallowRef
instead of ref
.
This warning appears because: Using reactive or ref (the same is true for declaration in the data function), declaring variables will act as a proxy, and our component has no other use after proxying , in order to save performance overhead, vue recommends that we use shallowRef or markRaw to skip the proxy proxy.
The solution is as mentioned above, you need to use shallowRef or markRaw for processing:
For Tabs processing:
import { markRaw, ref } from 'vue' import A from './components/A.vue' import B from './components/B.vue' interface ComponentList { name: string component: Component // ... } const tab = ref<ComponentList[]>([{ name: "组件 A", component: markRaw(A) }, { name: "组件 B", component: markRaw(B) }])
For dynamic routing processing:
import { markRaw } from 'vue' // 动态路由 export const asyncRouterMap: Array<RouteRecordRaw> = [ { path: '/', name: 'home', meta: { title: '首页' }, component: markRaw(BasicLayout), // 使用 markRaw // ... } ]
The difference between shallowRef and markRaw is that shallowRef will only respond to value modifications, such as:
const state = shallowRef({ count: 1 }) // 不会触发更改 state.value.count = 2 // 会触发更改 state.value = { count: 2 }
And markRaw marks an object as not being converted to a proxy. The object itself is then returned.
const foo = markRaw({}) console.log(isReactive(reactive(foo))) // false // 也适用于嵌套在其他响应性对象 const bar = reactive({ foo }) console.log(isReactive(bar.foo)) // false
It can be seen that the object processed by markRaw is no longer a responsive object.
For a component, it should not be a responsive object. When processing, there are two APIs, shallowRef and markRaw. It is recommended to use markRaw for processing.
(Learning video sharing: web front-end development, Basic programming video)
The above is the detailed content of A brief analysis of how to handle exceptions in Vue3 dynamic components. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
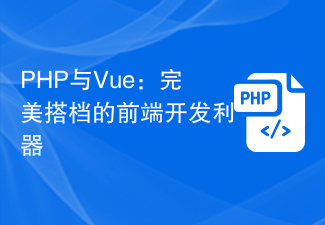
PHP and Vue: a perfect pairing of front-end development tools. In today's era of rapid development of the Internet, front-end development has become increasingly important. As users have higher and higher requirements for the experience of websites and applications, front-end developers need to use more efficient and flexible tools to create responsive and interactive interfaces. As two important technologies in the field of front-end development, PHP and Vue.js can be regarded as perfect tools when paired together. This article will explore the combination of PHP and Vue, as well as detailed code examples to help readers better understand and apply these two
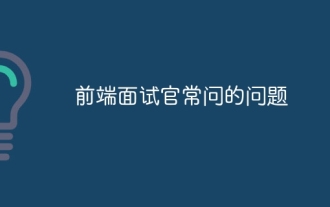
In front-end development interviews, common questions cover a wide range of topics, including HTML/CSS basics, JavaScript basics, frameworks and libraries, project experience, algorithms and data structures, performance optimization, cross-domain requests, front-end engineering, design patterns, and new technologies and trends. . Interviewer questions are designed to assess the candidate's technical skills, project experience, and understanding of industry trends. Therefore, candidates should be fully prepared in these areas to demonstrate their abilities and expertise.
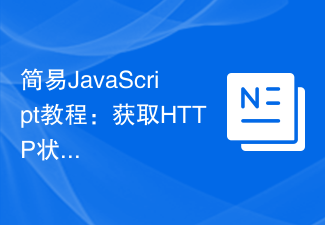
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
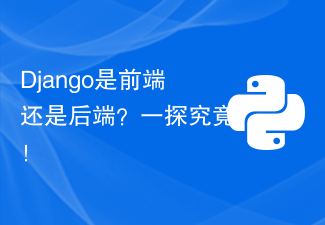
Django is a web application framework written in Python that emphasizes rapid development and clean methods. Although Django is a web framework, to answer the question whether Django is a front-end or a back-end, you need to have a deep understanding of the concepts of front-end and back-end. The front end refers to the interface that users directly interact with, and the back end refers to server-side programs. They interact with data through the HTTP protocol. When the front-end and back-end are separated, the front-end and back-end programs can be developed independently to implement business logic and interactive effects respectively, and data exchange.
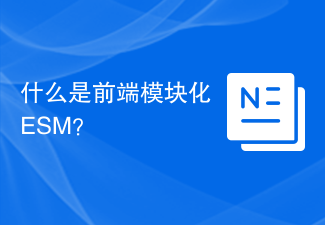
What is front-end ESM? Specific code examples are required. In front-end development, ESM refers to ECMAScriptModules, a modular development method based on the ECMAScript specification. ESM brings many benefits, such as better code organization, isolation between modules, and reusability. This article will introduce the basic concepts and usage of ESM and provide some specific code examples. The basic concept of ESM In ESM, we can divide the code into multiple modules, and each module exposes some interfaces for other modules to
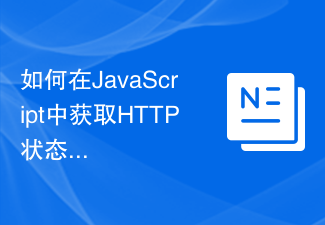
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
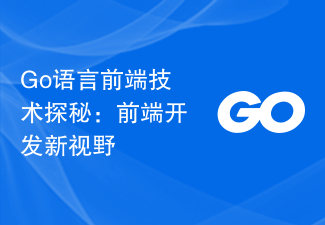
As a fast and efficient programming language, Go language is widely popular in the field of back-end development. However, few people associate Go language with front-end development. In fact, using Go language for front-end development can not only improve efficiency, but also bring new horizons to developers. This article will explore the possibility of using the Go language for front-end development and provide specific code examples to help readers better understand this area. In traditional front-end development, JavaScript, HTML, and CSS are often used to build user interfaces
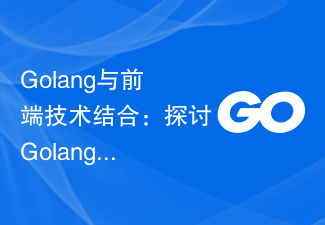
Combination of Golang and front-end technology: To explore how Golang plays a role in the front-end field, specific code examples are needed. With the rapid development of the Internet and mobile applications, front-end technology has become increasingly important. In this field, Golang, as a powerful back-end programming language, can also play an important role. This article will explore how Golang is combined with front-end technology and demonstrate its potential in the front-end field through specific code examples. The role of Golang in the front-end field is as an efficient, concise and easy-to-learn
