


Tutorial on how to represent the greatest common divisor in C language functions
Methods to efficiently and elegantly find the greatest common divisor in C language: use phase division to solve by constantly dividing the remainder until the remainder is 0. Two implementation methods are provided: recursion and iteration are concise and clear, and the iterative implementation is higher and more stable. Pay attention to handling negative numbers and 0 cases and consider performance optimization, but the phase division itself is efficient enough.
How to elegantly find the greatest common divisor in C language?
You may think that finding the greatest common divisor (GCD) is a small matter, and one line of code can be done? Indeed, it can be achieved with a loop, but that efficiency... tsk. In this article, let’s not play with those fancy ones, go straight to the topic and see how to write efficient and elegant GCD functions in C language. After reading it, you can not only write the code, but also understand the mathematical principles and optimization techniques behind it, and even improve it yourself.
Let’s talk about the conclusion first, we need to use the Euclidean algorithm. Why not use other methods? Because this thing is efficient, the algorithm is concise, and the code is also good-looking. Those stupid methods have many cycles and poor performance, which makes them difficult to watch.
Let’s review the basics first. To put it bluntly, the greatest common divisor is the largest integer that can divise two numbers at the same time. For example, the greatest common divisors of 12 and 18 are 6. How does phase division work? Simply put, it is to constantly divide a larger number by a smaller number and take the remainder until the remainder is 0. The divisor of the last division is the greatest common divisor.
Let’s look at the code, I try to write it concisely and easily understand:
<code class="c">int gcd(int a, int b) { // 确保a >= b,方便处理if (a </code>
The core of this code is to call gcd(b, a % b)
recursively. Each time the parameters a
and b
are changing, a
becomes the previous b
and b
becomes the previous remainder a % b
. Until b
becomes 0, recursively ends, and a
is returned as the result.
Some people may think recursion is not good, and the risk of stack overflow is high. This is indeed a problem, especially when the input number is very large. What should I do? Iterative version to save the scene:
<code class="c">int gcd_iterative(int a, int b) { while (b != 0) { int temp = b; b = a % b; a = temp; } return a; }</code>
This iterative version uses while
loop to implement the same function, avoiding recursive calls, which is more efficient and more stable. The code is also very concise and easy to understand.
Next, let’s talk about some common questions. For example, what should I do if the input is a negative number? If this situation is not handled in the code, it may cause an error to run directly. The solution is very simple. Add judgment at the beginning of the function and take the absolute value. Or, a more elegant approach is to have the function handle only non-negative integers and preprocess the input before calling the function.
There is another question that is easy to ignore: What happens to the function if the input is 0? Take a closer look at the iterative version. When a
or b
is 0, the loop ends immediately, returning another number. This fits the mathematical definition, but if your program has special requirements for 0, additional processing is required.
Finally, regarding performance optimization, phase division is actually efficient enough. There is no need to over-optimize unless you are dealing with astronomical numbers. At this time, you may need to consider more advanced algorithms, or use multi-precision arithmetic library. However, for most application scenarios, these two functions are sufficient. Remember that the readability and maintainability of the code are also important, and don't sacrifice the simplicity and understanding of the code in order to pursue extreme performance.
The above is the detailed content of Tutorial on how to represent the greatest common divisor in C language functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
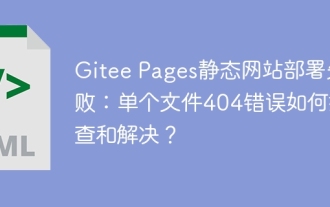
GiteePages static website deployment failed: 404 error troubleshooting and resolution when using Gitee...
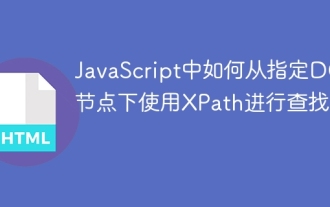
Detailed explanation of XPath search method under DOM nodes In JavaScript, we often need to find specific nodes from the DOM tree based on XPath expressions. If you need to...
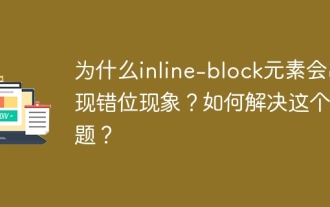
Regarding the reasons and solutions for misaligned display of inline-block elements. When writing web page layout, we often encounter some seemingly strange display problems. Compare...
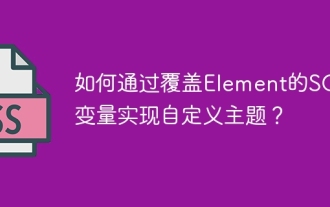
How to implement a custom theme by overriding the SCSS variable of Element? Using Element...
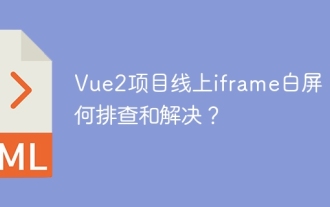
Troubleshooting and solving the online white screen of iframe in Vue2 project. In the development of Vue2 project, we often use iframes to embed other web content. However, the item...
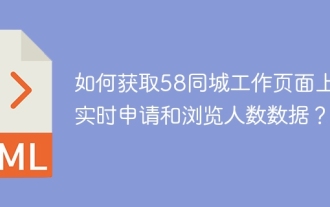
How to obtain dynamic data of 58.com work page while crawling? When crawling a work page of 58.com using crawler tools, you may encounter this...
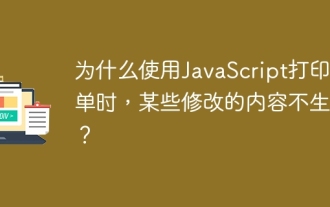
Why do some modified contents not take effect when printing forms using JavaScript? When printing forms using JavaScript, you may encounter such problems: �...
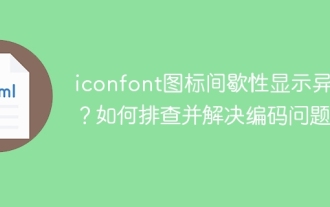
iconfont...
