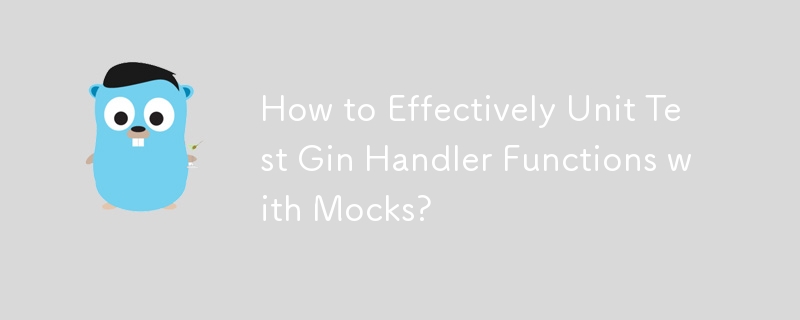
Mocks to Unit Test Gin Handler Functions
Testing c.BindQuery Functionality
To test operations involving the HTTP request in Gin, initialize an *http.Request and set it to the Gin context. Specifically for testing c.BindQuery, initialize the request's URL and URL.RawQuery:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | import (
"net/http/httptest"
"github.com/gin-gonic/gin"
)
func mockGin() (*gin.Context, *httptest.ResponseRecorder) {
w := httptest.NewRecorder()
c, _ := gin.CreateTestContext(w)
req := &http.Request{
URL: &url.URL{},
Header: make(http.Header),
}
testQuery := weldprogs.QueryParam{ }
q := req.URL.Query()
for _, s := range testQuery.Basematgroup_id {
q.Add( "basematgroup_id" , s)
}
req.URL.RawQuery = q.Encode()
c.Request = req
return c, w
}
|
Copy after login
Mocking JSON Binding
Refer to this resource for guidance on mocking JSON binding.
Testing Services
Services like services.WeldprogService.GetMaterialByFilter(&queryParam) cannot be tested as is. To make them testable:
- Convert them into interfaces.
- Inject them as dependencies into the handler.
- Set them as Gin context values.
Interface and Context Value Approach:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | type services interface {
GetMaterialByFilter(*weldprogs.QueryParam) (*weldprogs.MaterialByFilter, error)
}
func mockWeldprogService(service services) {
return func(c *gin.Context) {
c.Set( "svc_context_key" , service)
}
}
func TestGetMaterialByFilter(t *testing.T) {
w := httptest.NewRecorder()
c, _ := gin.CreateTestContext(w)
c.Use(mockWeldprogService(&mockSvc{}))
GetMaterialByFilter(c)
}
|
Copy after login
The above is the detailed content of How to Effectively Unit Test Gin Handler Functions with Mocks?. For more information, please follow other related articles on the PHP Chinese website!