


How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
JPA (Java Persistence API) is a Java specification for managing relational data in Java applications. It is used for object-relational mapping, which means it maps Java objects to database tables, facilitating data persistence and retrieval. To use JPA with advanced features like caching and lazy loading, follow these steps:
-
Setting up JPA in Your Project:
- Start by including a JPA implementation (like Hibernate or EclipseLink) in your project's dependencies. For example, with Maven, you would add dependencies for the JPA API and your chosen implementation.
- Configure the
persistence.xml
file, which specifies the JPA configuration details such as the data source, transaction type, and any additional properties required by your implementation.
-
Entity Mapping:
- Define your entities using Java classes annotated with JPA annotations (
@Entity
,@Table
,@Id
, etc.) to represent your database tables. - Use
@OneToMany
,@ManyToOne
,@ManyToMany
, and other annotations to define relationships between entities.
- Define your entities using Java classes annotated with JPA annotations (
-
Enabling Caching:
- To use caching, configure it in your
persistence.xml
. You might specify a second-level cache strategy to cache entity data across sessions. - Use annotations like
@Cacheable(true)
on your entities to indicate which entities should be cached.
- To use caching, configure it in your
-
Implementing Lazy Loading:
- Use the
fetch
attribute on relationship annotations (e.g.,@OneToMany(fetch = FetchType.LAZY)
) to specify lazy loading for related entities. - When you query data, JPA will initially load only the primary data. Related data will be loaded on-demand when you access the relationship.
- Use the
-
Using JPA in Your Application:
- Create an
EntityManagerFactory
to manageEntityManager
instances, which are used to interact with the database. - Use
EntityManager
methods likefind()
,persist()
,merge()
, andremove()
to perform CRUD operations.
- Create an
By carefully configuring these elements, you can leverage JPA's capabilities, including advanced features like caching and lazy loading, to enhance the performance and efficiency of your application.
What are the best practices for implementing caching in JPA to improve application performance?
Implementing caching effectively can significantly enhance application performance by reducing database load and improving data access times. Here are some best practices:
-
Use Second-Level Caching:
- Enable a second-level cache, which stores data across multiple sessions. This is especially beneficial for read-heavy applications where data changes infrequently.
- Configure the second-level cache in
persistence.xml
or through annotations.
-
Selectively Apply Caching:
- Not all data benefits from caching. Apply caching to entities that are frequently read but seldom updated. Use
@Cacheable(false)
to disable caching for entities where it might cause more harm than good.
- Not all data benefits from caching. Apply caching to entities that are frequently read but seldom updated. Use
-
Fine-Tune Cache Configuration:
- Adjust cache settings such as eviction policies (e.g., LRU, FIFO) and cache sizes to match your application's needs.
- Monitor cache hit and miss ratios to optimize cache performance.
-
Cache Concurrency Strategy:
- Choose an appropriate concurrency strategy (e.g., READ_ONLY, READ_WRITE, NONSTRICT_READ_WRITE) based on how often data changes and the consistency requirements of your application.
-
Invalidate Cache Appropriately:
- Set up mechanisms to clear or refresh the cache when data changes. This can be done manually or through event listeners triggered by entity changes.
-
Avoid Over-Caching:
- Be cautious about caching large data sets or infrequently accessed data, as this can consume memory and degrade performance.
By following these practices, you can maximize the benefits of caching while minimizing potential drawbacks.
How can lazy loading be effectively utilized in JPA to optimize data retrieval?
Lazy loading is a technique that defers the loading of related data until it is explicitly requested, thus improving initial data retrieval times. Here are ways to effectively use lazy loading in JPA:
-
Specify Lazy Loading in Mappings:
- Use the
fetch
attribute in relationship annotations to specify lazy loading. For example,@OneToMany(fetch = FetchType.LAZY)
.
- Use the
-
Use Proxies:
- JPA creates proxy objects for lazily loaded relationships. Accessing these objects triggers the loading of the related data.
-
Optimize Query Performance:
- Initial queries will be faster as they won't include related data, but remember that subsequent accesses might involve additional database calls.
-
Use Fetch Joins Strategically:
- For specific use cases where you know you'll need related data, use fetch joins to load related entities eagerly in a single query, e.g.,
SELECT e FROM Employee e JOIN FETCH e.department
.
- For specific use cases where you know you'll need related data, use fetch joins to load related entities eagerly in a single query, e.g.,
-
Avoid N 1 Select Problem:
- Be cautious of the N 1 select problem where accessing lazy-loaded collections results in a separate query for each item. Use techniques like batch fetching or join fetching to mitigate this.
-
Handling Lazy Initialization Exceptions:
- Be aware of lazy initialization exceptions that occur when trying to access lazy-loaded data outside of a transaction. Use techniques like
@Transactional
or fetch strategies to manage this.
- Be aware of lazy initialization exceptions that occur when trying to access lazy-loaded data outside of a transaction. Use techniques like
By effectively utilizing lazy loading, you can significantly improve the initial load times of your application while allowing for more granular control over data retrieval.
What are the potential pitfalls to watch out for when using advanced JPA features like caching and lazy loading?
While advanced JPA features like caching and lazy loading can enhance performance, they also come with potential pitfalls to watch out for:
-
Cache Inconsistency:
- Caching can lead to stale data if not properly managed. Changes to data might not be immediately reflected in the cache, leading to inconsistencies.
-
Memory Overhead:
- Caching can consume significant memory, especially if not optimized. Over-caching can lead to out-of-memory errors.
-
Lazy Initialization Exceptions:
- Lazy loading can cause exceptions if you attempt to access lazy-loaded properties outside of a transactional context (e.g., in a view layer).
-
N 1 Select Problem:
- When accessing lazy-loaded collections, you might end up with numerous additional queries (N 1), severely impacting performance.
-
Complex Configuration:
- Advanced JPA features often require nuanced configuration, which can be difficult to set up and maintain.
-
Performance Tuning Challenges:
- Optimizing caching and lazy loading strategies requires careful performance monitoring and tuning, which can be time-consuming.
-
Transaction Management:
- Ensuring proper transaction management is crucial, as both caching and lazy loading behaviors can depend heavily on transaction boundaries.
By understanding these potential pitfalls, you can take steps to mitigate their impact and leverage these advanced features effectively in your JPA-based applications.
The above is the detailed content of How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


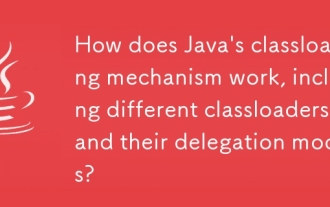
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
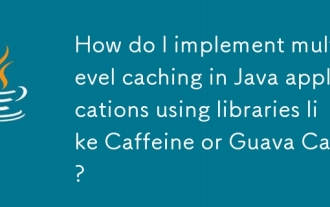
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
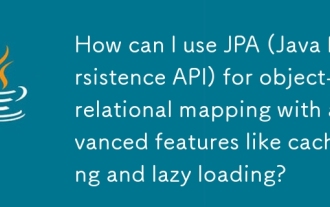
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
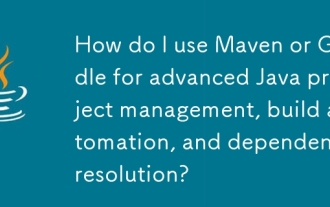
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
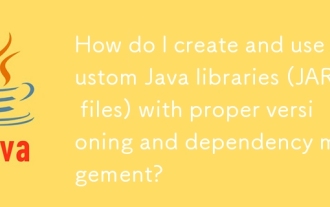
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
