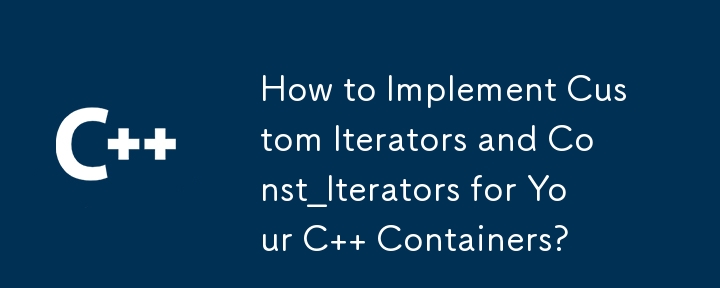
Implementing Custom Iterators and Const_Iterators for Custom Containers
Implementing iterators and const_iterators for custom containers can be a daunting task. This guide provides comprehensive guidelines and addresses common pitfalls to help you create robust and efficient iterators.
Guidelines for Iterator Creation
-
Determine the Iterator Type: First, determine the type of iterator appropriate for your container, considering its capabilities (input, output, forward, etc.).
-
Base Classes: Leverage base iterator classes from the standard library, such as std::iterator with the desired tag (e.g., random_access_iterator_tag) to handle common functionality and type definitions.
-
Template Iterators: Define your iterator class as a template to parameterize it for different value types, pointer types, or reference types as needed. For instance:
template <typename PointerType>
class MyIterator {
// Iterator definition goes here
};
typedef MyIterator<int*> iterator_type;
typedef MyIterator<const int*> const_iterator_type;
Copy after login
This approach allows you to define distinct types for non-const and const iterators.
Avoiding Code Duplication
To avoid code duplication between const_iterator and iterator:
-
Template Parameters: Ensure that the template parameters of the iterator class allow for different types for const and non-const iterators.
-
Specific Version: Define a specific version of your iterator class for const_iterators where necessary. This ensures that const_iterators have the appropriate behavior and cannot modify the underlying data.
Additional Considerations
- Ensure that the iterator class provides the required methods and operators (e.g., dereference, increment/decrement, equality comparison).
- Handle special cases like accessing elements at the beginning or end of the container.
- Consider the performance implications of iterator operations and optimize where possible.
References:
- Standard Library Reference for Iterators
- [Discussion on std::iterator Depreciation](https://www.reddit.com/r/cpp/comments/8d3opw/stditerator_deprecated_by_cpp17/)
The above is the detailed content of How to Implement Custom Iterators and Const_Iterators for Your C Containers?. For more information, please follow other related articles on the PHP Chinese website!