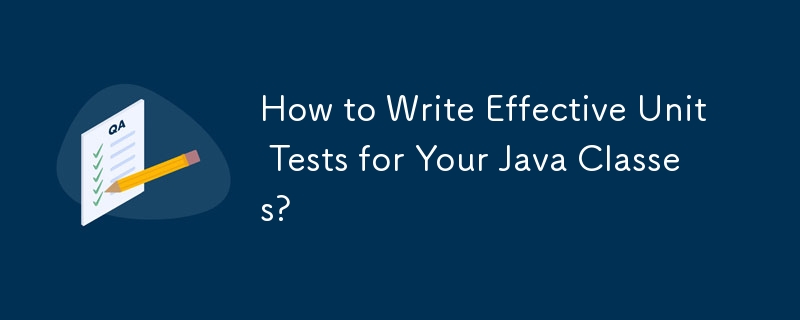
How to Write Unit Tests for Your Java Class
To effectively evaluate the functionality of a Java class, writing unit tests is crucial. Here's how you can approach it:
For Eclipse IDE:
- Create a new Java project and a new class with your binary sum logic.
- Go to File > New > JUnit Test Case.
- Initialize your test case in the setUp() method and write a test method, for instance, testAdd().
- Assert the expected result against the actual result using Assert.assertEquals().
- Run your test by right-clicking on the test class and selecting Run as > JUnit Test.
For IntelliJ IDEA:
- Create a "test" directory in the root project folder and mark it as a test sources root.
- Create a Java class (Test.java) in the appropriate package within the test directory.
- Import necessary testing libraries, such as org.junit.jupiter.api.Assertions.
- Write a test method with the @Test annotation, following the format: @Test public void test().
- Use Assertions class to verify expected and actual values, e.g., Assertions.assertEquals().
- Run your tests by right-clicking on the test and selecting Run.
The above is the detailed content of How to Write Effective Unit Tests for Your Java Classes?. For more information, please follow other related articles on the PHP Chinese website!