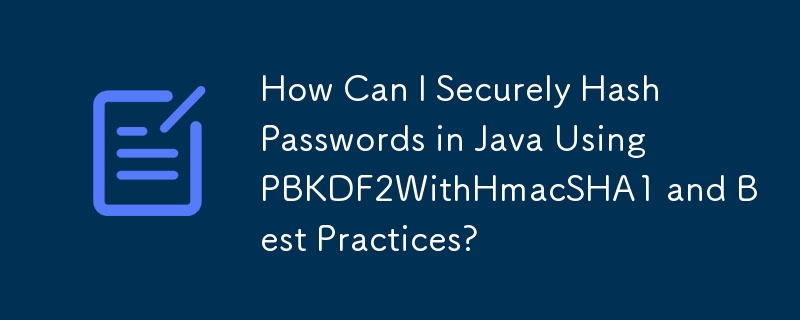
Password Hashing in Java: A Comprehensive Guide
Hashing passwords securely is crucial for protecting sensitive user data. In this comprehensive guide, we will delve into the nuances of password hashing in Java, addressing the following aspects:
Implementation using PBKDF2WithHmacSHA1
Java provides a built-in facility to securely hash passwords using PBKDF2WithHmacSHA1 (Password-Based Key Derivation Function 2), a widely recommended algorithm for password hashing. The implementation entails:
- Generating a random salt using SecureRandom.
- Creating a KeySpec object to configure the PBKDF2 algorithm.
- Deriving the hashed password using SecretKeyFactory.generateSecret(spec).
Password Authentication Utility
A utility class, PasswordAuthentication, is introduced to simplify password storage and authentication. The utility supports the following key features:
-
Hashing: Method to generate a secure authentication token for password storage.
-
Authentication: Method to check if a provided password matches a stored authentication token.
-
Cost Configuration: Parameterized constructor allows customizing the hashing cost.
PBKDF2 Algorithm Details
PBKDF2 is a highly secure hashing algorithm that iteratively derives a key from a password and a salt. The following key features contribute to its security:
- Salt: A random value added to the password to enhance uniqueness.
- Iterations: Controlling the number of iterations ensures high computational cost for password cracking attempts.
Pattern Matching for Token Validation
The PasswordAuthentication utility validates tokens using a specific pattern. Tokens generated using this utility conform to the format:
$(cost)$(encodedHash)
Copy after login
where:
- `$31$: Identifier prefix.
- cost: Exponential cost of hashing.
- encodedHash: Base64-encoded salt and hashed password.
Best Practices and Considerations
-
Store Passwords in char[]: Arrays should be cleared after use to avoid memory leaks.
-
Use Strong Passwords: Encourage users to create complex and unpredictable passwords.
-
Limit Failed Login Attempts: Implement rate limiting to prevent brute force attacks.
-
Secure Salt Generation: Utilize a SecureRandom for generating the salt to ensure its unpredictability.
The above is the detailed content of How Can I Securely Hash Passwords in Java Using PBKDF2WithHmacSHA1 and Best Practices?. For more information, please follow other related articles on the PHP Chinese website!