This article details building a high-performance API gateway using Swoole. It emphasizes Swoole's asynchronous architecture for efficient request handling and discusses framework choices (Swoft/Hyperf), routing, backend communication, and crucial as
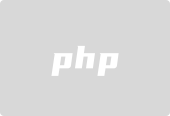
How to Build a High-Performance API Gateway Using Swoole?
Building a high-performance API gateway using Swoole involves leveraging its asynchronous, event-driven architecture to handle a large number of concurrent requests efficiently. Here's a step-by-step guide:
-
Choose a Framework (Optional but Recommended): While you can build directly with Swoole's core libraries, using a framework like Swoft or Hyperf can significantly simplify development and provide structure. These frameworks offer features like dependency injection, routing, and middleware management, making your code more maintainable and scalable.
-
Define Your API Routes: Determine how your gateway will route incoming requests to different backend services. This usually involves mapping URLs or specific request attributes to target services. Your chosen framework will provide mechanisms for defining these routes.
-
Implement Request Handling: Use Swoole's asynchronous capabilities to handle incoming requests. Instead of blocking on a single request, your gateway can concurrently process multiple requests. This involves using Swoole's
Server
class and its event handlers (e.g., onReceive
, onRequest
).
-
Backend Service Communication: Connect to your backend services using asynchronous HTTP clients provided by Swoole (e.g.,
Swoole\Coroutine\Http\Client
). This allows the gateway to concurrently fetch data from multiple services without blocking.
-
Response Aggregation and Transformation (if needed): If your gateway needs to aggregate data from multiple backend services or transform the response before sending it to the client, implement this logic within your request handlers.
-
Error Handling and Logging: Implement robust error handling and logging mechanisms to monitor the gateway's health and identify potential issues. Swoole provides tools for logging and exception handling.
-
Deployment and Monitoring: Deploy your gateway to a suitable environment (e.g., using Docker containers). Monitor key metrics such as request latency, throughput, and error rates to ensure optimal performance. Tools like Prometheus and Grafana can be useful for monitoring.
An example using Swoole's core (without a framework, for illustration):
$server = new Swoole\Http\Server("0.0.0.0", 9501);
$server->on('request', function (Swoole\Http\Request $request, Swoole\Http\Response $response) {
// Route the request based on the URL or other criteria
// ...
// Use Swoole\Coroutine\Http\Client to communicate with backend services
// ...
// Aggregate and transform responses (if needed)
// ...
$response->end("Response from API Gateway");
});
$server->start();
Copy after login
What are the key performance advantages of using Swoole for building an API gateway?
Swoole offers several key performance advantages for building API gateways:
- Asynchronous I/O: Swoole's asynchronous, event-driven architecture allows it to handle a large number of concurrent requests without blocking. This significantly improves throughput and reduces latency compared to traditional synchronous models.
- Coroutine Support: Swoole's coroutines enable writing asynchronous code that looks and feels like synchronous code, making it easier to develop and maintain high-performance applications. This simplifies complex asynchronous operations.
- High Concurrency: Swoole can handle tens of thousands of concurrent connections, making it suitable for high-traffic API gateways.
- Lightweight: Swoole is a relatively lightweight framework compared to some other solutions, consuming fewer system resources.
- Native Performance: Swoole is written in C, providing excellent performance and efficiency.
What are some common challenges encountered when building a high-performance API gateway with Swoole, and how can they be addressed?
Building a high-performance API gateway with Swoole presents some challenges:
- Complexity: While Swoole simplifies asynchronous programming, building a robust and scalable gateway still requires careful design and implementation. Using a framework can mitigate this.
- Debugging: Debugging asynchronous code can be more challenging than debugging synchronous code. Thorough logging and monitoring are crucial.
- Error Handling: Handling errors gracefully in an asynchronous environment is essential to prevent cascading failures. Implement robust error handling and fallback mechanisms.
- Scalability: As traffic increases, you'll need to scale your gateway horizontally (adding more servers). Employ load balancing techniques to distribute traffic effectively.
- Security: Protecting your API gateway from attacks is critical. Implement appropriate security measures such as input validation, authentication, and authorization.
Addressing these challenges:
- Use a framework: Frameworks like Swoft or Hyperf provide structure and tools to simplify development and debugging.
- Comprehensive logging and monitoring: Track key metrics and errors to identify and resolve issues quickly.
- Robust error handling: Implement proper exception handling and fallback mechanisms to prevent service disruptions.
- Horizontal scaling: Utilize load balancers and distribute traffic across multiple gateway instances.
- Security best practices: Employ strong authentication, authorization, and input validation techniques.
How can I integrate authentication and authorization mechanisms into a Swoole-based API gateway?
Integrating authentication and authorization into a Swoole-based API gateway can be achieved using several methods:
- API Keys: Generate unique API keys for each client and verify them in your gateway's request handlers. This is a simple method but less secure than others.
- OAuth 2.0: Implement an OAuth 2.0 server or use a third-party library to handle OAuth 2.0 flows. This provides robust authentication and authorization capabilities.
- JWT (JSON Web Tokens): Use JWTs to authenticate and authorize clients. JWTs are compact, self-contained tokens that can be easily verified by the gateway.
- Custom Authentication Schemes: You can implement your own custom authentication schemes based on your specific security requirements.
Implementation example (using JWT with a hypothetical JWT library):
// ... within your Swoole request handler ...
use Jwt\Jwt; // Hypothetical JWT library
$token = $request->header['Authorization']; // Assuming token is sent in Authorization header
try {
$payload = Jwt::verify($token, $yourSecretKey); // Verify JWT
$userId = $payload['user_id']; // Get user ID from payload
// Perform authorization checks based on user ID
// ...
// Proceed with request handling if authenticated and authorized
// ...
} catch (Exception $e) {
$response->status(401); // Unauthorized
$response->end("Unauthorized");
}
Copy after login
Remember to choose the authentication and authorization method that best suits your security requirements and integrate it securely within your Swoole-based API gateway. Always store your secrets securely (e.g., using environment variables) and avoid hardcoding them directly into your code.
The above is the detailed content of How to Build a High-Performance API Gateway Using Swoole?. For more information, please follow other related articles on the PHP Chinese website!