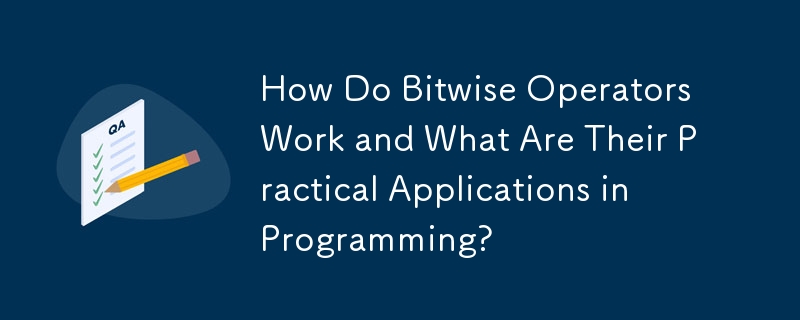
Understanding Bitwise Operations
In programming, bitwise operations manipulate multi-bit values one bit at a time. Unlike arithmetic operators, which perform mathematical calculations, bitwise operators focus on individual bits within a value.
Types of Bitwise Operators
-
Bitwise AND (&): Outputs 1 only if both input bits are 1, otherwise it's 0.
-
Bitwise OR (|): Outputs 1 if one or both input bits are 1, otherwise it's 0.
-
Bitwise XOR (^): Outputs 1 only if exactly one input bit is 1, otherwise it's 0.
-
Bitwise NOT (~): Outputs 1 only if its input bit is 0, otherwise it's 0.
Shift Operators
In Python, >> and << are commonly used in bitwise operations.
- Left Shift (<<): Shifts the bits to the left by the specified number of positions.
- Right Shift (>>): Shifts the bits to the right by the specified number of positions.
Practical Uses of Bitwise Operators
-
Filtering Data: By using & with a mask, it's possible to selectively filter out specific bits from a value.
-
Bit Packing: Combining multiple small values into a larger one by using << and |.
-
Manipulating Flags: Checking and setting individual flags within a status register.
-
Arithmetic Operations: Bitwise operators can be used for certain arithmetic operations, such as exponentiation (using repeated shifts).
Example
Consider the Python code:
x = 1 # 0001
x << 2 # Shift left 2 bits: 0100
# Result: 4
x | 2 # Bitwise OR: 0011
# Result: 3
x & 1 # Bitwise AND: 0001
# Result: 1
Copy after login
- Left Shift (<< 2): Shifts the bits of x to the left by 2 positions, resulting in a value of 4.
- Bitwise OR (| 2): Combines the bits of x with 2, resulting in a value of 3.
- Bitwise AND (& 1): Checks if the least significant bit of x is 1, which it is, resulting in a value of 1.
The above is the detailed content of How Do Bitwise Operators Work and What Are Their Practical Applications in Programming?. For more information, please follow other related articles on the PHP Chinese website!