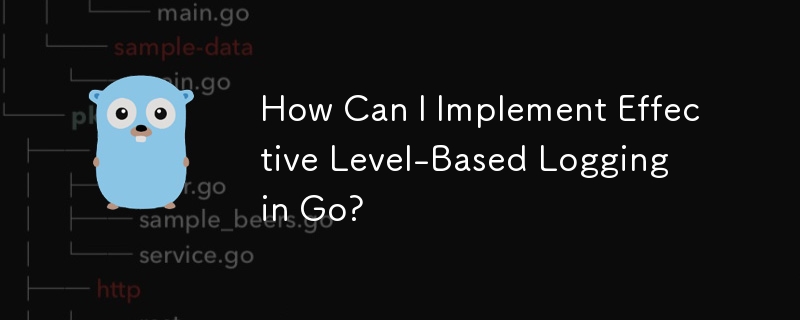
Level-Based Logging in Go: A Comprehensive Guide
In Go, logging is crucial for tracing application behavior and troubleshooting issues. Implementing level-based logging enables developers to configure the granularity of log messages displayed on the stdout and saved to a log file.
External Logging Wrappers
While Go's standard "log" package provides basic logging capabilities, consider utilizing external wrappers for more advanced features such as level-based logging. Here are some recommendations:
-
go-logging: Lightweight and customizable, with support for multiple logging levels, file sinks, and formatter configuration.
-
logrus: Widely adopted, with flexible logging levels, structured messages, and optional JSON output format.
-
log15: Designed for structured logging, simplifying log analysis and aggregation tasks.
-
glog: Google's official Go implementation of its C logging library, offering a familiar interface and extensive functionality.
-
kit/log: Emphasizes structured logging, enabling easy parsing and querying of log messages.
-
zap: High-performance and extensible, featuring fast logging and customizable formatters.
-
zerolog: Focused on zero-allocation JSON logging for performance-critical applications.
Implementing a Custom Wrapper
If you prefer to implement your own level-based logging wrapper, consider the following steps:
- Create a logger interface with methods for different logging levels (e.g., Error(), Info(), Debug()).
- Implement a concrete logger struct that handles log messages based on the provided logging level.
- Configure the logger with a log level specified as a command-line argument or from a configuration file.
- Initialize the logger and use it within your application to write messages to stdout and a log file (using io.Writer interface).
Remember to handle log message formatting, file rotation, and error handling appropriately to ensure reliable and comprehensive logging capabilities.
The above is the detailed content of How Can I Implement Effective Level-Based Logging in Go?. For more information, please follow other related articles on the PHP Chinese website!