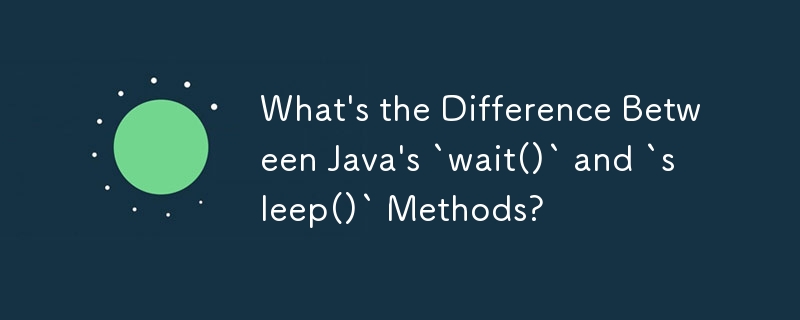
Understanding the Distinction Between "wait()" and "sleep()" in Java
Threads in Java have two crucial methods for thread synchronization: "wait()" and "sleep()." Recognizing the differences between these methods is essential for effectively managing thread execution.
Key Differences
-
Resource Control: "wait()" releases the lock on the current object, making the thread eligible to be awakened by another thread's call to "notify()." "sleep()", on the other hand, retains the thread's lock on the object.
-
CPU Consumption: "wait()" allows the thread to enter a dormant state without consuming CPU cycles, making it more energy-efficient. In contrast, "sleep()" consumes CPU cycles during the specified sleep duration.
Why Both Methods?
Java provides both "wait()" and "sleep()" to cater to specific use cases:
-
"wait()": Used when a thread needs to wait for an event or condition to occur. It releases the lock on the object so that other threads can proceed.
-
"sleep()": Used when a thread needs to temporarily pause its execution for a specified duration. It maintains the lock on the object, ensuring thread safety but consuming CPU resources.
Implementation Details
At the implementation level, "wait()" and "sleep()" have different mechanisms:
-
"wait()": Invokes the Object's "wait()" method, which puts the thread in a waiting pool associated with the object.
-
"sleep()": Utilizes static methods in the Thread class, including "sleep(long millis)," which pauses the thread for the specified number of milliseconds.
Best Practices
To avoid spurious wakeups from "wait()", it's recommended to use a conditional waiting technique:
synchronized (mon) {
while (!condition) {
mon.wait();
}
}
Copy after login
The above is the detailed content of What's the Difference Between Java's `wait()` and `sleep()` Methods?. For more information, please follow other related articles on the PHP Chinese website!