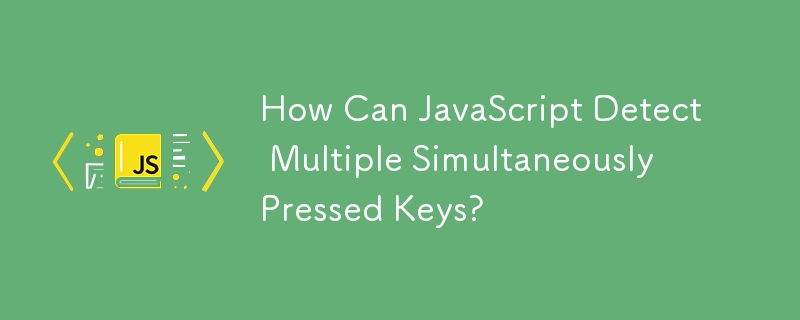
How to Detect If Multiple Keys Are Pressed At Once Using JavaScript
Problem Statement
When multiple keys are pressed simultaneously, a game engine may encounter issues with key detection. For instance, pressing the spacebar to jump and the right arrow key to move right might cause the character to stop moving after jumping. This is because the keydown function used to detect key presses cannot handle multiple simultaneous keystrokes.
Solution
- Create a JavaScript map object to keep track of pressed keys.
var map = {};
Copy after login
- Attach event listeners to both the keydown and keyup events.
onkeydown = onkeyup = function(e) {
e = e || event;
map[e.keyCode] = e.type == 'keydown';
};
Copy after login
- Check for multiple keys being pressed at once within your game engine logic.
if (map[17] && map[16] && map[65]) {
// CTRL+SHIFT+A pressed
}
Copy after login
Additional Notes
- keyCode is deprecated in favor of key, which returns a string representation of the key being pressed (e.g., "a" for A).
- Use addEventListener instead of element.onevent, as the latter overrides previous handlers.
- Prevent default browser actions by using event.preventDefault().
- Consider creating a helper class or function to abstract the multiple key detection logic.
The above is the detailed content of How Can JavaScript Detect Multiple Simultaneously Pressed Keys?. For more information, please follow other related articles on the PHP Chinese website!