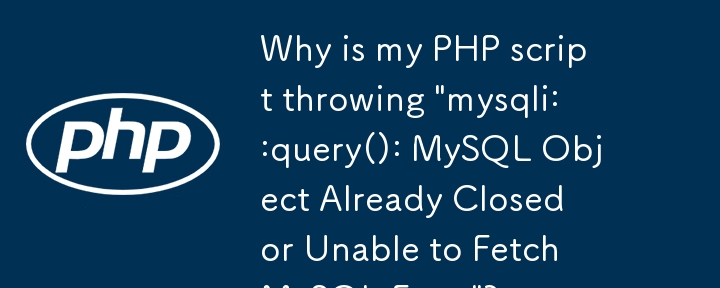
mysqli::query(): MySQL Object Already Closed or Unable to Fetch MySQL Error
Problem
While executing a PHP script, you encounter the following errors:
-
PHP 7: mysqli::query(): Couldn't fetch MySQL in [script_path] on line [line_number]
-
PHP 8: Uncaught Error: MySQL object is already closed
Explanation
These errors indicate that your PHP script is attempting to perform a MySQL query after the MySQL connection object has been closed.
Solution
Ensure that your MySQL connection object is still active before executing any queries.
// Check if the MySQL connection is open
if ($mysqli->connect_error) {
// Handle the connection error
} else {
// Execute the query
$result = $mysqli->query($query);
}
Copy after login
Possible Causes
-
Closing the connection too early: Ensure that you close the MySQL connection only after all queries have been executed.
-
Destructor issues: Avoid closing the MySQL connection in the destructor (__destruct) method of a class if there are still queries that need to be performed.
-
Incorrectly declared connection variables: Check that the MySQL connection variable ($mysqli in this case) is declared and assigned correctly throughout your script.
Additional Notes
- In the provided code snippet for the class EventCalendar, the MySQL connection is closed in the destructor (__destruct) method. This could be an issue if queries are still needed after the destructor is called.
- Ensure that you are using the correct PHP version and MySQL extension.
- Check the MySQL server logs for any additional error messages.
The above is the detailed content of Why is my PHP script throwing 'mysqli::query(): MySQL Object Already Closed or Unable to Fetch MySQL Error'?. For more information, please follow other related articles on the PHP Chinese website!