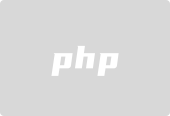
In this guide, we’ll create a simple moderation bot for Discord using Node.js and the Discord.js library. This bot will include features like banning, muting, and monitoring chat activity.
Creating a Moderation Bot for Discord
In this guide, we’ll create a simple moderation bot for Discord using Node.js and the Discord.js library. This bot will include features like banning, muting, and monitoring chat activity.
Prerequisites
-
Node.js Installed: Download and install Node.js from nodejs.org.
-
Discord Account: Ensure you have a Discord account and administrative access to the server where you’ll test the bot.
-
Basic JavaScript Knowledge: Familiarity with JavaScript basics is recommended.
Step 1: Create a New Discord Bot
- Go to the Discord Developer Portal.
- Click New Application and give your bot a name.
- In the left sidebar, go to Bot and click Add Bot.
- Copy the bot’s Token for later use (keep it private).
- Under "Privileged Gateway Intents," enable MESSAGE CONTENT INTENT to allow the bot to read messages.
Step 2: Set Up Your Project
- Open a terminal and create a new folder for your bot:
mkdir discord-moderation-bot
cd discord-moderation-bot
Copy after login
Copy after login
- Initialize a new Node.js project:
npm init -y
Copy after login
Copy after login
- Install Discord.js:
npm install discord.js
Copy after login
Copy after login
- Create an index.js file in the folder to hold your bot’s code:
touch index.js
Copy after login
Copy after login
Step 3: Write the Bot Code
Open index.js in a code editor and add the following code:
1. Import and Configure Discord.js
mkdir discord-moderation-bot
cd discord-moderation-bot
Copy after login
Copy after login
2. Set Up Bot Login and Ready Event
npm init -y
Copy after login
Copy after login
3. Add Moderation Commands
Ban Command
npm install discord.js
Copy after login
Copy after login
Mute Command
touch index.js
Copy after login
Copy after login
Clear Messages Command
const { Client, GatewayIntentBits } = require('discord.js');
const client = new Client({
intents: [
GatewayIntentBits.Guilds,
GatewayIntentBits.GuildMessages,
GatewayIntentBits.MessageContent,
GatewayIntentBits.GuildMembers
]
});
const TOKEN = 'YOUR_BOT_TOKEN'; // Replace with your bot token
Copy after login
Step 4: Invite the Bot to Your Server
- Go back to the Discord Developer Portal.
- In the left sidebar, click OAuth2 > URL Generator.
- Under Scopes, select bot. Under Bot Permissions, select:
- Ban Members
- Manage Roles
- Manage Messages
- Copy the generated URL and paste it into your browser to invite the bot to your server.
Step 5: Test Your Bot
- Run the bot:
client.once('ready', () => {
console.log(`Logged in as ${client.user.tag}!`);
});
client.login(TOKEN);
Copy after login
- In your Discord server, try using the following commands:
-
!ban @user to ban a user.
-
!mute @user to mute a user (ensure a "Muted" role exists).
-
!clear to delete a specified number of messages.
Additional Tips
-
Improve Error Handling: Add better logging and user feedback for errors.
-
Add a Help Command: Provide users with a list of commands and their descriptions.
-
Secure Your Bot Token: Use environment variables or a configuration file to keep your token safe.
-
Expand Features: Add warnings, unmute, or even automatic spam detection using a message tracker.
With this guide, you have a fully functional moderation bot that you can customize to suit your server’s needs!
The above is the detailed content of Creating a Moderation Bot for Discord. For more information, please follow other related articles on the PHP Chinese website!