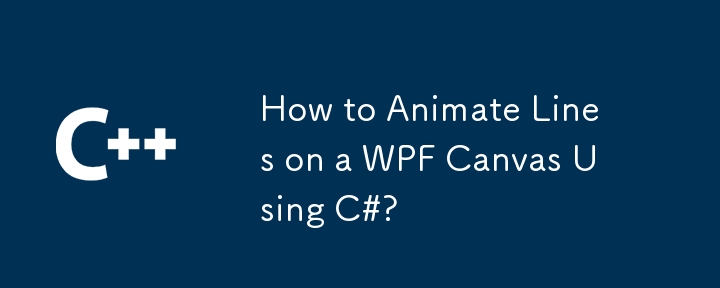
How to Create Animated Lines on a Canvas in C#
In a C#/WPF project, you can animate lines on a canvas by using system timers to update the line coordinates periodically.
To achieve this:
- Define a LineViewModel class that implements the INotifyPropertyChanged interface. This class will hold the line's coordinates, animation speed, and other properties.
- Create a ListBox with a Canvas as its ItemsPanel. Each item in the ListBox will represent a line.
- In the ListBox.ItemContainerStyle, define a ControlTemplate for the ListBoxItem. This template will include a Line element with its X1, Y1, X2, Y2, Thickness, Stroke, and Opacity properties bound to the corresponding properties in the LineViewModel.
- Initialize the LineViewModel with suitable initial coordinates and other properties. Add it as the DataContext for the ListBoxItem.
- Use a Timer within the LineViewModel to periodically adjust the X1, Y1, X2, and Y2 properties, effectively animating the line's position on the canvas.
- Provide controls to allow the user to adjust the animation speed. This can be achieved by modifying the AnimationSpeed property in the LineViewModel, which in turn affects the timer's interval.
- Bind the Animate property in the LineViewModel to a ToggleButton to toggle the animation on/off.
By implementing these steps, you can create an interactive animation where lines move across the canvas in a controlled and customizable manner.
The above is the detailed content of How to Animate Lines on a WPF Canvas Using C#?. For more information, please follow other related articles on the PHP Chinese website!